Java Debugging: A Comprehensive Guide
Debugging is an essential skill for Java developers, as it allows you to inspect and resolve issues in your code. Whether you're dealing with logical errors, unexpected crashes, or performance issues, knowing how to effectively debug Java applications is crucial.
In this guide, we will explore Java debugging techniques, tools, and best practices that can help you track down bugs and improve the quality of your software.
Table of Contents
- 1. Understanding Debugging
- 2. Common Java Debugging Tools
-
3. Basic Debugging in an IDE
- 3.1. Setting Breakpoints
- 3.2. Step Execution
- 3.3. Inspect Variables
- 3.4. Watches and Expressions
- 4. Remote Debugging
- 5. Debugging with Logs
-
6. Advanced Debugging Techniques
- 6.1. Exception Breakpoints
- 6.2. Conditional Breakpoints
- 6.3. Hot Code Replace (HCR)
- 6.4. Thread Debugging
- 7. Best Practices for Debugging Java Applications
- 8. Conclusion
1. Understanding Debugging
Debugging is the process of identifying, analyzing, and fixing bugs or errors in your software code. In Java, bugs can range from syntax errors (caught at compile time) to logical errors (detected at runtime), performance bottlenecks, or issues that arise only under specific conditions.
The Java Virtual Machine (JVM) provides several debugging features, and most modern IDEs, such as IntelliJ IDEA and Eclipse, offer built-in debugging tools that help developers inspect the runtime behavior of their applications.
2. Common Java Debugging Tools
Here are some popular Java debugging tools:
- IntelliJ IDEA: Offers a powerful debugger with features like breakpoints, variable inspection, step-through execution, and remote debugging.
- Eclipse IDE: A widely-used Java IDE with robust debugging capabilities including hot code replacement, thread debugging, and expression evaluations.
- JDB (Java Debugger): A command-line tool provided by the JDK that allows you to debug Java applications in environments where graphical interfaces are unavailable.
- VisualVM: A monitoring and debugging tool that can profile applications and analyze memory usage.
- JProfiler: A commercial profiling and debugging tool for performance monitoring and memory analysis in Java applications.
- JConsole: Used to monitor JVM performance metrics and detect issues like memory leaks.
3. Basic Debugging in an IDE
3.1. Setting Breakpoints
A breakpoint pauses the execution of your program at a specific line, allowing you to inspect the state of your application at that point.
How to Set a Breakpoint:
- In IntelliJ IDEA: Click in the gutter next to the line number where you want to set a breakpoint. A red dot indicates the breakpoint.
- In Eclipse: Similarly, click in the left margin of the code editor next to the line you want to pause.
When the execution reaches a breakpoint, the IDE halts the program, allowing you to explore the current state of the variables and program flow.
3.2. Step Execution
Once execution stops at a breakpoint, you can step through your code to understand its flow:
- Step Over: Moves to the next line in the same method, skipping over method calls.
- Step Into: Enters the method or function being called.
- Step Out: Exits the current method and returns to the caller.
3.3. Inspect Variables
When the program execution is paused, you can inspect the values of variables at that point in time.
- In most IDEs, you can hover over variables to see their current values.
- IDEs also provide a Variables pane where you can inspect all the variables in the current scope, including local variables and class members.
3.4. Watches and Expressions
You can also create watches to track the value of specific expressions or variables. This is useful when you want to observe how a variable changes as the code executes.
4. Remote Debugging
Remote debugging allows you to debug applications running on a different machine or environment (e.g., a production server) by connecting your IDE to the remote JVM.
Steps to Enable Remote Debugging:
- Add the following JVM options to the application you want to debug remotely:
-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=5005
- address=5005: This is the port on which the debugger will listen.
- suspend=n: Setting this to y will make the JVM wait until the debugger is attached before continuing execution.
- In your IDE (IntelliJ or Eclipse):
- Create a Remote Debugging Configuration.
- Specify the host and port (e.g., localhost:5005).
- Start the remote debugging session in the IDE.
This allows you to inspect, set breakpoints, and step through the code as if it were running locally.
5. Debugging with Logs
Logging is one of the most common and effective ways to debug an application, especially in production environments where debuggers cannot be attached.
Java provides the following logging frameworks:
- Log4j: A popular logging library that allows you to output logs at different levels (INFO, DEBUG, WARN, ERROR).
- SLF4J: A logging facade that works with various backends like Log4j and Logback.
- java.util.logging: The built-in Java logging API.
Example usage with SLF4J:
import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class MyClass { private static final Logger logger = LoggerFactory.getLogger(MyClass.class); public static void main(String[] args) { logger.info("Application started"); logger.debug("Debugging value: {}", someVariable); logger.error("An error occurred", exception); } }
Logging helps you track the application's behavior without needing to stop the application or attach a debugger.
6. Advanced Debugging Techniques
6.1. Exception Breakpoints
Exception breakpoints pause the execution when a specific type of exception is thrown, regardless of where it occurs in the code. This is useful for identifying where and why certain exceptions are raised.
In most IDEs (like IntelliJ or Eclipse):
- Go to the Breakpoints view.
- Add an Exception Breakpoint for a specific exception, such as NullPointerException.
6.2. Conditional Breakpoints
Sometimes you want to halt execution only when certain conditions are met. Conditional breakpoints allow you to specify conditions (e.g., variable values or states) under which the execution should stop.
How to set a conditional breakpoint:
- Right-click on a breakpoint and add a condition, such as x == 5. The program will only stop when this condition is true.
6.3. Hot Code Replace (HCR)
Hot Code Replace allows you to modify and apply changes to your code during a debugging session without restarting the application. This is especially useful in long-running applications or environments where frequent restarts are disruptive.
Most IDEs, like IntelliJ and Eclipse, support HCR when debugging. However, it has limitations: you cannot modify class structures (e.g., adding new methods or changing class hierarchy).
6.4. Thread Debugging
Java applications can have multiple threads running concurrently, and debugging multi-threaded applications can be tricky.
In the Debugger window of your IDE, you can:
- Inspect all running threads.
- Pause, resume, or terminate individual threads.
- Set breakpoints specific to a thread to analyze thread-specific behavior.
7. Best Practices for Debugging Java Applications
- Reproduce the Issue: Before debugging, ensure that you can consistently reproduce the bug or issue. This makes it easier to track down the problem.
- Use Logging: Even when using a debugger, logs provide a valuable history of events, especially when you’re dealing with issues in production or multi-threaded environments.
- Start with Simple Breakpoints: Don’t set too many breakpoints at first. Start by focusing on critical areas of the code where you suspect the issue originates.
- Use Conditional Breakpoints: Avoid stopping the program unnecessarily by using conditions on breakpoints to halt execution only when certain criteria are met.
- Don’t Overuse Debugging: Debugging can be time-consuming. Sometimes, stepping back and reviewing your code logically or using tests may help pinpoint issues faster.
- Focus on Edge Cases: Bugs often arise in edge cases, such as handling null values, out-of-bound errors, or thread synchronization issues.
8. Conclusion
Debugging is a key skill for every Java developer. Whether you’re using an IDE like IntelliJ IDEA or Eclipse, remote debugging a production environment, or relying on logs, understanding the tools and techniques for debugging is critical. By following the best practices mentioned in this guide and mastering advanced debugging techniques, you can improve your efficiency in identifying and fixing bugs, leading to higher quality and more stable software.
? Author
Join Our Telegram Community || Follow me on GitHub for more DevOps content!
The above is the detailed content of Java Debugging: A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










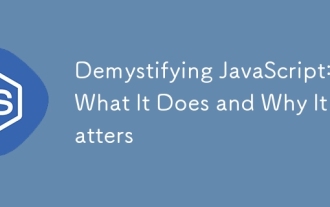
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
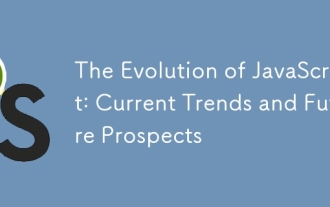
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
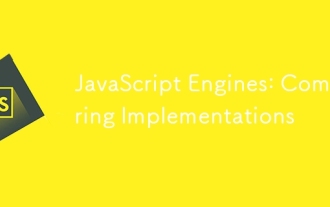
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
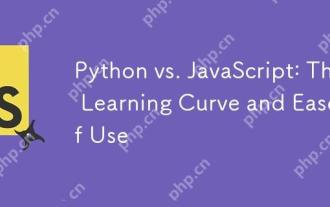
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
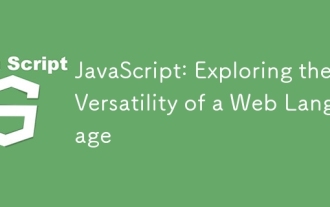
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
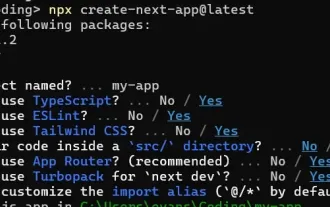
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
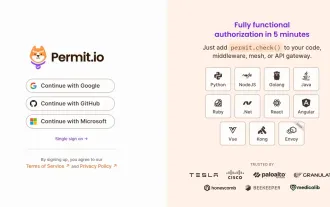
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
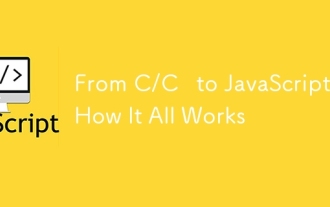
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
