How to Read a File in Reverse Order (From End to Start) in Java?
How to Read File from End to Start (in Reverse Order) in Java?
Reading a file in reverse order, from the end to the start, can be a useful operation in various situations. Here's a breakdown of how it can be achieved using a BufferReader:
The Problem:
You have a file and want to read it in a reverse line order.
The Solution:
To accomplish this task, we can utilize the ReverseLineInputStream class, which allows us to read lines from a file in the reverse order without loading the entire file into memory. The advantage of this method is that it can handle large files efficiently.
The ReverseLineInputStream class maintains pointers to the file and uses the standard InputStream methods. Its core functionality involves:
-
FindPrevLine Method:
- Determines the starting point of the previous line by identifying the last newline character (LF).
- Adjusts the currentLineStart and currentLineEnd pointers accordingly.
-
Read Method:
- Checks if there are more characters to read in the current line.
- Advances the file pointer and returns the character.
- If there are no more characters in the current line, it calls findPrevLine to move to the previous line and continues reading from there.
- Returns -1 when there are no more lines to read.
Here's an example of how to use the ReverseLineInputStream:
<code class="java">File file = new File("/var/nagios.log"); ReverseLineInputStream rlis = new ReverseLineInputStream(file); BufferedReader br = new BufferedReader(new InputStreamReader(rlis)); while (true) { String line = br.readLine(); if (line == null) { break; } System.out.println(line); }</code>
This code will read the contents of the /var/nagios.log file in reverse order, line by line.
The above is the detailed content of How to Read a File in Reverse Order (From End to Start) in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










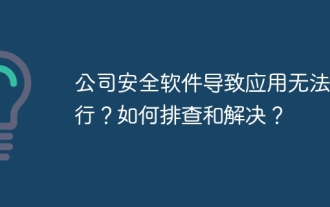
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
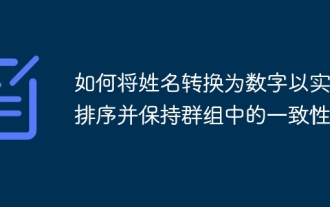
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
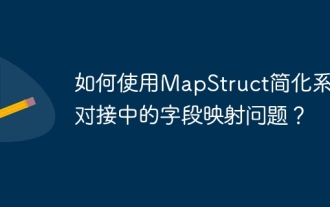
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
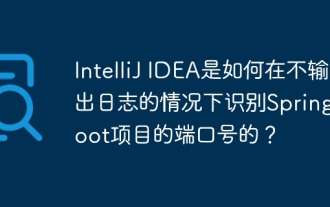
Start Spring using IntelliJIDEAUltimate version...
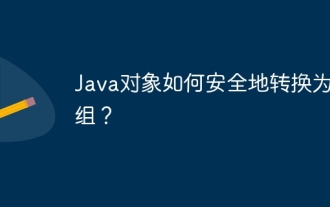
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
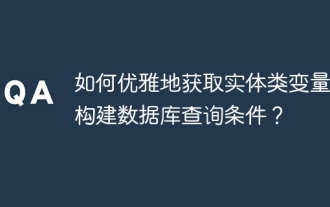
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
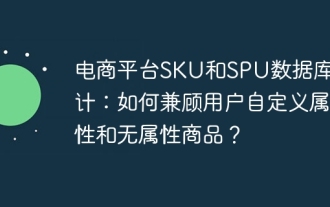
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
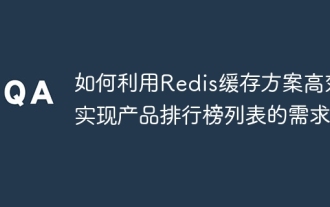
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
