


How to Subtract One List from Another Efficiently in Python?
Subtracting One List from Another: Efficient Techniques and Custom Implementation
Subtracting one list from another is a common operation in programming. In Python, performing this operation directly using the - operator can be limiting. To effectively subtract lists, consider the following approaches:
List Comprehension
To subtract one list (y) from another (x) while preserving the order of elements in x, use a list comprehension:
<code class="python">[item for item in x if item not in y]</code>
This approach iterates over each element in x and includes it in the new list only if it's not present in y.
Set Difference
If the order of elements is not crucial, a more efficient approach is to use a set difference:
<code class="python">list(set(x) - set(y))</code>
This method creates a set from each list, performs a subtraction on them, and converts the resulting set back to a list. It's faster than list comprehension but doesn't maintain the original order.
Custom Class
To allow subtraction syntax (x - y) to work directly on lists, one can create a custom class:
<code class="python">class MyList(list): ... def __sub__(self, other): ...</code>
Overriding the __sub__ method enables custom subtraction behavior, providing the desired functionality.
Example Usage:
<code class="python">x = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] y = [1, 3, 5, 7, 9] # List Comprehension result_comprehension = [item for item in x if item not in y] print(result_comprehension) # [0, 2, 4, 6, 8] # Set Difference result_set = list(set(x) - set(y)) print(result_set) # [0, 2, 4, 6, 8] # Custom Class class MyList(list): ... x_custom = MyList([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]) y_custom = MyList([1, 3, 5, 7, 9]) result_custom = x_custom - y_custom print(result_custom) # [0, 2, 4, 6, 8]</code>
These approaches provide different ways to subtract lists in Python, depending on the specific requirements and desired behavior.
The above is the detailed content of How to Subtract One List from Another Efficiently in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




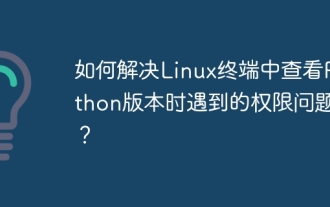
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
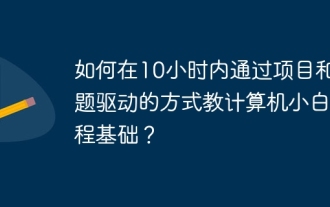
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
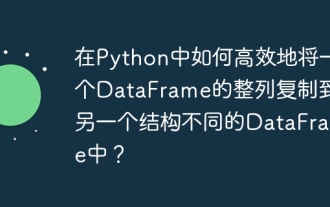
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
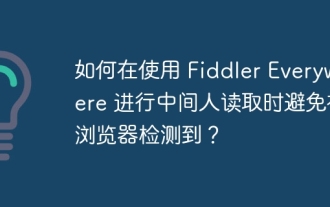
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
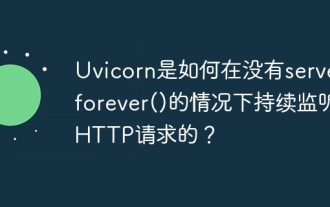
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
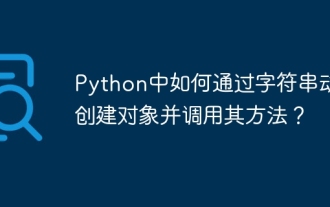
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
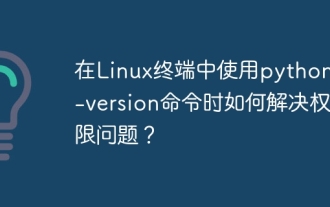
Using python in Linux terminal...
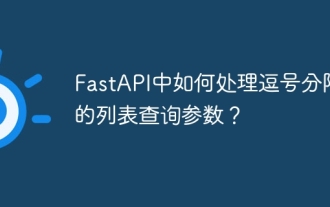
Fastapi ...
