How to Force-Download a CSV File Through AJAX in PHP?
Downloading Files with PHP and AJAX
Question:
How can I force-download a CSV file through an AJAX call in PHP?
Background:
You have created an AJAX function that generates a CSV file based on user input. After creation, you want to automatically download the file without prompting the user.
PHP Code:
In your PHP file (csv.php), you have attempted to use the following script to force download the CSV file:
<code class="php">$fileName = 'file.csv'; $downloadFileName = 'newfile.csv'; if (file_exists($fileName)) { header('Content-Description: File Transfer'); header('Content-Type: text/csv'); header('Content-Disposition: attachment; filename='.$downloadFileName); ob_clean(); flush(); readfile($fileName); exit; } echo "done";</code>
Issue:
When you run this script at the end of csv.php, the contents of the file are displayed on the page instead of being downloaded.
Solution:
AJAX cannot be used for directly downloading files. To force download the file, you can use one of the following methods:
- Pop up new window: Create a new window with the download link as its address.
- document.location = ...: Use the document.location = ... property to set the current location of the document to the download link.
Example:
You can modify your AJAX function as follows to pop up a new window with the download link:
<code class="javascript">function csv() { ajaxRequest = ajax(); postdata = "data=" + document.getElementById("id").value; ajaxRequest.onreadystatechange = function () { var ajaxDisplay = document.getElementById('ajaxDiv'); if (ajaxRequest.readyState == 4 && ajaxRequest.status == 200) { window.open(ajaxRequest.responseText); // Pop up a new window with the download link } } ajaxRequest.open("POST", "csv.php", false); ajaxRequest.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); ajaxRequest.send(postdata); }</code>
The above is the detailed content of How to Force-Download a CSV File Through AJAX in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
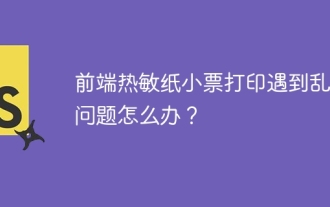
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
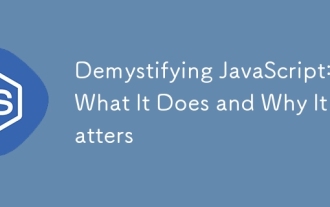
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
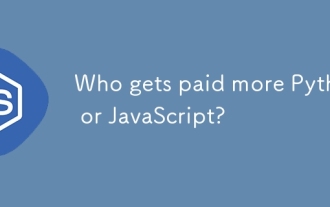
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
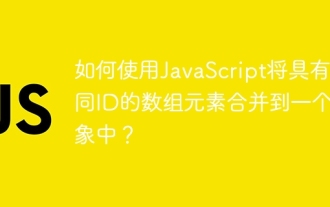
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
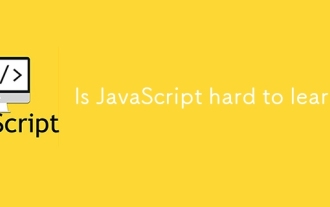
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
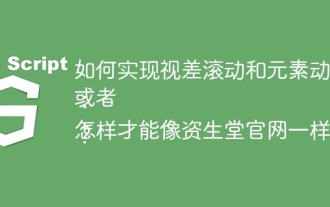
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
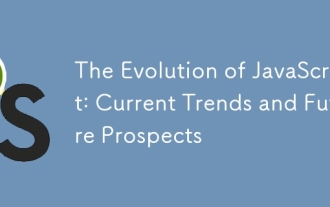
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
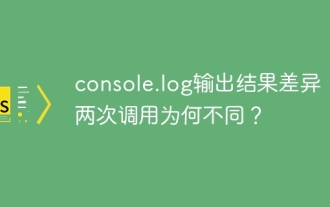
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
