How to Pause Node.js Functions Until Callback Execution
Strategies for Halting Node.js Functions Until Callback Execution
In Node.js, the asynchronous nature of event-driven programming requires a different approach when waiting for callbacks. Instead of employing synchronous blocking techniques, this article explores efficient alternatives for making your functions pause until the appointed time.
Understanding the Asynchronous Paradigm
Unlike traditional blocking functions, Node.js functions that initiate asynchronous operations immediately invoke the callback upon completion, without waiting for the response. To exemplify this:
<code class="javascript">function(query) { myApi.exec('SomeCommand', function(response) { return response; }); }</code>
This code may raise an error since the function returns immediately, while the callback hasn't yet been invoked. The function execution flow continues, rendering the callback response inaccessible.
The Proper Approach: Callback-Based Chaining
The recommended solution in Node.js lies in embracing the callback-based approach. Instead of attempting to block execution, your functions should accept a callback parameter that will be invoked when the operation is complete.
<code class="javascript">function(query, callback) { myApi.exec('SomeCommand', function(response) { callback(response); }); }</code>
Now, the function execution flow doesn't wait for the callback response; instead, it immediately invokes the callback, which can then be handled by the caller.
Usage Pattern
Instead of relying on a traditional return value, the modified function requires the caller to provide a callback function that will receive the response:
<code class="javascript">myFunction(query, function(returnValue) { // Process the return value here });</code>
By employing this callback-based approach, you can effectively pause your functions until the desired callbacks are invoked, allowing your Node.js applications to maintain their asynchronous and efficient design philosophy.
The above is the detailed content of How to Pause Node.js Functions Until Callback Execution. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










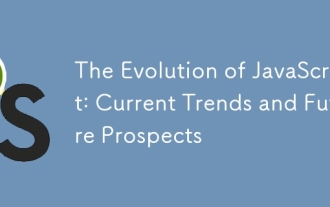
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
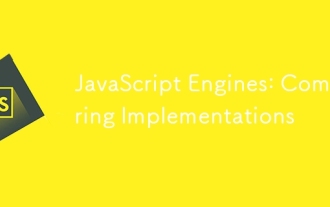
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
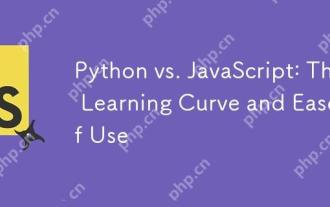
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
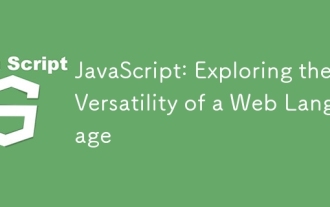
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
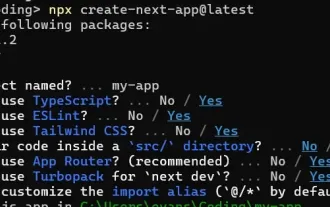
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
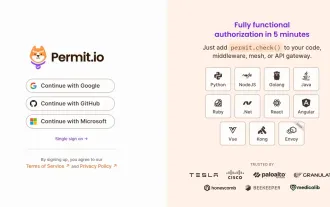
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
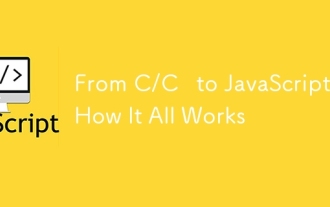
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
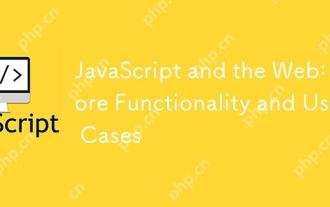
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
