Pure Component in React.js
Keeping components pure is a fundamental principle in React and functional programming. Here’s a deeper exploration of the concept of purity in components, including benefits and strategies for maintaining purity in your React components.
Keeping Components Pure in React
What are Pure Functions?
A pure function is a function that:
- Deterministic: Given the same input, it always produces the same output.
- No Side Effects: It does not cause any side effects, such as modifying external state or interacting with the outside world (e.g., making API calls, manipulating the DOM).
Why Use Pure Components?
Predictability: Pure components behave consistently. You can rely on their outputs, which simplifies reasoning about the application.
Easier Testing: Since pure components are predictable and have no side effects, they are easier to test. You can directly test the output based on the input props without worrying about external state changes.
Performance Optimization: Pure components help optimize rendering. React can efficiently determine if a component needs to re-render based on prop changes.
Maintainability: As your codebase grows, maintaining pure components becomes simpler. They encapsulate functionality without hidden dependencies, making debugging and refactoring easier.
Reuse: Pure components are highly reusable since they don't depend on external states. You can easily use them in different contexts.
How to Keep Components Pure
Here are some strategies to ensure your components remain pure:
-
Avoid Side Effects:
- Do not directly modify props or global state.
- Avoid asynchronous operations inside the render method (e.g., API calls, timers).
const PureComponent = ({ count }) => { // Pure function: does not cause side effects return <div>{count}</div>; };
-
Use React.memo:
- Wrap functional components with React.memo to prevent unnecessary re-renders when props haven’t changed.
const PureGreeting = React.memo(({ name }) => { return <h1>Hello, {name}!</h1>; });
-
Destructure Props:
- Destructure props in the component’s parameter list to keep the component’s structure clean and focused.
const PureButton = ({ label, onClick }) => { return <button onClick={onClick}>{label}</button>; };
-
Lift State Up:
- Manage state in parent components and pass down the required data and event handlers to child components. This keeps child components purely functional.
const ParentComponent = () => { const [count, setCount] = useState(0); return <PureCounter count={count} setCount={setCount} />; };
-
Avoid Inline Functions in Render:
- Instead of defining functions inline in the render method, define them outside. This prevents new function instances from being created on each render, which can lead to unnecessary re-renders.
const PureCounter = React.memo(({ count, setCount }) => { return <button onClick={() => setCount(count + 1)}>Increment</button>; });
-
Avoid Mutating State Directly:
- Use methods that return new states rather than mutating existing state directly. This aligns with immutability principles.
const handleAddItem = (item) => { setItems((prevItems) => [...prevItems, item]); // Pure approach };
Example of a Pure Component
Here’s a complete example of a pure functional component that follows these principles:
import React, { useState } from 'react'; const PureCounter = React.memo(({ count, onIncrement }) => { console.log('PureCounter Rendered'); return <button onClick={onIncrement}>Count: {count}</button>; }); const App = () => { const [count, setCount] = useState(0); const handleIncrement = () => { setCount((prevCount) => prevCount + 1); }; return ( <div> <h1>Pure Component Example</h1> <PureCounter count={count} onIncrement={handleIncrement} /> </div> ); }; export default App;
Conclusion
Keeping components pure in React not only simplifies development but also enhances performance and maintainability. By adhering to the principles of pure functions, you can create components that are predictable, reusable, and easy to test. Following best practices like avoiding side effects, using React.memo, and managing state appropriately can help you build a robust and salable application.
The above is the detailed content of Pure Component in React.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


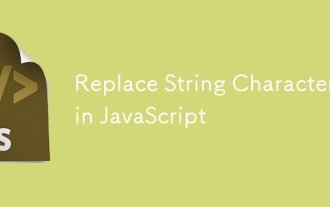
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
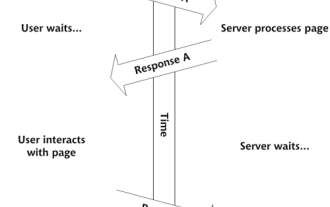
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
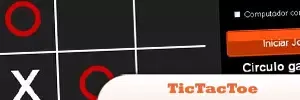
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
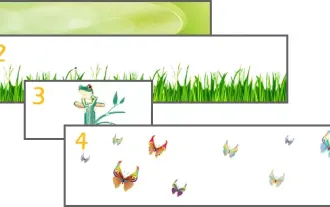
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
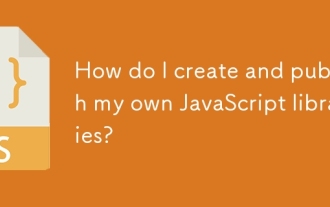
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
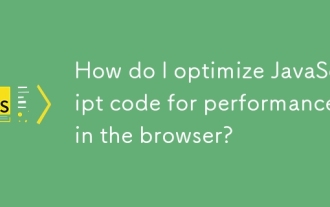
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
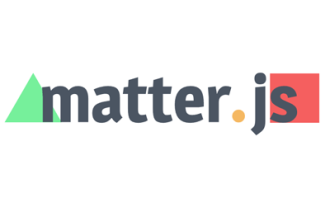
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
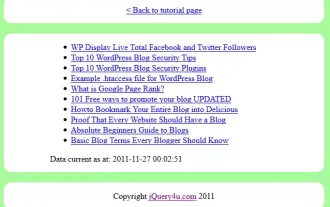
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
