When is a User-Defined Copy Constructor Necessary in C ?
Oct 23, 2024 pm 06:30 PMWhen is a User-Defined Copy Constructor Necessary?
In C , the compiler automatically generates a default copy constructor that performs member-wise copying. However, this default constructor may not always provide the desired behavior. When a copy constructor is required, programmers must write a user-defined version that specifies the specific behavior for copying the object.
Situations necessitating a user-defined copy constructor include:
Deep Copying: When an object has members that are pointers to dynamically allocated memory, the default copy constructor only copies the pointer values, not the actual data. To ensure a copy that contains independent data, a user-defined copy constructor must be implemented to perform deep copying.
Example:
<code class="cpp">class Class { public: Class(const char* str); ~Class(); private: char* stored; }; Class::Class(const char* str) { stored = new char[strlen(str) + 1]; strcpy(stored, str); } Class::~Class() { delete[] stored; }</code>
In this example, the default copy constructor would only copy the pointer stored, resulting in two copies pointing to the same dynamically allocated memory. To ensure safe copying, a user-defined copy constructor that allocates new memory and copies the data is necessary.
Resource Allocation: When the constructor of a class allocates resources that need to be shared or managed explicitly, a user-defined copy constructor can control the allocation and release of these resources. This helps prevent memory leaks and ensures the proper handling of shared resources.
Exception Handling: The copy constructor can be used to handle exceptions that may occur during the copying process. In cases where exceptions are likely to occur during copying, a user-defined copy constructor can explicitly deal with such situations.
Example:
<code class="cpp">class Class { public: Class(const Class& other) try : shared_resource(other.shared_resource) {} catch (...) {} private: std::shared_ptr<Resource> shared_resource; };</code>
In this example, the copy constructor attempts to share the same resource as the original object. If an exception occurs during resource acquisition, the new object is left uninitialized, reducing the risk of incorrect operation.
The above is the detailed content of When is a User-Defined Copy Constructor Necessary in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
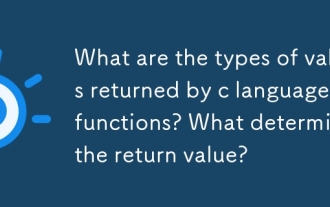
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
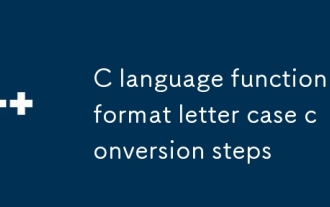
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
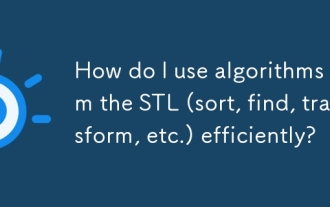
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
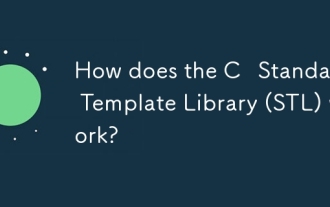
How does the C Standard Template Library (STL) work?
