How to Import Rows into PostgreSQL from STDIN Using Go?
Importing Rows into PostgreSQL from STDIN Using Go
In Go, you can import rows into PostgreSQL from standard input (STDIN) using the pq package. This approach directly feeds the data into the database without the need for intermediate files.
To achieve direct row importing from STDIN, follow these steps:
- Open a PostgreSQL Connection: Establish a connection to your PostgreSQL database using the sql.Open function.
- Begin a Transaction: Initiate a database transaction using the Begin function to group multiple statements together.
- Prepare Copy Statement: Create a prepared statement for copying data using pq.CopyIn. This statement specifies the target table name and the columns to import.
- Import Rows: Execute the prepared statement iteratively for each row of data you want to import. Use the Exec function to send each row to the database.
- Terminate Copy Statement: After adding all the rows, execute the prepared statement again without any arguments to finalize the import process.
- Close Prepared Statement: Release resources associated with the prepared statement by calling the Close method.
- Commit Transaction: Commit the transaction to make the changes permanent in the database.
Example Code:
Here's a code example that demonstrates row importing from STDIN using Go:
<code class="go">package main import ( "database/sql" "fmt" "io" "log" "github.com/lib/pq" ) func main() { db, err := sql.Open("postgres", "host=localhost port=5432 user=postgres password=mysecret dbname=mydatabase") if err != nil { log.Fatal(err) } defer db.Close() rows := [][]string{ {"Rob", "Pike"}, {"Ken", "Thompson"}, {"Robert", "Griesemer"}, } txn, err := db.Begin() if err != nil { log.Fatal(err) } stmt, err := txn.Prepare(pq.CopyIn("test", "first_name", "last_name")) if err != nil { log.Fatal(err) } for _, r := range rows { if _, err = stmt.Exec(r[0], r[1]); err != nil { log.Fatal(err) } } if _, err = stmt.Exec(); err != nil { log.Fatal(err) } if err = stmt.Close(); err != nil { log.Fatal(err) } if err = txn.Commit(); err != nil { log.Fatal(err) } fmt.Println("Rows imported successfully.") }</code>
By following these steps and utilizing the pq package, you can efficiently import data into PostgreSQL directly from STDIN in your Go programs.
The above is the detailed content of How to Import Rows into PostgreSQL from STDIN Using Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
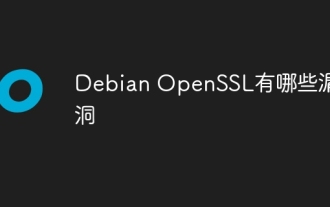
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
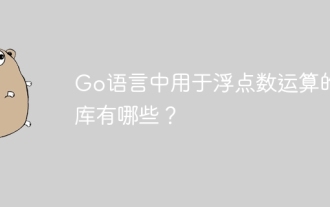
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
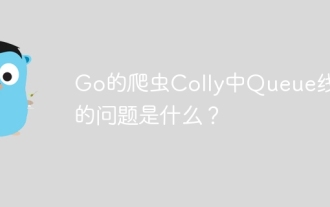
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
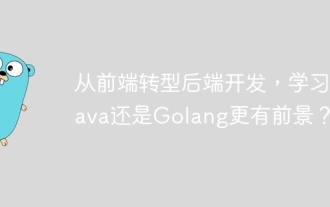
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
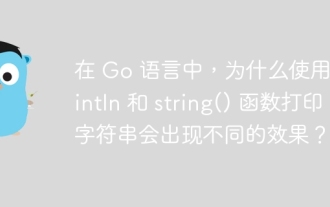
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
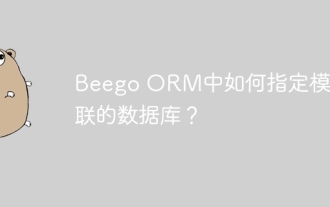
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
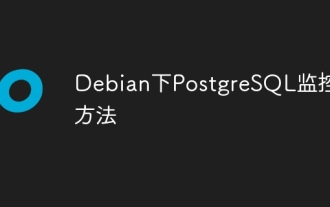
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
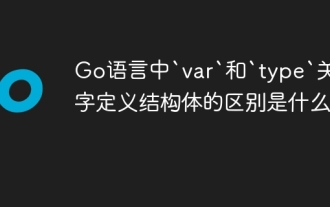
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
