


Why Iterating and Removing from a List in Python Can Cause Unexpected Results
Removing Items from a List During Iteration - Why the Typical Idiom Fails
In programming, it's common practice to iterate over a list and remove items as needed. However, when it comes to Python, there's a caveat to this idiom that can cause unexpected results.
Consider the following Python code:
<code class="python">letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l'] for i in letters: letters.remove(i) print(letters)</code>
This code attempts to remove all items from a list. Surprisingly, the output of the code is not an empty list, but instead contains every other item from the original list:
['b', 'd', 'f', 'h', 'j', 'l']
Explanation
The reason for this behavior lies in Python's handling of list modification during iteration. The documentation clearly states:
"It is not safe to modify the sequence being iterated over in the loop [...] If you need to modify the list you are iterating over [...] you must iterate over a copy."
In the provided code, we are attempting to modify the list letters while iterating over it. Python's design for handling this situation is that the iteration proceeds with skipping every other item after each removal.
Rewriting the Code
To avoid this issue, the code must be rewritten to create a copy of the list before iterating over it. There are several methods to accomplish this:
- del letters[:]: Removes all items from the list, effectively replacing it with an empty list.
- letters[:] = []: Similar to del letters[:], but generates a new empty list instead of modifying the original.
- letters = []: Assigns a completely new empty list to the variable letters.
Alternatively, if the goal is to remove specific items based on a condition, it's more efficient to use the filter() function to create a new list containing only the desired items. This approach avoids the need for iteration over a copy of the original list.
The above is the detailed content of Why Iterating and Removing from a List in Python Can Cause Unexpected Results. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










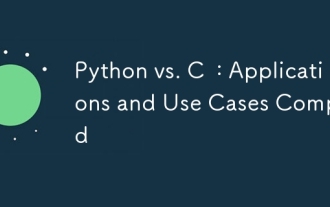
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
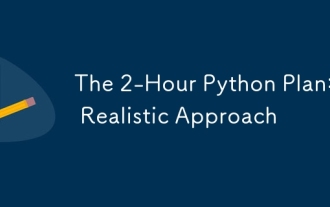
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
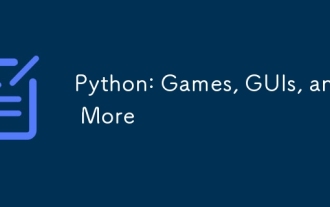
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
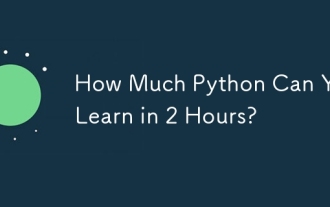
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
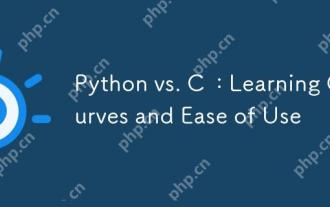
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
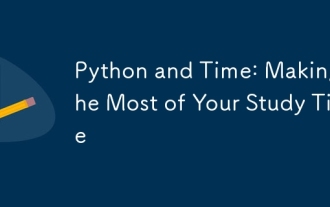
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
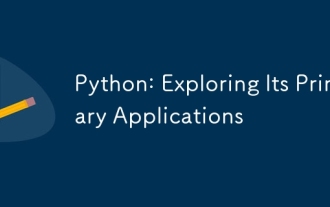
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
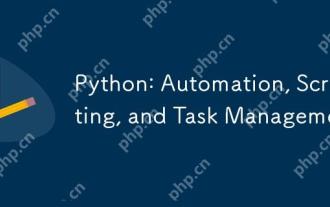
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
