


How to Retrieve All Elements in an XML Array in Golang without Limiting to Just the First Element?
Unmarshal Array Elements in XML: Retrieve All Elements, Not Just the First
When unmarshaling an XML array in Golang using xml.Unmarshal([]byte(p.Val.Inner), &t), you may encounter a situation where only the first element is being retrieved. To resolve this issue, utilize xml.Decoder and repeatedly invoke its Decode method.
Steps to Unmarshal All XML Array Elements:
- Create a new xml.Decoder using xml.NewDecoder(bytes.NewBufferString(VV)), where VV is the XML string containing the array elements.
- Enter a loop to process each XML element:
- Declare a variable t of the target slice type (e.g., HostSystemIdentificationInfo).
- Call d.Decode(&t) to unmarshal the next XML element into the t variable.
- Repeat steps 2-4 until the d.Decode(&t) call returns io.EOF.
Modified Golang Code:
<code class="go">package main import ( "bytes" "encoding/xml" "fmt" "io" "log" ) type HostSystemIdentificationInfo []struct { IdentiferValue string `xml:"identifierValue"` IdentiferType struct { Label string `xml:"label"` Summary string `xml:"summary"` Key string `xml:"key"` } `xml:"identifierType"` } func main() { d := xml.NewDecoder(bytes.NewBufferString(VV)) for { var t HostSystemIdentificationInfo err := d.Decode(&t) if err == io.EOF { break } if err != nil { log.Fatal(err) } fmt.Println(t) } } const VV = `<HostSystemIdentificationInfo xsi:type="HostSystemIdentificationInfo"> <identifierValue> unknown</identifierValue> <identifierType> <label>Asset Tag</label> <summary>Asset tag of the system</summary> <key>AssetTag</key> </identifierType> </HostSystemIdentificationInfo> <HostSystemIdentificationInfo xsi:type="HostSystemIdentificationInfo"> <identifierValue>Dell System</identifierValue> <identifierType> <label>OEM specific string</label> <summary>OEM specific string</summary> <key>OemSpecificString</key> </identifierType> </HostSystemIdentificationInfo> <HostSystemIdentificationInfo xsi:type="HostSystemIdentificationInfo"> <identifierValue>5[0000]</identifierValue> <identifierType> <label>OEM specific string</label> <summary>OEM specific string</summary> <key>OemSpecificString</key> </identifierType> </HostSystemIdentificationInfo> <HostSystemIdentificationInfo xsi:type="HostSystemIdentificationInfo"> <identifierValue>REDACTED</identifierValue> <identifierType> <label>Service tag</label> <summary>Service tag of the system</summary> <key>ServiceTag</key> </identifierType> </HostSystemIdentificationInfo>`</code>
Sample Output:
[{ unknown {Asset Tag Asset tag of the system AssetTag}}] [{Dell System {OEM specific string OEM specific string OemSpecificString}}] [{5[0000] {OEM specific string OEM specific string OemSpecificString}}] [{REDACTED {Service tag Service tag of the system ServiceTag}}]
By using xml.Decoder and repeatedly invoking Decode, you can successfully retrieve all elements within the XML array, resolving the issue of only obtaining the first element.
The above is the detailed content of How to Retrieve All Elements in an XML Array in Golang without Limiting to Just the First Element?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










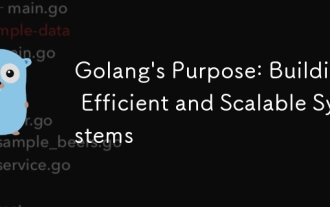
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
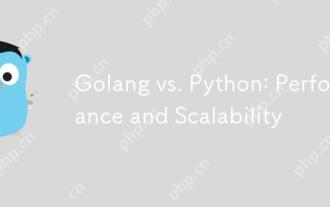
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
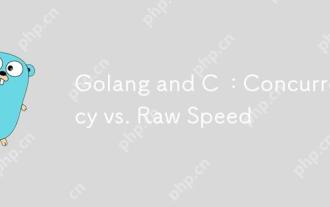
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
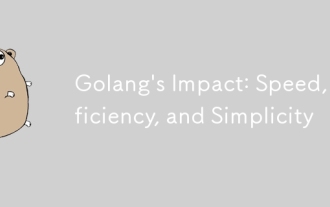
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
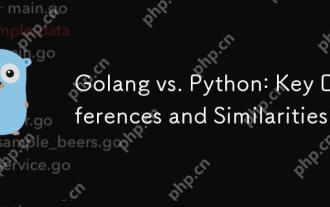
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
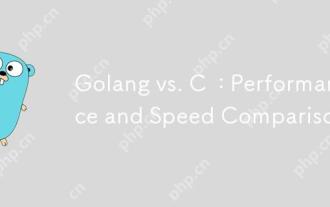
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
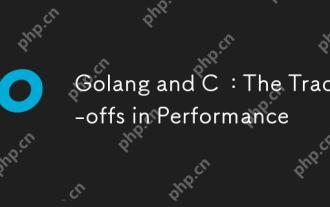
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
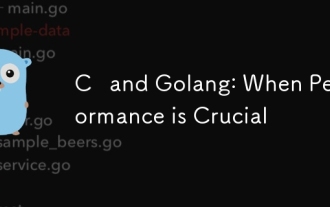
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
