


How to Create Custom Multipart/Mixed Requests in Go and Resolve Server Parsing Errors?
Multipart Requests in Go: A Comprehensive Guide
In server-client communication, multipart requests play a crucial role in transmitting complex data. These requests combine multiple parts into a single body, each with its own content type and optional metadata. In Go, generating multipart requests requires careful consideration. This article aims to provide a comprehensive solution to creating custom multipart requests.
Issue:
When working with multipart/mixed requests in Go, developers may encounter a common challenge. The server fails to correctly interpret the request body, leading to HTTP errors. This often stems from incorrect multipart generation. The following code demonstrates an example:
<code class="go">var jsonStr = []byte(`{"hello" : "world"}`) func main() { body := &bytes.Buffer{} writer := multipart.NewWriter(body) part, _:= writer.CreateFormField("") part.Write(jsonStr) writer.Close() req, _ := http.NewRequest("POST", "blabla", body) req.Header.Set("Content-Type", writer.FormDataContentType()) ... }</code>
In this scenario, writer.CreateFormField is utilized to create a multipart form field. However, the server expects a multipart/mixed request, resulting in parsing errors.
Solution:
To resolve this issue and successfully generate a multipart/mixed request, the following steps should be followed:
- Initialize a new bytes.Buffer to serve as the request body:
<code class="go">body := &bytes.Buffer{}</code>
- Create a multipart.Writer instance to construct the multipart request:
<code class="go">writer := multipart.NewWriter(body)</code>
- Establish a new part using writer.CreatePart and specify the correct Content-Type header:
<code class="go">part, _ := writer.CreatePart(textproto.MIMEHeader{"Content-Type": {"application/json"}})</code>
- Write the payload to the part:
<code class="go">part.Write(jsonStr)</code>
- Terminate the multipart writer to prepare the body for submission:
<code class="go">writer.Close()</code>
- Create a new HTTP request and populate it with the boundary and request body:
<code class="go">req, _ := http.NewRequest("POST", "http://1.1.1.1/blabla", body) req.Header.Set("Content-Type", "multipart/mixed; boundary="+writer.Boundary())</code>
Implementing these modifications ensures the multipart/mixed request is properly formatted and ready for transmission to the server.
Additional Tips:
For those familiar with cURL, here's how to achieve the same result:
curl -X POST \ --url http://1.1.1.1/blabla \ --header 'Content-Type: multipart/mixed; boundary=--boundary-of-form' \ --form '{"hello": "world"}'
Remember to replace "--boundary-of-form" with an appropriate boundary string.
By incorporating these recommendations into your code, you can effectively generate multipart/mixed requests in Go, ensuring accurate data transmission and seamless integration with target servers.
The above is the detailed content of How to Create Custom Multipart/Mixed Requests in Go and Resolve Server Parsing Errors?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










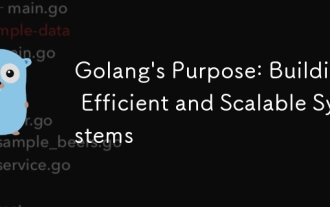
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
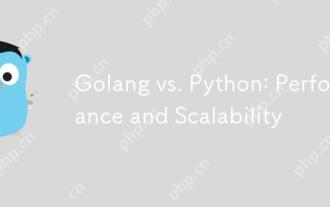
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
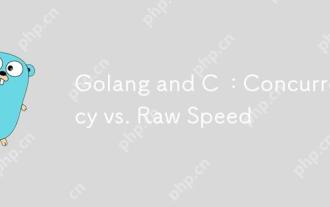
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
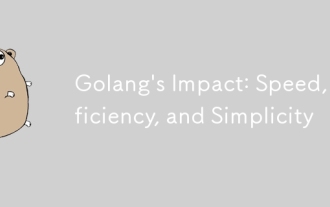
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
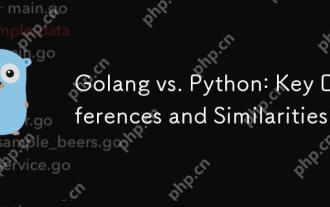
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
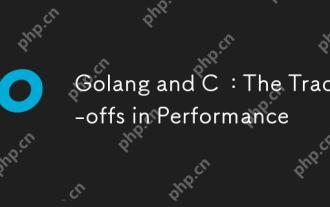
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
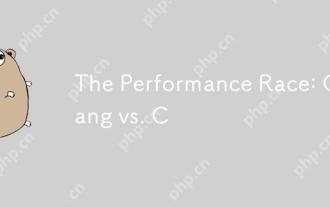
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
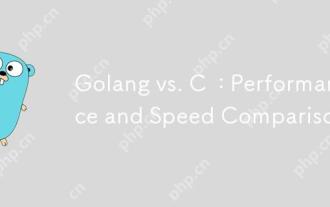
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
