How to Sort HTML Tables with JavaScript: A Comprehensive Solution
Sorting HTML Tables with JavaScript: A Simple Solution Revisited
Finding a suitable table sorting solution in JavaScript can be a challenge. You may need to sort columns alphabetically, including numbers, but without ignoring specific characters or handling currency.
Fortunately, there's a straightforward and effective JavaScript solution that can help:
Plain JavaScript (ES6):
- Supports both alpha and numeric sorting in ascending and descending order
- Works in Chrome, Firefox, Safari, and even IE11
Implementation Details:
- Add a click event listener to each table header cell (th).
- Retrieve all table rows (except the first one) for the table.
- Sort the rows based on the value of the clicked column.
- Reinsert the sorted rows back into the table.
Code Snippet:
<code class="javascript">const getCellValue = (tr, idx) => tr.children[idx].innerText || tr.children[idx].textContent; const comparer = (idx, asc) => (a, b) => ((v1, v2) => v1 !== '' && v2 !== '' && !isNaN(v1) && !isNaN(v2) ? v1 - v2 : v1.toString().localeCompare(v2) )(getCellValue(asc ? a : b, idx), getCellValue(asc ? b : a, idx)); document.querySelectorAll('th').forEach(th => th.addEventListener('click', (() => { const table = th.closest('table'); Array.from(table.querySelectorAll('tr:nth-child(n+2)')) .sort(comparer(Array.from(th.parentNode.children).indexOf(th), this.asc = !this.asc)) .forEach(tr => table.appendChild(tr) ); })));</code>
Styling:
Add the following CSS to style the table and make the header cells clickable:
<code class="css">table, th, td { border: 1px solid black; } th { cursor: pointer; }</code>
Usage:
Include the JavaScript and CSS in your HTML document and add a table with header cells and data rows.
Example:
<code class="html"><table> <tr><th>Country</th><th>Date</th><th>Size</th></tr> <tr><td>France</td><td>2001-01-01</td><td><i>25</i></td></tr> <tr><td><a href="#">spain</a></td><td><i>2005-05-05</i></td><td></td></tr> <tr><td><b>Lebanon</b></td><td><a href="#">2002-02-02</a></td><td><b>-17</b></td></tr> <tr><td><i>Argentina</i></td><td>2005-04-04</td><td><a href="#">100</a></td></tr> <tr><td>USA</td><td></td><td>-6</td></tr> </table></code>
Conclusion:
With this simple and efficient JavaScript solution, you can easily sort HTML tables with a click of a column header, regardless of the data type.
The above is the detailed content of How to Sort HTML Tables with JavaScript: A Comprehensive Solution. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


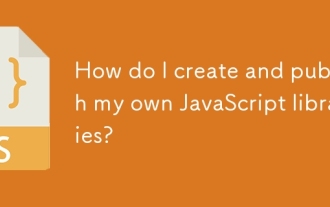
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
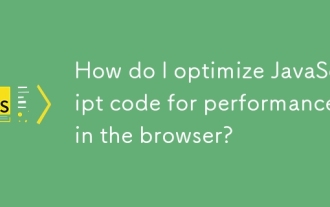
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
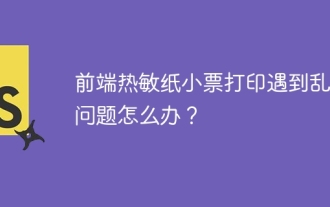
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
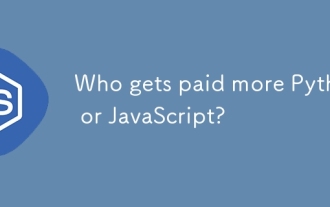
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
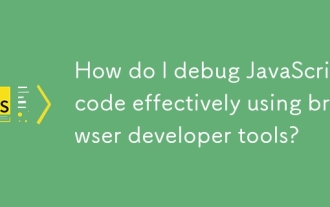
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
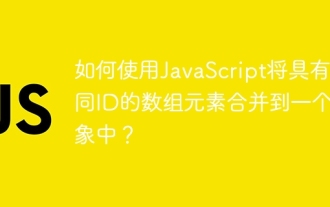
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
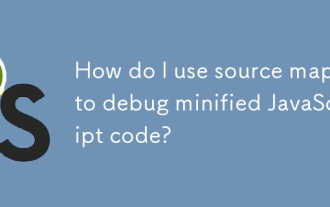
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
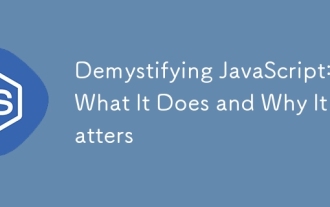
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
