Sending Emails with AWS SES: A Comprehensive Guide
AWS Simple Email Service (SES) is a powerful, cost-effective solution that can help you send emails securely, whether it's for transactional messages, marketing campaigns, or automated notifications.
In this blog post, we'll explore how to use AWS SES to send emails, covering various use cases like sending HTML templates, attachments, and even calendar events. We'll walk through practical examples to help you get started quickly.
Table of Contents
- What is AWS SES?
- Setting Up AWS SES
- Sending a Simple Email
- Sending HTML Emails
- Sending Emails with Attachments
- Sending Calendar Invites
- Best Practices
- Conclusion
What is AWS SES?
AWS Simple Email Service (SES) is a cloud-based email sending service designed to help digital marketers and application developers send marketing, notification, and transactional emails. It is a reliable, scalable, and cost-effective service for businesses of all sizes.
Key Features:
- Scalability: Handles large volumes of emails effortlessly.
- Deliverability: High deliverability rates due to AWS's reputation.
- Cost-Effective: Pay-as-you-go pricing model.
- Security: Supports authentication mechanisms like DKIM and SPF.
Setting Up AWS SES
Before we dive into sending emails, let's set up AWS SES for your account.
Step 1: Verify Your Email Address or Domain
AWS SES requires you to verify the email addresses or domains you plan to use.
- Verify an Email Address:
- Go to the AWS SES console.
- Navigate to Email Addresses under Identity Management.
- Click Verify a New Email Address.
- Enter your email address and click Verify This Email Address.
- Check your inbox and click the verification link in the email from AWS.
- Verify a Domain:
- Navigate to Domains under Identity Management.
- Click Verify a New Domain.
- Enter your domain name.
- AWS will provide DNS records. Add these to your domain's DNS settings.
Step 2: Request Production Access
By default, new AWS accounts are in the Sandbox environment, which limits email sending capabilities.
- Go to the SES Sending Limits page.
- Click Request a Sending Limit Increase.
- Fill out the request form to move out of the sandbox.
Step 3: Set Up AWS Credentials
You'll need AWS access keys to interact with SES programmatically.
- Go to the AWS IAM console.
- Create a new user with Programmatic access.
- Attach the AmazonSESFullAccess policy.
- Save your Access Key ID and Secret Access Key.
Sending a Simple Email
Let's start by sending a simple plain-text email using AWS SDK for Node.js.
Prerequisites
- Node.js installed on your machine.
- AWS SDK for Node.js (aws-sdk) installed.
Code Example
const AWS = require('aws-sdk'); // Configure AWS SDK AWS.config.update({ accessKeyId: 'YOUR_ACCESS_KEY_ID', secretAccessKey: 'YOUR_SECRET_ACCESS_KEY', region: 'us-east-1', // Replace with your SES region }); const ses = new AWS.SES(); const params = { Source: 'sender@example.com', Destination: { ToAddresses: ['recipient@example.com'], }, Message: { Subject: { Data: 'Test Email from AWS SES', }, Body: { Text: { Data: 'Hello, this is a test email sent using AWS SES!', }, }, }, }; ses.sendEmail(params, (err, data) => { if (err) { console.error('Error sending email', err); } else { console.log('Email sent successfully', data); } });
Explanation:
- Source: The verified email address you're sending from.
- Destination: The recipient's email address.
- Message: Contains the subject and body of the email.
Sending HTML Emails
Now, let's send an email with HTML content to make it more visually appealing.
Code Example
const params = { Source: 'sender@example.com', Destination: { ToAddresses: ['recipient@example.com'], }, Message: { Subject: { Data: 'Welcome to Our Service!', }, Body: { Html: { Data: ` <html> <body> <h1>Welcome!</h1> <p>We're glad to have you on board.</p> </body> </html> `, }, }, }, }; ses.sendEmail(params, (err, data) => { if (err) { console.error('Error sending HTML email', err); } else { console.log('HTML email sent successfully', data); } });
Tips:
- You can include CSS styles inline or use basic styling to ensure compatibility across email clients.
- Always include a plain-text version as a fallback.
Sending Emails with Attachments
To send emails with attachments, we'll use the sendRawEmail method instead of sendEmail.
Code Example
const fs = require('fs'); const path = require('path'); const AWS = require('aws-sdk'); const ses = new AWS.SES(); // Read the attachment file const filePath = path.join(__dirname, 'attachment.pdf'); const fileContent = fs.readFileSync(filePath); // Define the email parameters const params = { RawMessage: { Data: createRawEmail(), }, }; function createRawEmail() { const boundary = '----=_Part_0_123456789.123456789'; let rawEmail = [ `From: sender@example.com`, `To: recipient@example.com`, `Subject: Email with Attachment`, `MIME-Version: 1.0`, `Content-Type: multipart/mixed; boundary="${boundary}"`, ``, `--${boundary}`, `Content-Type: text/plain; charset=UTF-8`, `Content-Transfer-Encoding: 7bit`, ``, `Hello, please find the attached document.`, `--${boundary}`, `Content-Type: application/pdf; name="attachment.pdf"`, `Content-Description: attachment.pdf`, `Content-Disposition: attachment; filename="attachment.pdf";`, `Content-Transfer-Encoding: base64`, ``, fileContent.toString('base64'), `--${boundary}--`, ].join('\n'); return rawEmail; } ses.sendRawEmail(params, (err, data) => { if (err) { console.error('Error sending email with attachment', err); } else { console.log('Email with attachment sent successfully', data); } });
Explanation:
- Multipart MIME Message: We construct a raw email with MIME boundaries to include attachments.
- Base64 Encoding: Attachments must be base64 encoded.
- Content Headers: Proper headers are necessary for the email client to interpret the attachment correctly.
Sending Calendar Invites
To send a calendar event, we'll include an .ics file as an attachment.
Code Example
function createCalendarEvent() { const event = [ 'BEGIN:VCALENDAR', 'VERSION:2.0', 'BEGIN:VEVENT', 'DTSTAMP:20231016T090000Z', 'DTSTART:20231020T100000Z', 'DTEND:20231020T110000Z', 'SUMMARY:Meeting Invitation', 'DESCRIPTION:Discuss project updates', 'LOCATION:Conference Room', 'END:VEVENT', 'END:VCALENDAR', ].join('\n'); return Buffer.from(event).toString('base64'); } function createRawEmail() { const boundary = '----=_Part_0_123456789.123456789'; let rawEmail = [ `From: sender@example.com`, `To: recipient@example.com`, `Subject: Meeting Invitation`, `MIME-Version: 1.0`, `Content-Type: multipart/mixed; boundary="${boundary}"`, ``, `--${boundary}`, `Content-Type: text/plain; charset=UTF-8`, `Content-Transfer-Encoding: 7bit`, ``, `Hello, you're invited to a meeting.`, `--${boundary}`, `Content-Type: text/calendar; method=REQUEST; name="invite.ics"`, `Content-Transfer-Encoding: base64`, `Content-Disposition: attachment; filename="invite.ics"`, ``, createCalendarEvent(), `--${boundary}--`, ].join('\n'); return rawEmail; } ses.sendRawEmail(params, (err, data) => { if (err) { console.error('Error sending calendar invite', err); } else { console.log('Calendar invite sent successfully', data); } });
Explanation:
- Calendar Event Format: We create an .ics file content using the iCalendar format.
- Method=REQUEST: Indicates that this is a meeting invitation.
- Proper Headers: Ensuring correct Content-Type and Content-Disposition for calendar files.
Best Practices
- Error Handling: Always include robust error handling in your applications.
- Email Validation: Ensure that email addresses are valid before sending.
- Throttling: Be aware of your SES sending limits to avoid throttling.
- Unsubscribe Links: Include unsubscribe options in marketing emails to comply with regulations.
- Monitoring: Use AWS CloudWatch to monitor your email sending activities.
- Security: Protect your AWS credentials and use IAM roles where possible.
Conclusion
AWS SES is a versatile service that can handle a variety of email sending needs. Whether you're sending simple notifications, marketing emails with rich HTML content, or complex messages with attachments and calendar events, AWS SES has you covered.
By following this guide, you should now have a solid understanding of how to:
- Set up AWS SES for your account.
- Send plain-text and HTML emails.
- Include attachments and calendar invites in your emails.
Thank you for reading! If you have any questions or tips to share, feel free to leave a comment below. Happy coding!
The above is the detailed content of Sending Emails with AWS SES: A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










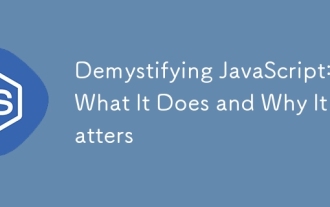
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
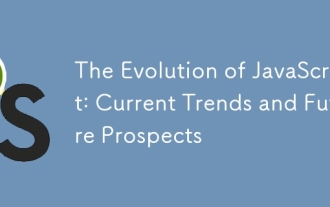
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
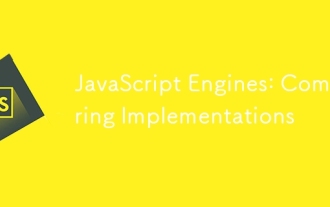
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
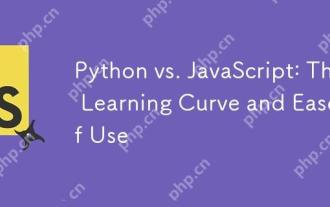
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
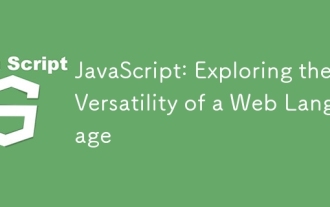
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
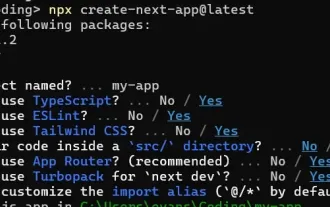
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
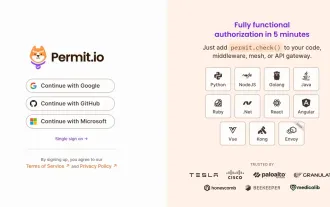
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
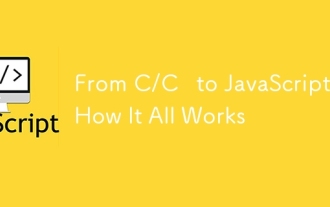
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
