How to Implement Recursion Effectively in Python
Understanding Recursion in Python
Recursion is a programming technique where a function calls itself to solve a problem. In this article, we will focus on implementing recursion in Python to find the sum of integers in a list, as well as other common recursive applications.
List Sum Using Recursion
Suppose we have a function, listSum, that takes a list of integers and returns their sum. Here's its basic recursive implementation:
<code class="python">def listSum(ls): # Base condition: if the list is empty, return 0 if not ls: return 0 # Recursive call with the rest of the list return ls[0] + listSum(ls[1:])</code>
Tail Call Recursion
To optimize the above recursion, we can use tail call recursion. This involves passing the current result along with the list to the recursive call:
<code class="python">def listSum(ls, result): if not ls: return result return listSum(ls[1:], result + ls[0])</code>
Passing Around Index
To avoid creating intermediate lists, we can pass the index of the current element to the recursive call:
<code class="python">def listSum(ls, index, result): if index == len(ls): return result return listSum(ls, index + 1, result + ls[index])</code>
Inner Function Version
If you prefer a more encapsulated approach, you can define an inner function within listSum to handle the recursive logic:
<code class="python">def listSum(ls): def recursion(index, result): if index == len(ls): return result return recursion(index + 1, result + ls[index]) return recursion(0, 0)</code>
Default Parameters
For convenience, you can use default parameters to simplify the function call:
<code class="python">def listSum(ls, index=0, result=0): if index == len(ls): return result return listSum(ls, index + 1, result + ls[index])</code>
Recursive Power Problem
Recursion can also be applied to calculate powers. Consider the power function that takes a base and exponent:
<code class="python">def power(base, exponent): if exponent <= 1: return base return base * power(base, exponent - 1)</code>
Tail Call Optimized Power
To optimize power using tail call recursion:
<code class="python">def power(base, exponent, result=1): if exponent <= 0: return result return power(base, exponent - 1, result * base)</code>
The above is the detailed content of How to Implement Recursion Effectively in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
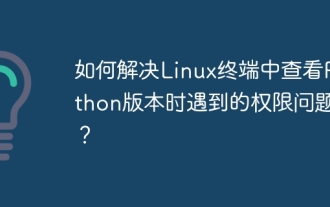
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
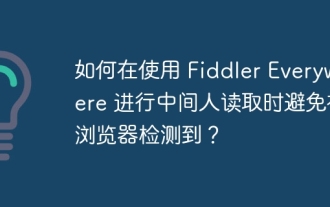
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
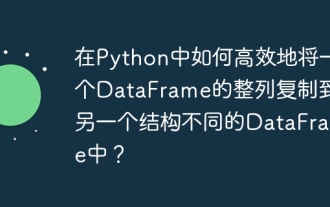
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
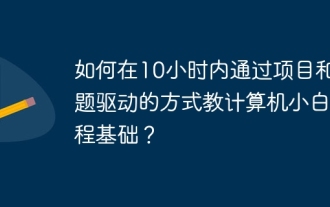
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
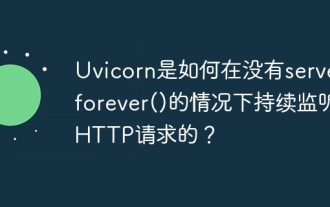
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
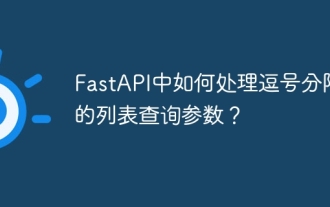
Fastapi ...
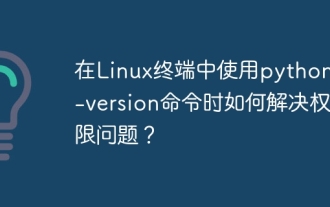
Using python in Linux terminal...
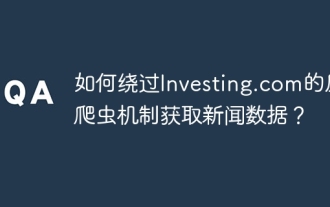
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
