


How to Access Struct Fields in HTML Templates when Using Maps in Go?
Accessing Struct Fields in HTML Templates with Go's html/template
In Go's html/template package, you can encounter challenges when accessing the fields of a struct that is stored as a value in a map. This article provides a solution to this problem, enabling you to retrieve and display individual fields of the struct within your templates.
Consider the following example, where we define a Task struct:
<code class="go">type Task struct { Cmd string Args []string Desc string }</code>
We initialize a map with the Task struct as values and strings as keys:
<code class="go">var taskMap = map[string]Task{ "find": Task{ Cmd: "find", Args: []string{"/tmp/"}, Desc: "find files in /tmp dir", }, "grep": Task{ Cmd: "grep", Args: []string{"foo", "/tmp/*", "-R"}, Desc: "grep files match having foo", }, }</code>
Now, we want to parse an HTML page using the taskMap data using html/template:
<code class="go">func listHandle(w http.ResponseWriter, r *http.Request) { t, _ := template.ParseFiles("index.tmpl") t.Execute(w, taskMap) }</code>
Here's the corresponding template, index.tmpl:
<code class="html"><html> {{range $k, $v := .}} <li>Task Name: {{$k}}</li> <li>Task Value: {{$v}}</li> <li>Task Description: {{$v.Desc}}</li> {{end}} </html></code>
While accessing the $k and $v variables from the map works as expected, accessing the Desc field using {{$v.Desc}} fails. To resolve this, we need to ensure that the fields we want to access in the template are exported. In Go, fields are exported when they start with an uppercase letter.
Solution:
Modify the Task struct to export the Desc field:
<code class="go">type Task struct { Cmd string Args []string Desc string }</code>
Update the map with the exported Desc field:
<code class="go">var taskMap = map[string]Task{ "find": Task{ Cmd: "find", Args: []string{"/tmp/"}, Desc: "find files in /tmp dir", }, "grep": Task{ Cmd: "grep", Args: []string{"foo", "/tmp/*", "-R"}, Desc: "grep files match having foo", }, }</code>
In the template, update the syntax to reference the exported Desc field:
<code class="html">{{range $k, $v := .}} <li>Task Name: {{$k}}</li> <li>Task Value: {{$v}}</li> <li>Task Description: {{$v.Desc}}</li> {{end}}</code>
By following these steps, you will be able to access the struct fields in your HTML templates, allowing you to easily display and utilize the data stored in your Go maps.
The above is the detailed content of How to Access Struct Fields in HTML Templates when Using Maps in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










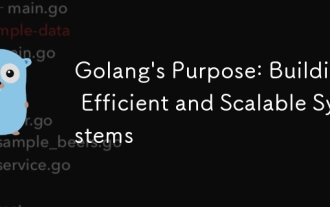
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
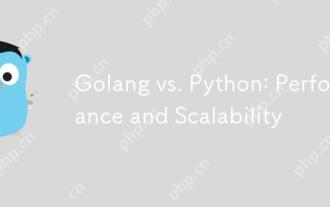
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
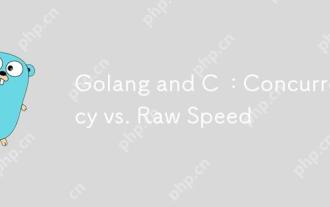
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
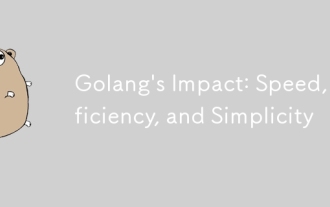
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
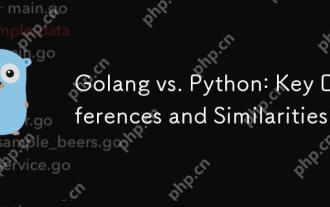
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
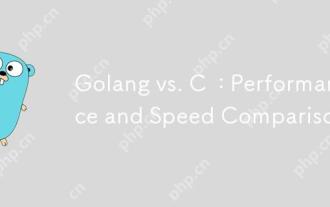
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
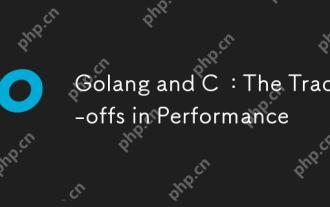
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
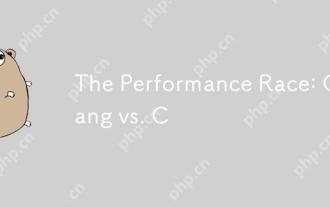
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
