


How to Effectively Loop Through Selected Elements Using document.querySelectorAll
Looping Through Selected Elements with document.querySelectorAll
document.querySelectorAll is a powerful method that allows you to select multiple elements based on a specified CSS selector. To iterate over these selected elements, it's crucial to understand the nuances of the resulting NodeList object.
NodeList vs. Array
The issue you faced when using a for...in loop is that NodeList is not an array. While NodeList resembles an array, it possesses additional properties like item() and namedItem(). As a result, a for...in loop iterates over these properties in addition to the elements themselves, leading to the extra 3 items you encountered.
Best Practices for Looping
To avoid this issue and loop correctly, consider the following approaches:
Using a For Loop with Index
<code class="javascript">var checkboxes = document.querySelectorAll(".check"); for (var i = 0; i < checkboxes.length; i++) { // Do something with checkboxes[i] }</code>
Using a ForEach Loop (ES2015)
The forEach() method is designed specifically for iterating over arrays and NodeLists. It simplifies the looping syntax, making it more concise:
<code class="javascript">var checkboxes = document.querySelectorAll(".check"); checkboxes.forEach(function(checkbox) { // Do something with checkbox });</code>
Converting NodeList to Array (ES2015)
Another effective method, particularly for ES2015 environments, is to convert the NodeList to an array using spread syntax:
<code class="javascript">var div_list = document.querySelectorAll("div"); // returns NodeList var div_array = [...div_list]; // converts NodeList to Array div_array.forEach(function(div) { // Do something with div });</code>
Additional Considerations
- For browser compatibility, ensure you use a transpiler like Babel.js if necessary.
- If you work with Node.js, you can leverage the .map() method on NodeLists.
The above is the detailed content of How to Effectively Loop Through Selected Elements Using document.querySelectorAll. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
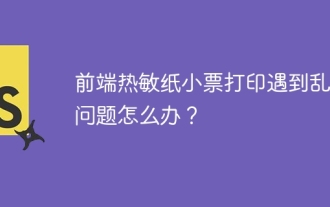
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
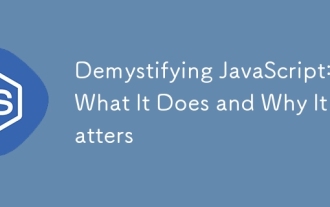
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
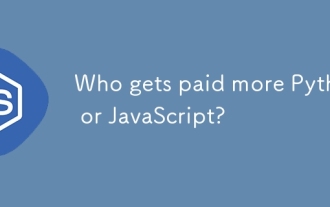
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
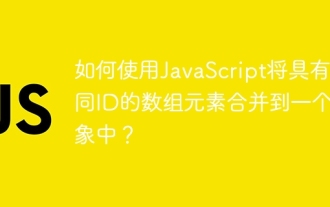
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
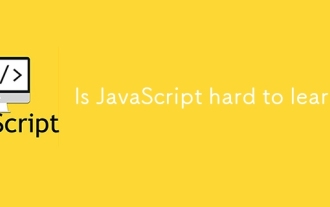
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
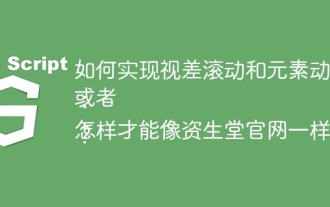
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
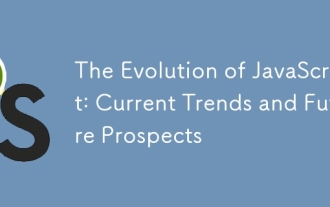
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
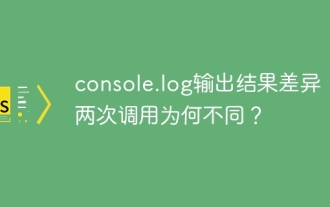
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
