How to Set Embedded Struct Fields with Reflection in Go?
Setting Fields of Embedded Structs with Reflection: Unraveling the Mysteries
In Go, the ability to dynamically manipulate data structures using reflection is a powerful tool. However, it can present challenges when dealing with embedded structs. This article explores a common issue that arises when attempting to set field values in such structs using reflection.
The Problem:
You encounter a situation where you desire to modify the Field1 field within an Entry struct that is embedded within a ProductionInfo struct. However, despite calling reflect.CanSet(), it consistently returns false, indicating that the field cannot be set.
The Solution:
Delving into the provided code, we identify several key issues that need to be addressed:
- Target the Correct Struct: The SetField function initially operates on the ProductionInfo struct, whereas the intended target is the Entry struct. To correct this, the call should specify the Entry struct instead: SetField(source.StructA[0], "Field1", "NEW_VALUE").
- Pass a Pointer to the Struct: Reflection requires pointers to structs in order to modify their fields. Therefore, it is necessary to pass a pointer to the Entry struct: SetField(&source.StructA[0], "Field1", "NEW_VALUE").
- Obtain the Value of the Pointed Struct: Once you have a pointer to the struct, you can use reflect.ValueOf(source).Elem() to navigate to the reflect.Value of the pointed struct (the struct value).
- Correctly Access the Field: Once you have the value of the struct, you can access the field by name. In this case, you should use v.FieldByName(fieldName) instead of v.Field(k).
By implementing these changes, you enable the SetField function to successfully set the field value using reflection. Here is the modified code:
<code class="go">func SetField(source interface{}, fieldName string, fieldValue string) { v := reflect.ValueOf(source).Elem() fmt.Println(v.FieldByName(fieldName).CanSet()) if v.FieldByName(fieldName).CanSet() { v.FieldByName(fieldName).SetString(fieldValue) } } func main() { source := ProductionInfo{} source.StructA = append(source.StructA, Entry{Field1: "A", Field2: 2}) fmt.Println("Before: ", source.StructA[0]) SetField(&source.StructA[0], "Field1", "NEW_VALUE") fmt.Println("After: ", source.StructA[0]) }</code>
By following these steps, you can effectively set field values in embedded structs using reflection, providing you with greater flexibility in manipulating complex data structures.
The above is the detailed content of How to Set Embedded Struct Fields with Reflection in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


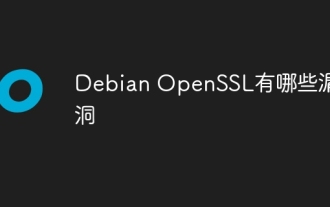
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
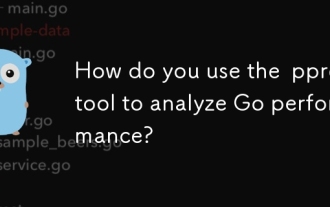
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
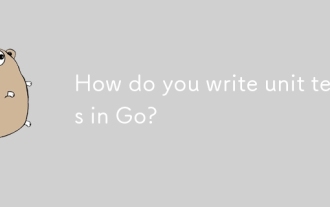
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
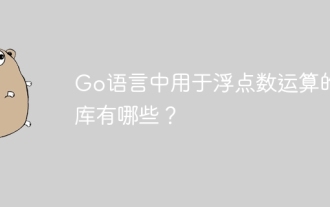
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
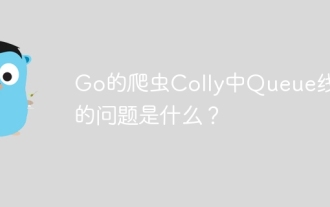
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
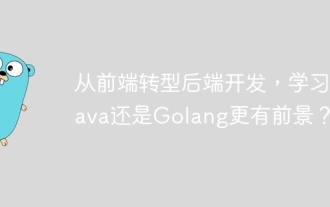
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
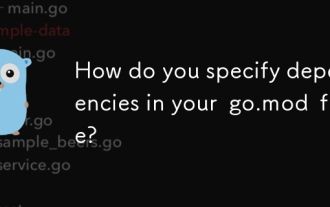
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
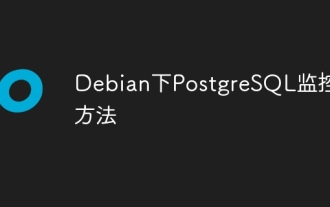
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
