Next.js : Incremental Static Regeneration (ISR)
With Incremental Static Regeneration (ISR)
We can update static content without recreating the entire site. We can reduce the server load by providing pre-created static pages for most requests.We can process a large number of content pages before the next creation times are long.
Let's start with an example :
export const revalidate = 30 export const dynamicParams = true // or false, to 404 on unknown paths export async function generateStaticParams() { const posts = await fetch('https://jsonplaceholder.typicode.com/posts/').then((res) => res.json() ) return posts.map((post) => ({ id: String(post.id), })) } export default async function Page({ params }) { const post = await fetch(`https://jsonplaceholder.typicode.com/posts/${params.id}`).then( (res) => res.json() ) return ( <main> <h1>{post.title}</h1> <p>{post.content}</p> </main> ) }
How this example works ?
All requests made to these pages (e.g. /post/1) are cached and instantaneous. After 30 seconds has passed, the next request will still show the cached (stale) page. The cache is invalidated and a new version of the page begins generating in the background. Once generated successfully, Next.js will display and cache the updated page If /post/12 is requested, Next.js will generate and cache this page on-demand.
If you need more precision, on-demand revalidation can be used, but if you need real-time data, consider switching to dynamic processing.
On-demand Revalidation with revalidatePath
Next.js allows you to manually clear the cache of a specific page or route . That is, when a certain content is updated, instead of recreating all the pages, you can only recreate the changed page or content.
You want the page to be updated immediately when there is a change (for example, when a new post is added), you can trigger this process by using the revalidatePath function. In other words, you can make the new content appear as soon as a new content is added to the user.
'use server' import { revalidatePath } from 'next/cache' export async function createPost() { // Invalidate the /posts route in the cache revalidatePath('/posts') }
On-demand Revalidation with revalidateTag
Next.js applications, it allows you to manually clear their cache by tagging certain data or content. This is used to clear the entire cache of that content when a certain content changes. When the content is updated, users see the latest data.
export default async function Page() { const data = await fetch('https://api.vercel.app/blog', { next: { tags: ['posts'] }, }) const posts = await data.json() // ... }
You can then use revalidateTag :
'use server' import { revalidateTag } from 'next/cache' export async function createPost() { // Invalidate all data tagged with 'posts' in the cache revalidateTag('posts') }
ISR is not supported when creating a Static Export. If you have multiple fetch requests in a statically rendered route, and each has a different revalidate frequency, the lowest time will be used for ISR. However, those revalidate frequencies will still be respected by the Data Cache.If any of the fetch requests used on a route have a revalidate' time of 0, or an explicit no-store, the route will be dynamically rendered. Middleware won't be executed for on-demand USER requests, meaning any path rewrites or logic in Middleware will not be applied. Ensure you are revalidating the exact path. For example, /post/1 instead of a rewritten /post-1.
If you like my articles, you can buy me a coffee :)
The above is the detailed content of Next.js : Incremental Static Regeneration (ISR). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










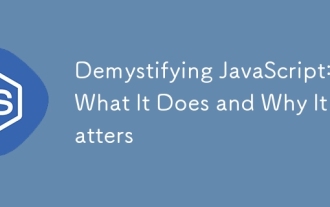
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
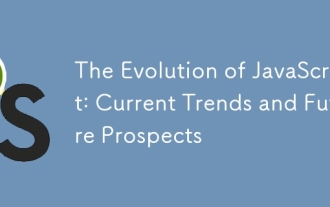
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
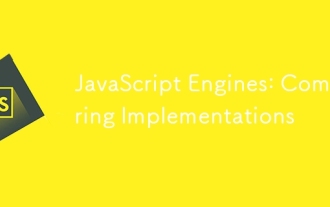
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
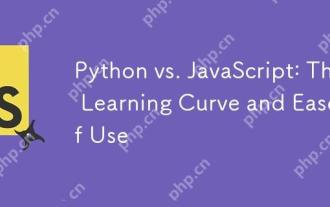
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
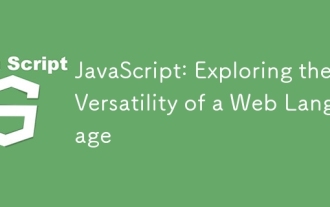
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
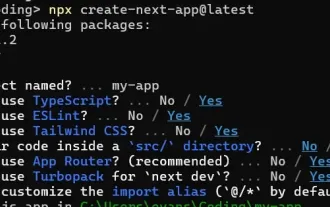
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
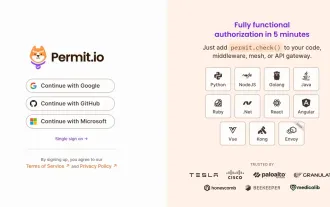
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
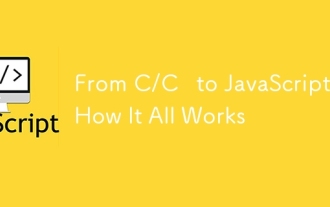
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
