Understanding and Using Javascript Console API in Detail
If you like my articles, you can buy me a coffee :)
The console API is used for debugging, printing messages, and transferring various information to the console, especially in JavaScript runtimes such as browsers and Node.js. But in order to use the console api correctly, you need to know exactly what the console API is.
In this article, I will explain the console API to you. Console API is an object. This object has keys, and when you write a console method, you access the value of a key in the console object.
Now, I will explain to you by coding my own console object so that you can understand the logic of how the log method works in the console API.
const customConsole = { log: function(message) { const timestamp = new Date().toISOString(); const output = `[${timestamp}] LOG: ${message}`; alert(output); // Displaying the output (replace with console.log in a real scenario) } }; customConsole.log("Hello, this is a custom console log!");
Try to read the code. As can be seen in the code, I created a custom console object and defined a key for this object, and the value of this key is a function. Then I accessed the log key of this object.
As a result, the console API doesn't just have a "log" method. So how many are there? Let's find out now.
As seen in the photo, the console object has more than one key and the values of these keys. These values are functions.
We can access these functions with the console object.
console.error() console.warn()
Now let's learn what some of these functions do.
1. console.debug()
console.debug , a function in JavaScript used for debugging purposes in the browser console. By default, output from the console.debug() method will not be visible in Chrome developer tools.
Example :
function subtract(a, b) { console.debug("subtract function called:", { a, b }); const result = a - b; if (result > 0) { console.debug("Result is positive."); } else if (result < 0) { console.debug("Result is negative."); } else { console.debug("Result is zero."); } return result; } let result = subtract(10, 5);
Output :
output from the console.debug() method will not be visible in Chrome developer tools.
2. console.error()
It is a method used in JavaScript to print error messages to the console. It is used to facilitate debugging when an error occurs. the message may receive special formatting, such as red colors and a error icon.
Example :
async function fetchData(ıd) { try { const response = await fetch(`https://jsonplaceholder.typicode.com/posts/${ıd}`); if (!response.ok) { throw new Error(`HTTP error! status: ${response.status}`); } const data = await response.json(); console.log("posts data fetched successfully:", data); return data; } catch (error) { console.error(" error posts data :", error.message); } } fetchData(1);
If there is an error while fetching data, this is the output :
3. console.warn()
It is a method used in JavaScript to print potential problems or situations that require attentionthe console. It is used to facilitate debugging when an error occurs. the message may receive special formatting, such as yellow colors and a warning icon.
Example :
const customConsole = { log: function(message) { const timestamp = new Date().toISOString(); const output = `[${timestamp}] LOG: ${message}`; alert(output); // Displaying the output (replace with console.log in a real scenario) } }; customConsole.log("Hello, this is a custom console log!");
4. console.dir()
The console.dir() method displays a list of the properties of the specified JavaScript object. In browser consoles, the output is presented as a hierarchical listing with disclosure triangles that let you see the contents of child objects.
Example :
console.error() console.warn()
Output :
5. console.dirxml()
The console.dirxml() method displays an interactive tree of the descendant elements of the specified XML/HTML element. If it is not possible to display as an element the JavaScript Object view is shown instead. The output is presented as a hierarchical listing of expandable nodes that let you see the contents of child nodes.
Example :
function subtract(a, b) { console.debug("subtract function called:", { a, b }); const result = a - b; if (result > 0) { console.debug("Result is positive."); } else if (result < 0) { console.debug("Result is negative."); } else { console.debug("Result is zero."); } return result; } let result = subtract(10, 5);
Output :
6. console.assert()
The console.assert() method writes an error message to the console if the assertion is false. If the assertion is true, nothing happens.
Example :
async function fetchData(ıd) { try { const response = await fetch(`https://jsonplaceholder.typicode.com/posts/${ıd}`); if (!response.ok) { throw new Error(`HTTP error! status: ${response.status}`); } const data = await response.json(); console.log("posts data fetched successfully:", data); return data; } catch (error) { console.error(" error posts data :", error.message); } } fetchData(1);
Output :
7. console.count()
The console.count() method logs the number of times that this particular call to count() has been called.
Example :
if (password.length < minLength) { console.warn("Warning: Password must be at least 8 characters long."); return false; }
Output :
Conclusion
If a condition is not true, it prints an error message. If the condition is true, it won't print anything.
The above is the detailed content of Understanding and Using Javascript Console API in Detail. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










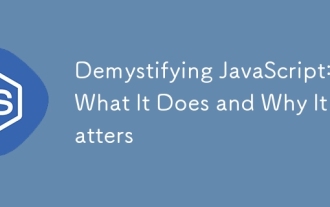
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
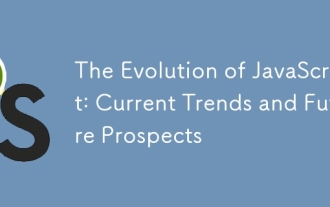
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
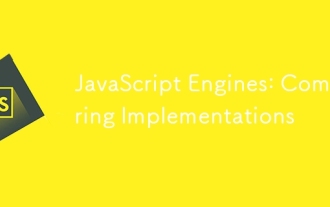
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
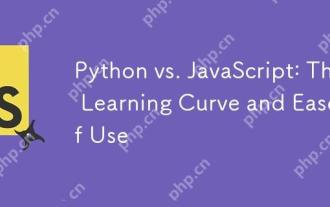
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
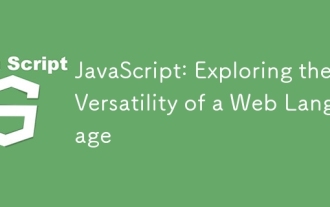
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
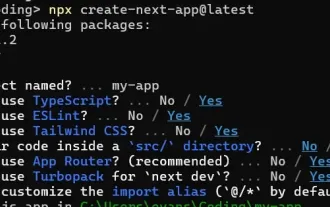
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
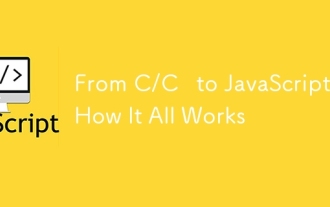
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
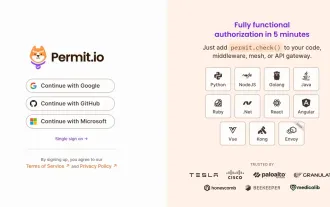
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
