


How to Calculate Sum of List Integers Using Recursion in Python?
Understanding Recursion in Python: Summing List Integers
Recursion is a programming technique where a function calls itself repeatedly to solve smaller instances of a problem until a base condition is reached. In Python, we can apply recursion to various tasks, including calculating sums of list integers.
Recursive Function: listSum
To define a recursive function listSum that takes a list of integers and returns their sum, we can break down the problem as follows:
- Base Condition: If the list is empty (list == []), the sum is 0.
- Recursive Step: If the list is not empty, the sum is the first element (list[0]) plus the sum of the remaining elements (listSum(list[1:])).
Simple Version:
<code class="python">def listSum(ls): # Base condition if not ls: return 0 # First element + result of calling `listsum` with rest of the elements return ls[0] + listSum(ls[1:])</code>
Tail Call Recursion:
To improve efficiency, we can pass the current sum to the function parameter:
<code class="python">def listSum(ls, result): # Base condition if not ls: return result # Call with next index and add the current element to result return listSum(ls[1:], result + ls[0])</code>
Passing Around Index Version:
To avoid creating intermediate lists, we can pass the index of the current element:
<code class="python">def listSum(ls, index, result): # Base condition if index == len(ls): return result # Call with next index and add the current element to result return listSum(ls, index + 1, result + ls[index])</code>
Inner Function Version:
To simplify code, we can define a recursive inner function:
<code class="python">def listSum(ls): def recursion(index, result): # Base condition if index == len(ls): return result # Call with next index and add the current element to result return recursion(index + 1, result + ls[index]) return recursion(0, 0)</code>
Default Parameters Version:
Using default parameters, we can simplify further:
<code class="python">def listSum(ls, index=0, result=0): # Base condition if index == len(ls): return result # Call with next index and add the current element to result return listSum(ls, index + 1, result + ls[index])</code>
The above is the detailed content of How to Calculate Sum of List Integers Using Recursion in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










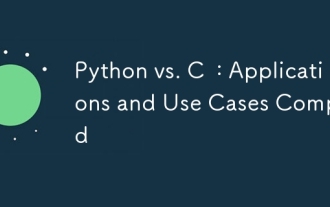
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
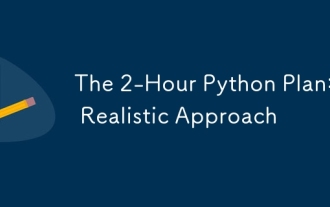
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
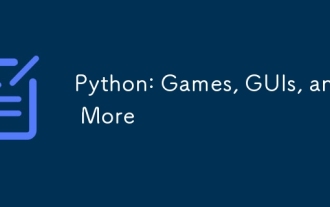
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
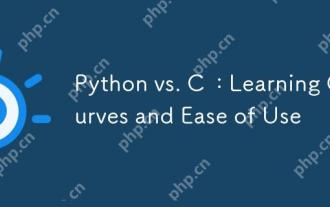
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
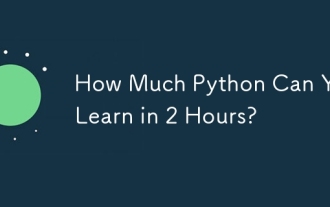
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
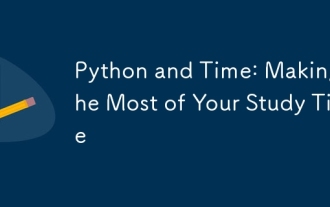
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
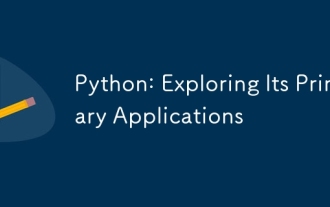
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
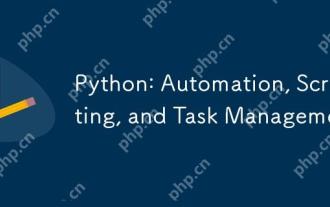
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
