Building a Room Cost Estimator with JavaScript
What Will This Estimator Do?
- Allow users to select a room type (Queen, King, or Suite).
- Let users choose the number of nights they want to stay.
- Apply any discounts (e.g., AAA/Senior or Military).
- Calculate the room cost, apply tax, and display the total.
STEP_1: Handling Form Submission
First, we need to capture what the user submits in the form. We'll use JavaScript to prevent the page from refreshing when the form is submitted, allowing us to handle the calculation without losing the input.
document.getElementById("costForm").addEventListener("submit", function (event) { event.preventDefault(); // Prevents the page from reloading // 1. Get the values from the form const name = document.getElementById("name").value; const checkInDate = document.getElementById("checkInDate").value; const nights = parseInt(document.getElementById("nights").value); const roomType = document.querySelector('input[name="roomType"]:checked').value; const discount = document.querySelector('input[name="discount"]:checked').value; const adults = parseInt(document.getElementById("adults").value); const children = parseInt(document.getElementById("children").value); // 2. Check room occupancy const maxOccupancy = getMaxOccupancy(roomType); if (adults + children > maxOccupancy) { document.getElementById("messageDiv").innerText = `The room you selected cannot hold your group. Max occupancy is ${maxOccupancy}.`; return; } // 3. Clear previous messages and calculate the cost document.getElementById("messageDiv").innerText = ""; calculateCost(roomType, checkInDate, nights, discount); });
WHAT IT DOES:
- Form submission: The form listens for the "submit" event, and event.preventDefault() prevents the page from refreshing so we can handle the input with JavaScript.
- Getting form values: We retrieve the values from the form, like the user’s name, the number of nights, the room type, etc.
- Room occupancy check: We use a function to check if the number of guests exceeds the room’s maximum occupancy. If it does, we show an error message.
STEP_2: Checking Maximum Occupancy
Now, let's create the function that checks how many people can stay in each room type. This function is important because the hotel rooms have limits on how many guests they can accommodate.
function getMaxOccupancy(roomType) { if (roomType === "queen") { return 5; // Queen rooms can hold up to 5 people } else if (roomType === "king") { return 2; // King rooms can hold up to 2 people } else if (roomType === "suite") { return 6; // 2-Bedroom Suites can hold up to 6 people } }
WHAT IT DOES:
-
Room types: Each room type (Queen, King, Suite) has a different maximum occupancy.
- Queen room: Max occupancy is 5 people.
- King room: Max occupancy is 2 people.
- Suite: Max occupancy is 6 people.
- If the number of adults children exceeds the limit, we show an error and stop the calculation.
STEP_3: Calculating the Room Rate Based on Date
Next, we calculate the nightly room rate based on the check-in date and room type. The rates are higher during the high season (June to August) and lower during the rest of the year.
document.getElementById("costForm").addEventListener("submit", function (event) { event.preventDefault(); // Prevents the page from reloading // 1. Get the values from the form const name = document.getElementById("name").value; const checkInDate = document.getElementById("checkInDate").value; const nights = parseInt(document.getElementById("nights").value); const roomType = document.querySelector('input[name="roomType"]:checked').value; const discount = document.querySelector('input[name="discount"]:checked').value; const adults = parseInt(document.getElementById("adults").value); const children = parseInt(document.getElementById("children").value); // 2. Check room occupancy const maxOccupancy = getMaxOccupancy(roomType); if (adults + children > maxOccupancy) { document.getElementById("messageDiv").innerText = `The room you selected cannot hold your group. Max occupancy is ${maxOccupancy}.`; return; } // 3. Clear previous messages and calculate the cost document.getElementById("messageDiv").innerText = ""; calculateCost(roomType, checkInDate, nights, discount); });
WHAT IT DOES:
- Date calculation: We use the JavaScript Date object to extract the month from the check-in date.
-
High season (June - August): Rates are higher during these months.
- Queen and King rooms: $250 per night.
- Suites: $350 per night.
-
Off-season (rest of the year): Rates are lower.
- Queen and King rooms: $150 per night.
- Suites: $210 per night.
STEP_4: Calculating the Total Cost
Finally, we calculate the total cost of the stay. We apply any discounts and then add tax to get the final total.
function getMaxOccupancy(roomType) { if (roomType === "queen") { return 5; // Queen rooms can hold up to 5 people } else if (roomType === "king") { return 2; // King rooms can hold up to 2 people } else if (roomType === "suite") { return 6; // 2-Bedroom Suites can hold up to 6 people } }
WHAT IT DOES:
- Room rate: We calculate the room rate per night using the getRoomRate() function.
- Total room cost: We multiply the room rate by the number of nights to get the total cost before discounts.
- Applying discounts: If the user selects AAA/Senior or Military, we apply a discount (10% or 20%, respectively).
- Tax: We add 12% tax to the discounted room cost.
- Displaying results: The total cost (with a breakdown) is displayed on the page for the user to see.
Conclusion
- 1. Get Form Values: When the user submits the form, the code first grabs all the input values from the form (name, check-in date, number of nights, etc.).
- 2. Validate Occupancy: The script checks if the number of guests exceeds the room’s maximum occupancy and shows an error if it does.
- 3. Calculate Room Cost: Based on the selected room type and the check-in date, the script calculates the nightly rate, multiplies it by the number of nights, and applies any discounts.
- 4. Add Tax: The script adds a 12% tax to the discounted room cost.
- 5. Display the Result: The final cost (with a breakdown) is shown to the user in the result section.
And that’s it! We’ve built a simple yet functional Room Cost Estimator using JavaScript.
My GitHub Repo for this code is here! Including the basic HTML and Bootstrap styling for the page
Author
Thounny Keo
Creative Developer & Designer
Frontend Development Student | Year Up United
The above is the detailed content of Building a Room Cost Estimator with JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










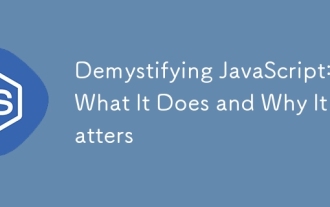
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
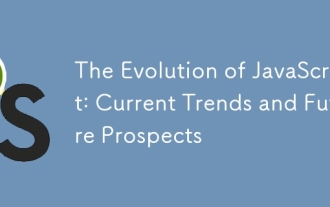
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
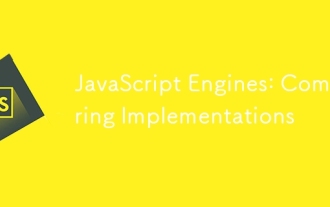
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
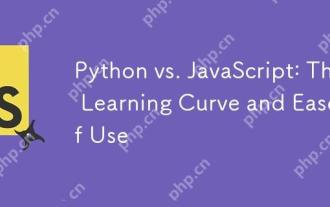
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
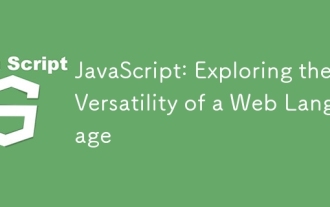
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
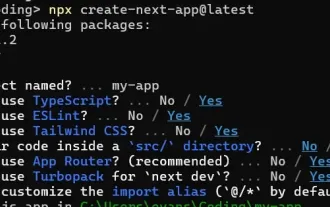
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
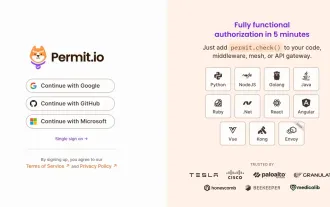
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
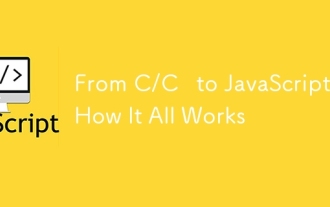
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
