


How to Implement QThreads in PyQt for Responsive GUI Applications?
Proper Implementation of QThreads in PyQt
In PyQt Gui applications, separating lengthy operations into separate threads is often desirable to maintain GUI responsiveness. However, re-implementing the run method is discouraged as per the reference mentioned in the question.
To demonstrate the correct approach in Python, consider the following example that utilizes signals and slots for communication between the GUI and worker thread:
- Create a Worker Thread: Initialize a worker object and move it to a separate thread to be started.
- Connect Signals and Slots: Establish signal and slot connections between the worker and GUI objects for status updates.
- Start Worker Thread: When the "Start" button is clicked, the GUI signals the worker thread to initiate calculations.
- Cancel Worker Thread: If needed, the "Cancel" button forcibly terminates the worker thread and creates a new one.
Here is the code implementation:
<code class="python">from PyQt4 import QtGui, QtCore import sys import random class Example(QtCore.QObject): signalStatus = QtCore.pyqtSignal(str) def __init__(self, parent=None): super(self.__class__, self).__init__(parent) self.gui = Window() self.createWorkerThread() self._connectSignals() self.gui.show() def _connectSignals(self): self.gui.button_cancel.clicked.connect(self.forceWorkerReset) self.signalStatus.connect(self.gui.updateStatus) self.parent().aboutToQuit.connect(self.forceWorkerQuit) def createWorkerThread(self): self.worker = WorkerObject() self.worker_thread = QtCore.QThread() self.worker.moveToThread(self.worker_thread) self.worker_thread.start() self.worker.signalStatus.connect(self.gui.updateStatus) self.gui.button_start.clicked.connect(self.worker.startWork) def forceWorkerReset(self): if self.worker_thread.isRunning(): print('Terminating thread.') self.worker_thread.terminate() print('Waiting for thread termination.') self.worker_thread.wait() self.signalStatus.emit('Idle.') print('building new working object.') self.createWorkerThread() def forceWorkerQuit(self): if self.worker_thread.isRunning(): self.worker_thread.terminate() self.worker_thread.wait() class WorkerObject(QtCore.QObject): signalStatus = QtCore.pyqtSignal(str) def __init__(self, parent=None): super(self.__class__, self).__init__(parent) @QtCore.pyqtSlot() def startWork(self): for ii in range(7): number = random.randint(0, 5000**ii) self.signalStatus.emit('Iteration: {}, Factoring: {}'.format(ii, number)) factors = self.primeFactors(number) print('Number: ', number, 'Factors: ', factors) self.signalStatus.emit('Idle.') def primeFactors(self, n): i = 2 factors = [] while i * i <= n: if n % i: i += 1 else: n //= i factors.append(i) if n > 1: factors.append(n) return factors class Window(QtGui.QWidget): def __init__(self): QtGui.QWidget.__init__(self) self.button_start = QtGui.QPushButton('Start', self) self.button_cancel = QtGui.QPushButton('Cancel', self) self.label_status = QtGui.QLabel('', self) layout = QtGui.QVBoxLayout(self) layout.addWidget(self.button_start) layout.addWidget(self.button_cancel) layout.addWidget(self.label_status) self.setFixedSize(400, 200) @QtCore.pyqtSlot(str) def updateStatus(self, status): self.label_status.setText(status) if __name__ == '__main__': app = QtGui.QApplication(sys.argv) example = Example(app) sys.exit(app.exec_())</code>
By adhering to this approach, you can effectively utilize QThreads in PyQt applications, ensuring that the GUI remains responsive even during lengthy operations.
The above is the detailed content of How to Implement QThreads in PyQt for Responsive GUI Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










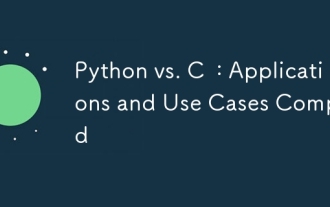
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
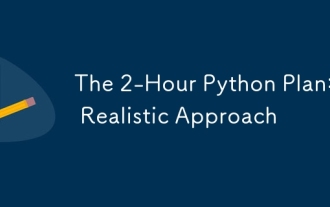
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
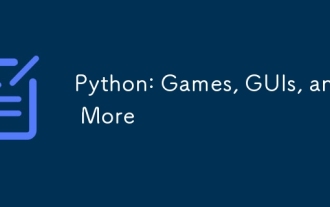
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
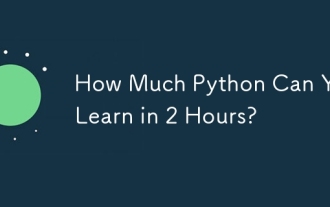
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
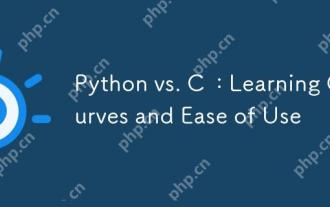
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
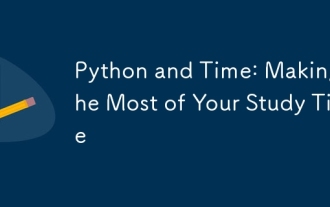
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
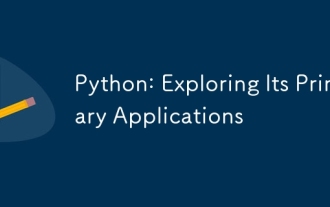
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
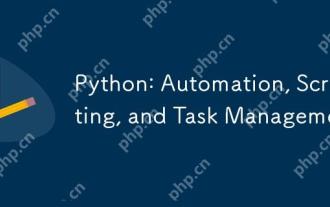
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
