seeing react through java lens
In our coding bootcamp, as we speedran through React and sweated through our labs, our instructor would say - "If you squint, React is a lot like Java."
At first, it was just a catchy and funny phrase. ? However, most recently, I revisited React while working on a personal Google Map calculator project. Days deep into it, I could start to see some of those similarities come to light.
Let’s dive into these connections and see how the foundational concepts of Java can illuminate our understanding of React. ?
Table of Contents
App.jsx as the Java main Class (psvm)
State Management with Hooks as Java Getters and Setters
Containers as Java Classes
Components as Java Methods
React’s Return in Components
Props as Java Method Parameters
Callback Functions as Java Methods that Return Values
1. App.jsx as the Java main Class (psvm)
Java:
In Java, the main class serves as the entry point for the program, and it initiates the execution of the program.
For instance, you might instantiate objects of different classes and invoke their respective methods:
public class Main { public static void main(String[] args) { Home home = new Home(); home.render(); About about = new About(); about.show(); } }
React:
Similarly, in a React application, the App.jsx file plays a comparable role by orchestrating the main application flow.
Just as the main method in Java can call multiple functions, App.jsx is responsible for rendering all the components based on the application's routing and current state.
<Routes> <Route exact path="/" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes>
In the above React example from App.jsx, the components rendered in the return statement mirror the process of calling methods or initializing objects in Java.
In this case, the containers
2. State Management with Hooks as Java Getters and Setters
Java:
In Java, you manage properties with variables and public getter/setter methods to get and set properties of attributes, such as a user's username.
private String username; public String getUsername() { return this.username; } public void setUserData(String username) { this.username = username; }
React:
React’s useState hooks handle application state similarly to how Java uses getter and setter methods to manage object properties.
The useState hook in React allows you to declare state variables that can change over time, much like Java's instance variables in a class.
const [username, setUsername] = useState("");
In the example above:
- setUserName serves as the setter method, allowing updates to the username. While useState("") means username is initialized as an empty string, the setUserName updates the value.
Below we have a function handleInputChange that detects a change in a web form to update user info and updates the value of username to what the user inputs.
public class Main { public static void main(String[] args) { Home home = new Home(); home.render(); About about = new About(); about.show(); } }
- You can think of accessing username as a getter.
Whenever you reference username in a component, you are effectively using a getter to access its value. For example, my webpage could render the username by:
<Routes> <Route exact path="/" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes>
3. Containers as Java Classes
Java:
In Java, classes group related tasks and data together. They help manage how information flows in your application.
In this example, the Calculator class handles calculations and stores the result.
private String username; public String getUsername() { return this.username; } public void setUserData(String username) { this.username = username; }
React:
Similarly, in React, containers play a key role by connecting the application's data to the components. They handle things like fetching data from API calls and managing the app's state.
In this example, the Calculator container manages the state of the input value and the result,
const [username, setUsername] = useState("");
4. Components as Java Methods
Java:
Methods in Java perform specific actions, such as handling user input. These methods can be invoked as needed to facilitate various functionalities in your application.
const handleInputChange = (event) => { setUserName(event.target.value); };
React:
Just like Java methods are small, focused tasks, React Components serve a similar purpose, acting as the fundamental building blocks of your user interface.
Each component is crafted for a specific functionality and can be reused throughout the application.
The ManualFilter component below is solely focused on filtering options for users. It presents checkboxes that allow users to select specific categories.
This component can then be called within a UserForm container page.
<p>Welcome to our page {username}</p>
5. React’s Return in Components
Java:
In Java, a method might return a value that another part of the program uses to generate output.
For example, the renderOutput method returns a string containing the user's goal, which can then be displayed elsewhere in the program.
public class Calculator { private int result; public void calculateSum(int a, int b) { result = a + b; } public int getResult() { return result; } }
React:
The return statement in React components is crucial for rendering the user interface. In React, what you return from a component dictates what the user sees on the screen.
This is similar to how as aforementioned, a method in Java that returns data intended for processing or display in another part of the program.
In this example, the UserGoal component returns a paragraph element that displays the user's goal.
public class Main { public static void main(String[] args) { Home home = new Home(); home.render(); About about = new About(); about.show(); } }
6. Props as Java Method Parameters
Java:
You can passing arguments to a Java method, where the arguments can affect the state or behavior of the calling object.
For example, consider a simple Java method that takes a message as a parameter. The message it receives will affect what the console will show.
<Routes> <Route exact path="/" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes>
React:
In React, components can receive props, which are similar to parameters in Java methods. React components use props to determine their content and functionality.
Props control how components behave and what data they display.
Let's say we have a parent component called WelcomePage that will pass a message to the MessageDisplay child component.
In other words, imagine a MessageDisplay as a section on the WelcomePage landing page where a message shows.
We can define a message in the parent component and pass it as a prop to the MessageDisplay component:
private String username; public String getUsername() { return this.username; } public void setUserData(String username) { this.username = username; }
The MessageDisplay component will receive this prop and render it:
const [username, setUsername] = useState("");
7. Callback Functions as Java Methods that Return Values
Java:
In Java, you often have methods within classes that perform specific actions and return values to their caller. For example, you might have a class named Calculator with a method that calculates the difference between two numbers:
const handleInputChange = (event) => { setUserName(event.target.value); };
^In another class you create an instance of the Calculator class and call that method.
React:
React follows a similar concept, but it focuses on the relationship between components.
When you have a parent component that contains child components, callback functions help facilitate communication between them. (Remember: parent is a main container that holds the other components - similar to our earlier example of a parent "landing page" with a sub-component of a message box)
For instance, let’s say you have a ChildComponent that needs to send some calculated data back to its parent component.
Below we pass the handleCalculationResult function from the parent to the child as a prop.
This function acts like a callback:
<p>Welcome to our page {username}</p>
You can see below how onCalculate is a callback function received in the ChildComponent from the parent component.
When the button in ChildComponent is clicked, it performs the calculation and uses onCalculate to send the result back to the parent. This mimics how Java methods return values to their callers.
public class Calculator { private int result; public void calculateSum(int a, int b) { result = a + b; } public int getResult() { return result; } }
In this way, the parent manages the overall application state and behavior while the child focuses on a specific action (in this case, the calculation).
The above is the detailed content of seeing react through java lens. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










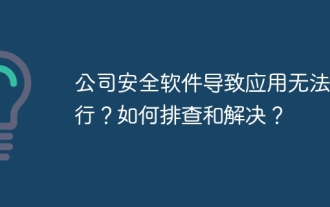
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
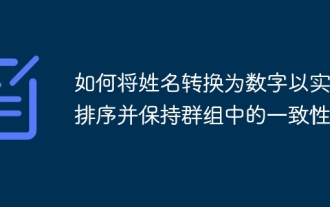
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
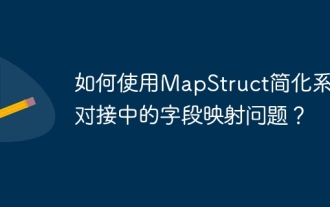
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
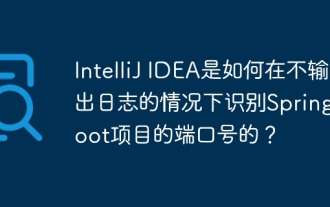
Start Spring using IntelliJIDEAUltimate version...
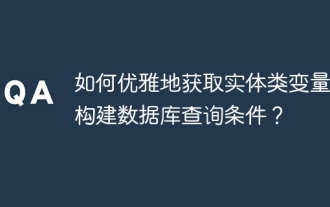
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
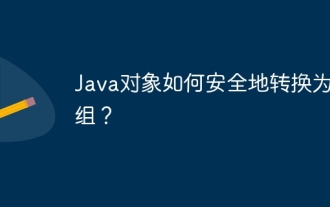
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
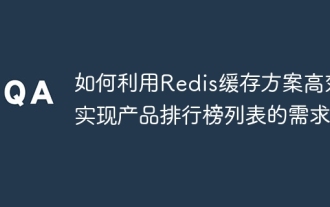
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
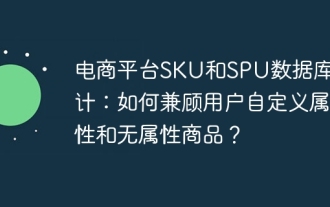
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
