


How to Efficiently Filter Pandas Data Objects Using Boolean Indexing?
Efficient Filtering of Pandas Dataframes and Series Using Boolean Indexing
In data analysis scenarios, applying multiple filters to narrow down results is often crucial. This article aims to address an efficient approach to chaining multiple comparison operations on Pandas data objects.
The Challenge
The goal is to process a dictionary of relational operators and apply them additively to a given Pandas Series or DataFrame, resulting in a filtered dataset. This operation requires minimizing unnecessary data copying, especially when dealing with large datasets.
Solution: Boolean Indexing
Pandas provides a highly efficient mechanism for filtering data using boolean indexing. Boolean indexing involves creating logical conditions and then indexing the data using these conditions. Consider the following example:
<code class="python">df.loc[df['col1'] >= 1, 'col1']</code>
This line of code selects all rows in the DataFrame df where the value in the 'col1' column is greater than or equal to 1. The result is a new Series object containing the filtered values.
To apply multiple filters, we can combine boolean conditions using logical operators like & (and) and | (or). For instance:
<code class="python">df[(df['col1'] >= 1) & (df['col1'] <= 1)]
This operation filters rows where 'col1' is both greater than or equal to 1 and less than or equal to 1.
Helper Functions
To simplify the process of applying multiple filters, we can create helper functions:
<code class="python">def b(x, col, op, n): return op(x[col], n) def f(x, *b): return x[(np.logical_and(*b))]
The b function creates a boolean condition for a given column and operator, while f applies multiple boolean conditions to a DataFrame or Series.
Usage Example
To use these functions, we can provide a dictionary of filter criteria:
<code class="python">filters = {'>=': [1], '<=': [1]}</code>
<code class="python">b1 = b(df, 'col1', ge, 1) b2 = b(df, 'col1', le, 1) filtered_df = f(df, b1, b2)</code>
This code applies the filters to the 'col1' column in the DataFrame df and returns a new DataFrame with the filtered results.
Enhanced Functionality
Pandas 0.13 introduced the query method, which offers a convenient way to apply filters using string expressions. For valid column identifiers, the following code becomes possible:
<code class="python">df.query('col1 <= 1 & 1 <= col1')</code>
This line achieves the same filtering as our previous example using a more concise syntax.
By utilizing boolean indexing and helper functions, we can efficiently apply multiple filters to Pandas dataframes and series. This approach minimizes data copying and enhances performance, particularly when working with large datasets.
The above is the detailed content of How to Efficiently Filter Pandas Data Objects Using Boolean Indexing?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










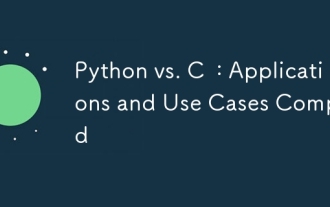
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
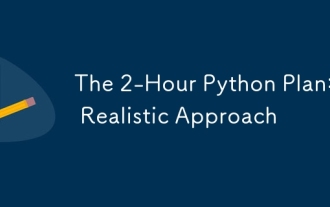
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
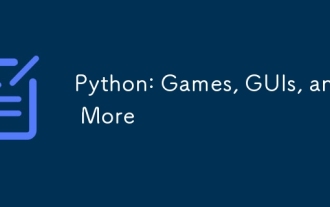
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
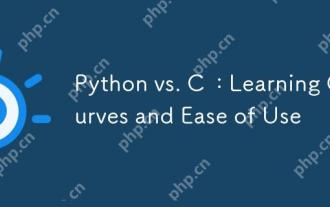
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
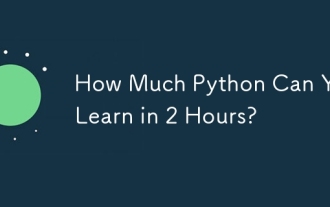
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
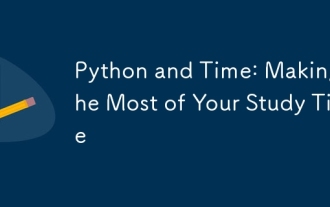
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
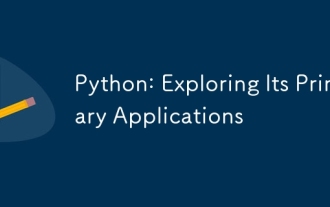
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
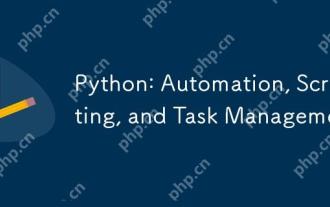
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
