


Mastering Closures in JavaScript: Understanding Scope, Encapsulation, and Performance
Closure
Definition
Closure is a feature that allows the functions to access all other variables and functions which are declared in same scope level (lexical scope).
- Closure is a feature that allows the functions to access all other variables and functions which are declared in same scope level (lexical scope).
- Closure will be created when a function is defined within another function (outer function and inner function). The inner function will create a closure that provides access to inner function to all variables and functions created in that outer function.
- Closures allow the inner function to access variables declared in the outer function, even after the outer function has returned.
- We have to handle the memory properly, closure can lead to both memory leak and better memory management.
-
Closures in JavaScript serve a similar purpose to private methods in Java by allowing you to create private variables and encapsulate functionality.
Example
function outerFunction() { let outerVariable = 'I am from outer scope'; function innerFunction() { console.log(outerVariable); // Accessing outerVariable from the outer scope } return innerFunction; // Return the inner function } const closureFunction = outerFunction(); // Call outerFunction, which returns innerFunction closureFunction(); // Outputs: I am from outer scope
Copy after login- See in the above example we calling inner function only because it is only returned. Eventhough we have access to outerVariable.
- Let’s see another best example
function handleCount() { let count = 0; return { increment: () => { count++; return count; }, decrement: () => { count--; return count; }, getCount: () => { return count; }, }; } const counter = handleCount(); console.log(counter.increment()); // Outputs: 1 console.log(counter.increment()); // Outputs: 2 console.log(counter.getCount()); // Outputs: 2 console.log(counter.decrement()); // Outputs: 1 console.log(counter.getCount()); // Outputs: 1
Copy after login- Now for every time we called increment, decrement, getCount not handleCount will get initiated only the called function will get executed but also we have access to the variables outside of it scope. This is called as closure.
Key points to remember
- Definition: A closure is a function that retains access to its lexical scope, even when the function is executed outside that scope.
- Scope Chain: Closures are created when a function is defined within another function. The inner function forms a closure that includes the outer function’s scope.
- Access to Variables: Closures allow the inner function to access variables declared in the outer function, even after the outer function has returned.
- Private Variables: Closures are often used to create private variables. This means that variables can be hidden from the global scope and only accessed through the closure.
- Memory Management: Closures can lead to better memory efficiency, as they retain only the variables that are needed for execution.
- Performance Considerations: While closures are powerful, they can also lead to memory leaks if not managed properly, especially in long-lived objects or event listeners.
Use cases
- Encapsulation: To create private data or methods.
- Function Factories: To create functions with preset parameters.
- Callbacks: For asynchronous programming (like in event handlers or setTimeout).
- CSS: rem and em. rem is derived from root element (html / body) but em is derived from its near parent.
Common interview questions
- What is a closure, and how does it work?
- Can you explain the difference between a closure and a regular function?
- Provide an example of a closure in JavaScript.
- What are the use cases of closures in JavaScript?
- How can closures lead to memory leaks, and how can you prevent them?
The above is the detailed content of Mastering Closures in JavaScript: Understanding Scope, Encapsulation, and Performance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










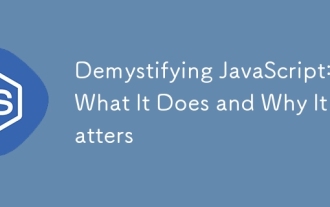
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
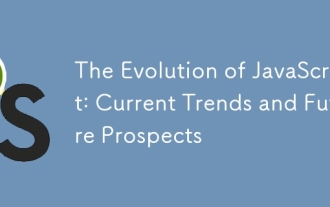
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
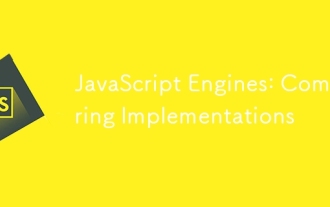
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
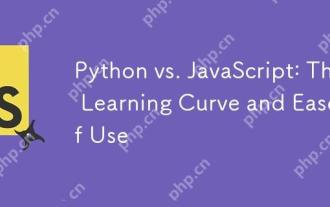
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
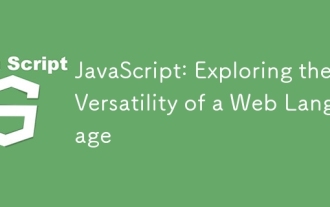
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
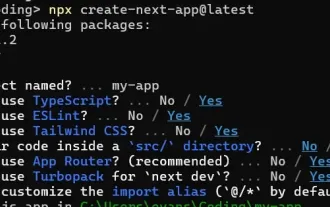
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
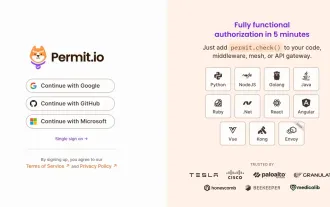
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
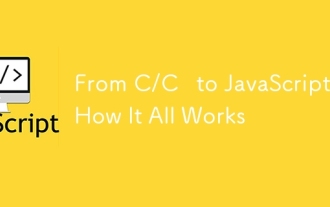
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
