How to Import Rows to PostgreSQL from STDIN in Go?
Importing Rows to PostgreSQL from STDIN
In Python, the subprocess module provides a convenient way to write data to PostgreSQL from STDIN using the COPY command. However, achieving this same functionality in Go involves a different approach.
The official documentation for the github.com/lib/pq package offers an example of how to import records directly from STDIN using the CopyIn function. Here's an adapted version of the provided code:
<code class="go">package main import ( "database/sql" "fmt" "log" "github.com/lib/pq" ) func main() { records := [][]string{ {"Rob", "Pike"}, {"Ken", "Thompson"}, {"Robert", "Griesemer"}, } db, err := sql.Open("postgres", "dbname=postgres user=postgres password=postgres") if err != nil { log.Fatalf("open: %v", err) } if err = db.Ping(); err != nil { log.Fatalf("open ping: %v", err) } defer db.Close() txn, err := db.Begin() if err != nil { log.Fatalf("begin: %v", err) } stmt, err := txn.Prepare(pq.CopyIn("test", "first_name", "last_name")) if err != nil { log.Fatalf("prepare: %v", err) } for _, r := range records { _, err = stmt.Exec(r[0], r[1]) if err != nil { log.Fatalf("exec: %v", err) } } _, err = stmt.Exec() if err != nil { log.Fatalf("exec: %v", err) } err = stmt.Close() if err != nil { log.Fatalf("stmt close: %v", err) } err = txn.Commit() if err != nil { log.Fatalf("commit: %v", err) } log.Println("Records successfully imported") }</code>
This revised code uses a transaction to ensure atomicity, consistency, isolation, and durability (ACID) properties during the import process. The CopyIn function allows you to specify the target table name and the columns to which the data will be inserted.
The provided sample data is then inserted into the "test" table using prepared statements. Prepared statements enhance performance by precompiling the SQL query, reducing the overhead associated with parsing and planning.
Once all records are inserted, the transaction is committed and the data is permanently stored in the database.
The above is the detailed content of How to Import Rows to PostgreSQL from STDIN in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
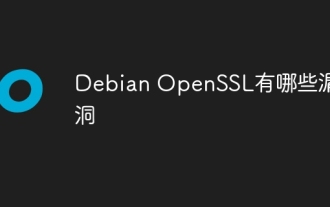
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
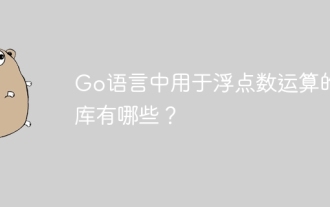
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
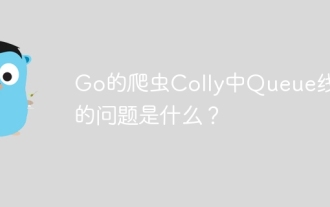
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
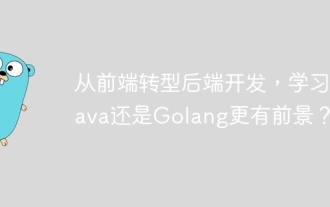
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
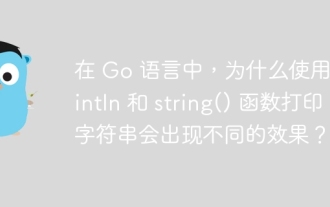
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
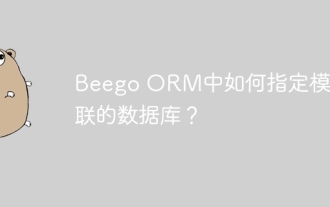
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
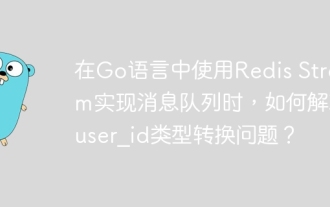
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
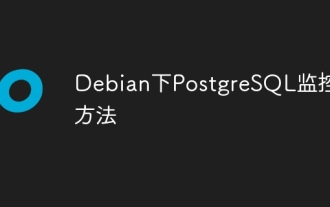
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
