


How to Resolve Syntax Errors in Go for MySQL Queries Using SET Variables?
MySQL Queries with SET Variables in Go
In Go, it's possible to use SET statements before running MySQL queries. However, there are certain syntax requirements that must be met. This article explores an issue encountered when attempting to execute such queries and provides a solution.
The original code snippet involves defining a function that selects records based on a user ID and a sorting parameter. It uses SET statements to assign values to variables before executing the query. However, this approach resulted in a syntax error when run through Go.
The error can be attributed to the way the query is constructed. Specifically, the SET statements need to be encapsulated in parentheses and placed before the SELECT statement. Additionally, the MySQL DSN should include the settings "multiStatements=true" and "interpolateParams=true."
The following updated code snippet resolves the issue:
<code class="go">func (d *DB) SelectByUserId(uid string, srt string, pg, lim int) ([]Inventory, error) { query := ` (SET @user_id := ?, @orderBy := ?;) SELECT * FROM inventory WHERE user_id = @user_id ORDER BY (CASE WHEN @orderBy = 'type,asc' THEN type END), (CASE WHEN @orderBy = 'type,desc' THEN type END) DESC, (CASE WHEN @orderBy = 'visible,asc' THEN visible END), (CASE WHEN @orderBy = 'visible,desc' THEN visible END) DESC, (CASE WHEN @orderBy = 'create_date,asc' THEN create_date END), (CASE WHEN @orderBy = 'create_date,desc' THEN create_date END) DESC, (CASE WHEN @orderBy = 'update_date,asc' THEN update_date END), (CASE WHEN @orderBy = 'update_date,desc' THEN update_date END) DESC LIMIT ?,?; ` dsn := d.dsn + "?multiStatements=true&interpolateParams=true" db, err := sql.Open("mysql", dsn) if err != nil { return nil, err } defer db.Close() rows, err := db.Query( query, uid, srt, pg*lim, lim, ) if err != nil { return nil, err } defer rows.Close() result := make([]Inventory, 0) for rows.Next() { var inv Inventory if err := rows.Scan( &inv.Id, &inv.UserId, &inv.Type, &inv.Name, &inv.Description, &inv.Visible, &inv.CreateDate, &inv.UpdateDate); err != nil { return result, err } result = append(result, inv) } if err = rows.Err(); err != nil { return result, err } return result, nil }</code>
By enclosing the SET statements in parentheses and enabling multi-statements and parameter interpolation in the DSN, the query successfully executes in Go without any syntax errors. Additionally, converting the database and tables to utf8mb4_general_ci collation resolves any potential issues related to character set compatibility.
The above is the detailed content of How to Resolve Syntax Errors in Go for MySQL Queries Using SET Variables?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










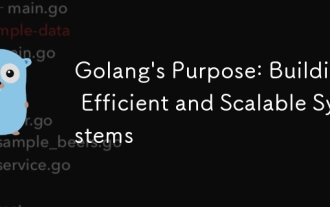
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
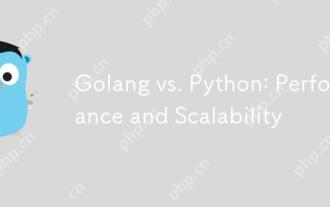
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
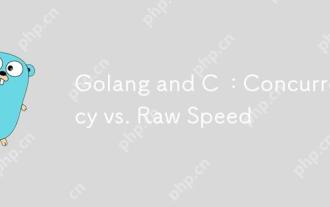
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
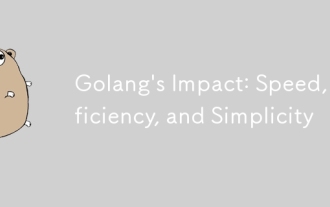
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
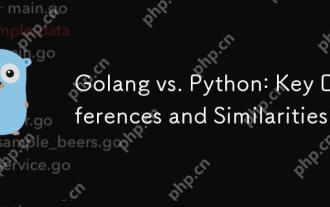
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
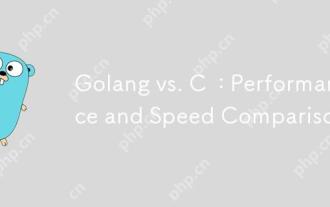
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
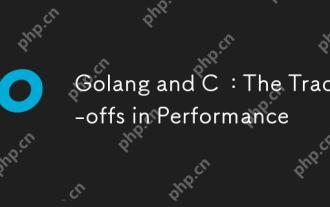
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
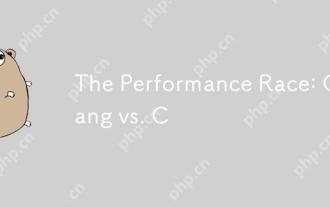
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
