How to Reliably Access Asynchronous Return Values with jQuery?
JavaScript Asynchronous Return Value with jQuery
Question:
How can we reliably access the GUID value returned from an asynchronous jQuery function?
Answer:
Asynchronous calls, by their nature, cannot provide a return value. jQuery's asynchronous functions return immediately, meaning the value they produce is not available when the function returns.
Solution:
There are two main approaches to handle this challenge:
1. Callback Functions:
This involves passing a callback function to the asynchronous function, which receives the result when it becomes available.
<code class="javascript">function trackPage() { var elqTracker = new jQuery.elq(459); elqTracker.pageTrack({ success: function() { elqTracker.getGUID(function(guid) { // Handle the GUID here alert(guid); }); } }); }</code>
2. Promises:
jQuery's deferred objects (promises) allow you to create asynchronous logic that returns a promise. Callbacks can be attached to these promises to receive the result when it becomes available.
<code class="javascript">function trackPage() { var elqTracker = new jQuery.elq(459); var dfd = $.Deferred(); elqTracker.pageTrack({ success: function() { elqTracker.getGUID(function(guid) { dfd.resolve(guid); }); } }); return dfd.promise(); } // Usage: trackPage().done(function(guid) { alert("Got GUID: " + guid); });</code>
Additional Notes:
- Return values should be declared in the outer scope of the asynchronous function to ensure proper access.
- jQuery's AJAX module also returns promises, providing consistency in asynchronous logic handling.
- Promises allow for chaining multiple callbacks, offering flexibility in handling results.
The above is the detailed content of How to Reliably Access Asynchronous Return Values with jQuery?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


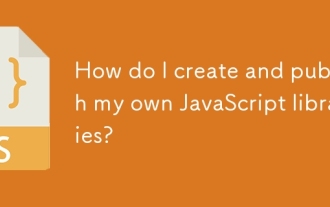
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
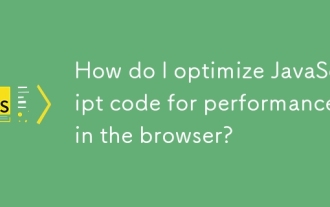
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
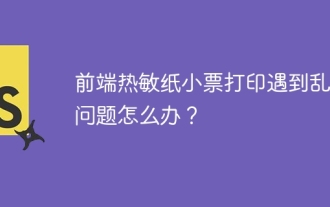
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
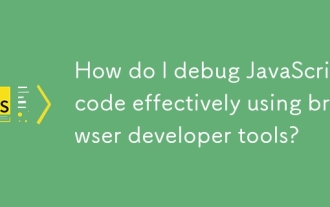
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
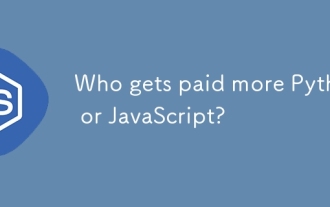
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
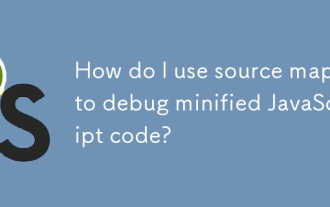
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
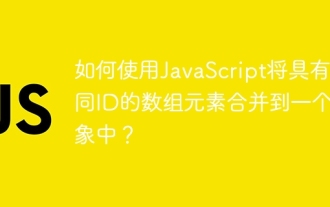
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
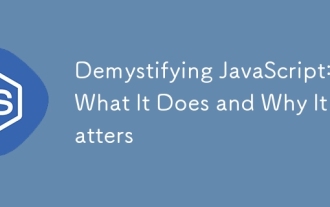
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
