How to Upload Files to AWS Ssing Java: A Step-by-Step Guide
Introduction
Amazon Simple Storage Service (S3) is a powerful and scalable object storage service providing a reliable and cost-effective solution to store and retrieve any amount of data from anywhere on the web. In this article, we will explore how to interact with AWS S3 to upload files using Java and Spring Boot.
Prerequisites
Before diving into the code, ensure you have the following:
- An AWS Account: Sign up for an account on the AWS Website
- Java Development Environment: Ensure you have Java installed on your machine along with a build tool like Maven or Gradle for dependency management.
- Basic Knowledge of Java: Familiarity with Java syntax and programming concepts will be helpful.
Setting Up AWS SDK for Java
To interact with AWS S3 using Java, you'll need the AWS SDK for Java. Here's how to add it to your project:
For Maven: Add the following dependency to your pom.xml file:
1 2 3 4 5 |
|
For Gradle: Add the following line to your build.gradle file:
1 |
|
Configuring AWS Credentials
To securely interact with AWS S3, you'll need to store your AWS credentials. In this tutorial, we'll use the application.properties file to manage credentials.
First, add the following lines to your application.properties file:
1 2 3 |
|
Next, in your Java application, you can load these properties and use them to configure the AWS S3 client:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
|
Uploading Files to S3
Here is a sample method that demonstrates how to upload a file to AWS S3 using the AmazonS3 client.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
|
uploadFile() Method Breakdown:
Parameters:
- bucketName: The name of the S3 bucket to upload the file to.
- filePath: The local path to the file being uploaded.
File Creation & Existence Check:
- A File object is created from the provided filePath
- The method checks if the file exists using file.exists() If the file doesn't exist, it logs an error message.
Uploading the File:
- If the file exists, it's uploaded to the S3 bucket using the putObject() method.
- Logs a success message if the upload completes successfully.
Conclusion
In this article, we covered the process of uploading files to AWS S3 using Java. We explored how to configure AWS credentials, set up the S3 client using Spring Boot, and wrote a simple method to upload files to your S3 bucket. With this foundation, you can now integrate S3 file uploads into your Java applications seamlessly.
The above is the detailed content of How to Upload Files to AWS Ssing Java: A Step-by-Step Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










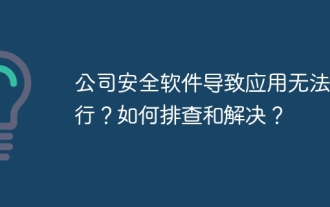
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
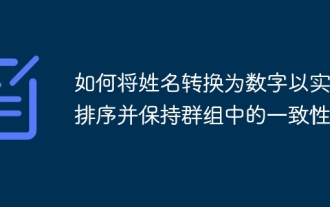
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
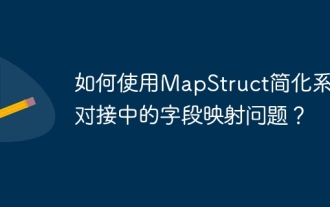
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
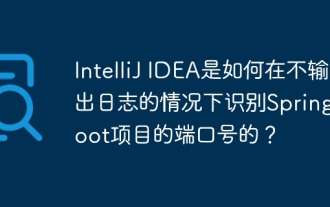
Start Spring using IntelliJIDEAUltimate version...
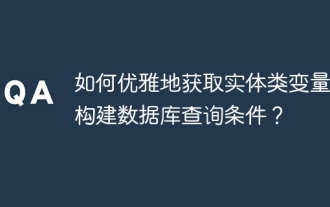
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
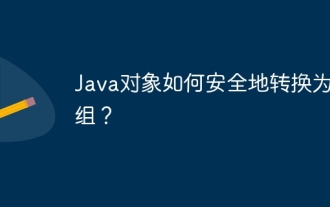
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
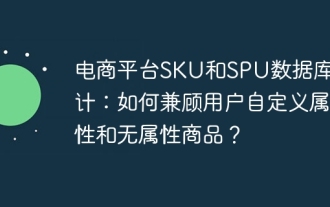
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
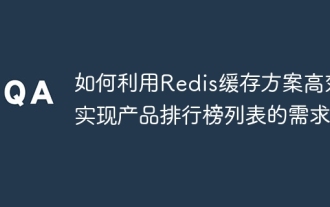
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
