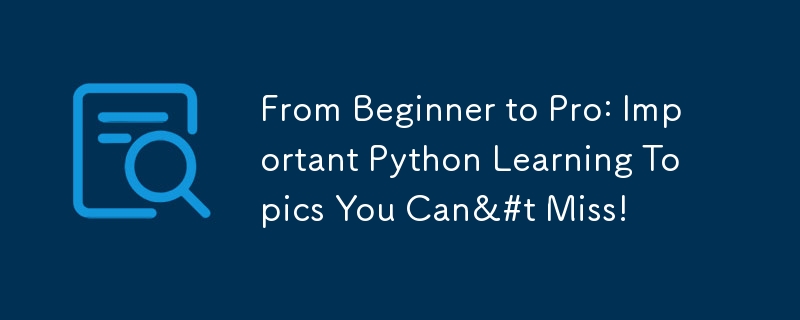
Hey guys! If you’re starting to learn Python, great choice! I found some cool stats about it, and while looking for a good syllabus, I noticed some topics come up a lot. So, I made a beginner friendly Python syllabus that covers all the key concepts. I hope you like it!
1. Introduction to Python
- What is Python?
- Installing Python
- Running Python scripts
- Python IDEs (Integrated Development Environments)
- Basic Syntax: Comments, Indentation, and Variables
- Python Data Types: Strings, Integers, Floats, Booleans
- Basic Input and Output
- Python's Interactive Mode and REPL
- Using Jupyter Notebooks
- Understanding the Python Shell
- Basic Troubleshooting: Common Errors and Fixes
2. Control Flow
- Conditional Statements: if, else, elif
- Comparison and Logical Operators
- Loops:
-
for loops
-
while loops
- Loop control statements: break, continue, pass
- List and Dictionary Comprehensions
- Nested Loops
- Using enumerate() with Loops
- The zip() Function for Iteration
- Error Handling in Loops
3. Functions
- Defining Functions with def
- Parameters and Arguments
- Return Values
- Variable Scope: Local vs Global
- Lambda Functions
- Recursion
- Default and Keyword Arguments
-
Variable-length Arguments (*args and `kwargs`)**
- Higher-order Functions
- Decorators (basic introduction)
4. Data Structures
- Lists:
- Indexing, Slicing, and Methods (append, insert, remove, etc.)
- Tuples:
- Immutability and Use Cases
- Dictionaries:
- Key-Value Pairs, Methods (get, keys, values, etc.)
- Sets:
- Set Operations (union, intersection, difference)
- Nested Data Structures
- List vs. Tuple vs. Set vs. Dictionary
- Understanding collections module: Counter, defaultdict, OrderedDict
- Data Structure Performance Considerations
5. Object-Oriented Programming (OOP)
- Classes and Objects
- Attributes and Methods
- The self Keyword
- Constructors (__init__)
- Inheritance
- Single and Multiple Inheritance
- Polymorphism
- Encapsulation and Abstraction
- Special Methods: str, repr, len, etc.
- Class vs. Instance Variables
- Class Methods and Static Methods
- Composition vs. Inheritance
- Abstract Base Classes (ABCs)
6. Error Handling
- Types of Errors: Syntax, Logic, Runtime
-
try, except, finally blocks
- Raising Exceptions with raise
- Custom Exception Classes
- Using assert for Debugging
- Logging Errors with the logging Module
- Creating Context Managers for Error Handling
- Best Practices in Error Handling
7. File Handling
- Opening Files: open(), read(), write()
- Reading and Writing to Files
- File Modes (r, w, a, b)
- Working with File Paths
- Using with to Automatically Close Files
- Reading and Writing CSV Files
- Working with JSON Files
- File Iterators
- Handling Large Files with Buffered Reading/Writing
8. Modules and Packages
- Importing Modules: import, from ... import
- Python Standard Library (e.g., math, random, datetime)
- Creating and Using Custom Modules
- Using Third-Party Packages with pip
- Virtual Environments
- Understanding the __init__.py file
- Building Your Own Package
- Using requirements.txt for Dependency Management
- Exploring the sys and os Modules
9. Working with Libraries
- NumPy (for array manipulation)
- Pandas (for data analysis and manipulation)
- Matplotlib and Seaborn (for data visualization)
- Requests (for handling HTTP requests)
- JSON Handling
- Using SciPy for Scientific Computing
- Working with SQLAlchemy for Database Interaction
- Web Scraping with Beautiful Soup and Scrapy
- Introduction to TensorFlow and Keras for Machine Learning
10. Advanced Topics
- List and Dictionary Comprehensions (advanced usage)
- Generators and yield keyword
- Decorators and @decorator_name
- Context Managers
- Regular Expressions (Regex)
- Unit Testing with unittest
- Metaclasses and their Use Cases
- Asynchronous Programming (async/await)
- Threading and Multiprocessing
- Python’s functools module (e.g., lru_cache, partial)
- Descriptors and Property Decorators
- Type Hinting and Annotations
- Advanced Error Handling and Custom Exceptions
11. Working with APIs
- What are APIs?
- Consuming APIs with Python
- Authentication (Basic, OAuth)
- Parsing JSON from APIs
- Using the requests Library for API Calls
- Working with REST vs. SOAP APIs
- Handling API Rate Limiting
- Creating Your Own API with Flask or FastAPI
12. Introduction to Data Science
- Basics of Data Manipulation with Pandas
- Data Visualization with Matplotlib/Seaborn
- Basic Statistics in Python
- Introduction to Machine Learning with Scikit-learn (optional)
- Exploratory Data Analysis (EDA)
- Feature Engineering and Selection
- Data Cleaning Techniques
- Understanding Overfitting and Underfitting
13. Final Project
- Develop a Python project that integrates different concepts:
- Data Analysis, Web Scraping, or a Simple Game
- Project Planning and Documentation
- Version Control with Git
- Deployment Options (e.g., Heroku, GitHub Pages)
- Presenting Your Project: Best Practices
Resources to Learn Python:
- Learn Python Free
- Kaggel Course on Python
- CodeAcacdmy Adv Python Course
- Official Python DOC
If you have any suggestions or if I missed something, just drop a comment! Happy coding!
The above is the detailed content of From Beginner to Pro: Important Python Learning Topics You Cant Miss!. For more information, please follow other related articles on the PHP Chinese website!