


How to Enforce Character Limits on TextAreas in HTML without Event Handlers?
Enforcing TextArea Character Limits in HTML with JavaScript
Expanding upon the functionality provided by the HTML maxlength attribute, JavaScript offers a versatile solution for automatically imposing character limits on text areas. By eliminating the need for manual event handling, this approach provides a streamlined and efficient way to enforce input restrictions.
Imposing Maxlength without Event Handlers
The conventional approach to limiting textarea characters involves using event handlers like onkeypress or onkeyup. However, this becomes tedious when needing to specify limits for multiple text areas.
JavaScript allows for a more elegant solution that bypasses explicit event handling:
<code class="javascript">window.onload = function() { // Retrieve all text areas on the page var txts = document.getElementsByTagName('TEXTAREA'); // Loop through each text area for(var i = 0, l = txts.length; i < l; i++) { // If the textarea has a valid maxlength attribute (numeric value) if(/^[0-9]+$/.test(txts[i].getAttribute("maxlength"))) { // Define a function to handle maxlength enforcement var func = function() { var len = parseInt(this.getAttribute("maxlength"), 10); // Check if the input length exceeds the limit if(this.value.length > len) { // Alert the user and truncate the input alert('Maximum length exceeded: ' + len); this.value = this.value.substr(0, len); return false; } } // Assign the function to the onkeyup and onblur events txts[i].onkeyup = func; txts[i].onblur = func; } }; };</code>
Implementation Details
- window.onload: Execute the script once the page is loaded.
- getElementsByTagName: Retrieve all text area elements on the page.
- Regex test: Check if the maxlength attribute is a valid numeric value.
- func: Defines a function that checks if the input length exceeds the maxlength and truncates the input if necessary.
- Event handling: Assigns the func function to the onkeyup and onblur events for each text area with a valid maxlength attribute.
Benefits
- Automatic enforcement: Character limits are automatically imposed without requiring manual event handling for each text area.
- Ease of implementation: The provided script simplifies the process of enforcing maxlength across multiple text areas.
- Modular approach: The maxlength enforcement logic is self-contained within a function, making it easy to reuse or customize.
The above is the detailed content of How to Enforce Character Limits on TextAreas in HTML without Event Handlers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










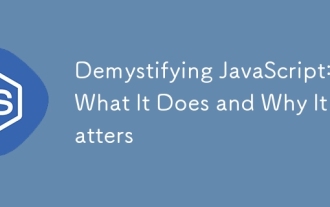
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
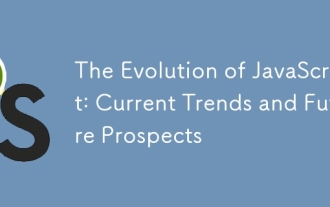
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
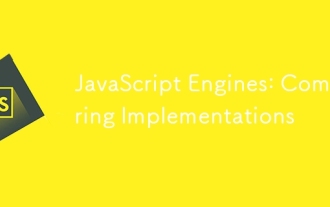
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
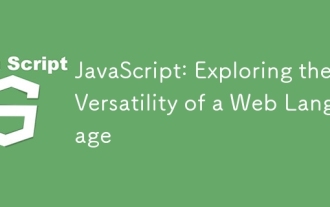
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
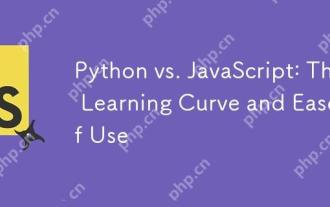
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
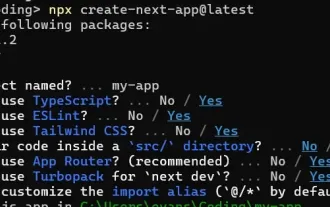
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
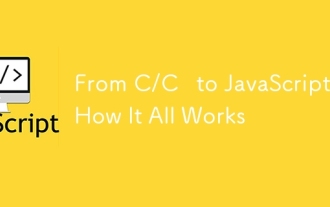
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
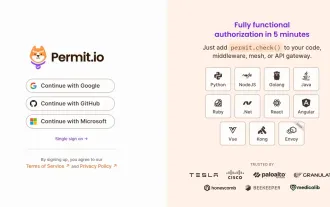
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
