When Method Overloading Doesn\'t Work in Python?
Method Overloading in Python
In Python, method overloading is the ability to define multiple methods with the same name but different parameters. However, this can lead to some unexpected behavior.
Example 1:
<code class="python">class A: def stackoverflow(self): print ('first method') def stackoverflow(self, i): print ('second method', i)</code>
If you call the method with an argument, the second method will be invoked:
<code class="python">ob=A() ob.stackoverflow(2) # Output: second method 2</code>
But if you call it without an argument, Python will raise an error:
<code class="python">ob=A() ob.stackoverflow() # Output: TypeError: stackoverflow() takes exactly 2 arguments (1 given)</code>
This is because Python considers the first method as having no arguments, not one default argument.
Solution:
To solve this issue, you can use default parameter values:
<code class="python">class A: def stackoverflow(self, i='some_default_value'): print('only method')</code>
Now, both calls will work:
<code class="python">ob=A() ob.stackoverflow(2) # Output: only method ob.stackoverflow() # Output: only method</code>
Advanced Overloading with Single Dispatch
Python 3.4 introduced single dispatch generic functions, which allow you to define specific behaviors for different argument types:
<code class="python">from functools import singledispatch @singledispatch def fun(arg, verbose=False): if verbose: print("Let me just say,", end=" ") print(arg) @fun.register(int) def _(arg, verbose=False): if verbose: print("Strength in numbers, eh?", end=" ") print(arg) @fun.register(list) def _(arg, verbose=False): if verbose: print("Enumerate this:") for i, elem in enumerate(arg): print(i, elem)</code>
This allows you to call fun with different argument types and get the appropriate behavior:
<code class="python">fun(42) # Output: Strength in numbers, eh? 42 fun([1, 2, 3]) # Output: Enumerate this: # 0 1 # 1 2 # 2 3</code>
The above is the detailed content of When Method Overloading Doesn\'t Work in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










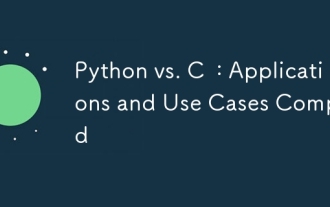
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
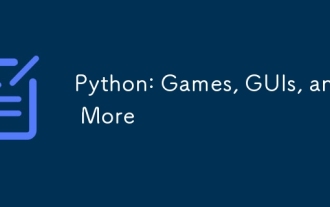
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
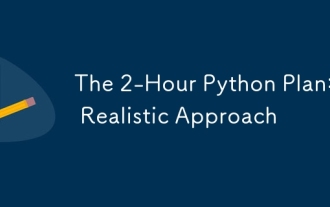
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
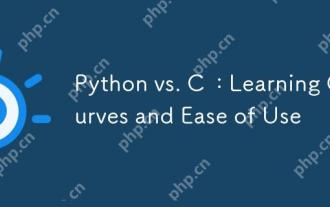
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
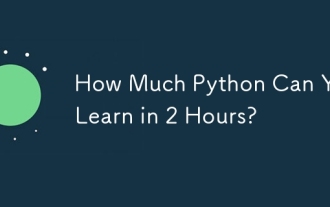
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
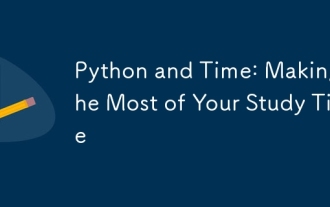
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
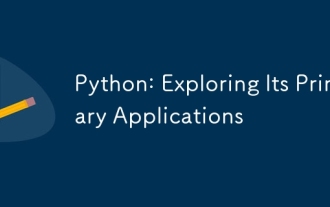
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
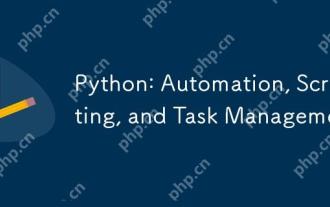
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
