


How to Use the Kubernetes Go Client to Retrieve Detailed Pod Information Like \'kubectl get pods\'?
Kubernetes go-client: Fetching Pod Details Like 'kubectl get pods'
To obtain pod details from a Kubernetes cluster using the Kubernetes client-go, follow these steps:
-
Create a Pod Interface: Use the client-go's PodInterface to manage pods in a specific namespace.
<code class="go">podInterface := client.KubeClient.CoreV1().Pods(namespace)</code>
Copy after login -
List Pods: Retrieve all pods in the namespace.
<code class="go">podList, err := podInterface.List(context.TODO(), v1.ListOptions{})</code>
Copy after login -
Iterate Over Pods: Iterate through the retrieved pod list to extract specific details like name, status, ready state, restarts, and age.
<code class="go">for _, pod := range podList.Items { // Calculate pod age age := time.Since(pod.GetCreationTimestamp().Time).Round(time.Second) // Get pod status podStatus := pod.Status // Accumulate container stats var containerRestarts, containerReady, totalContainers int32 for range pod.Spec.Containers { // Add restart count from container status containerRestarts += podStatus.ContainerStatuses[container].RestartCount // Calculate number of ready containers if podStatus.ContainerStatuses[container].Ready { containerReady++ } totalContainers++ } }</code>
Copy after login
This approach effectively generates a table similar to the output of 'kubectl get pods -n
The above is the detailed content of How to Use the Kubernetes Go Client to Retrieve Detailed Pod Information Like \'kubectl get pods\'?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










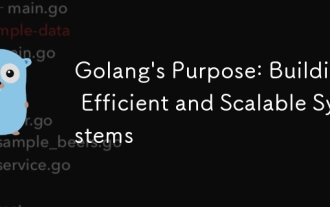
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
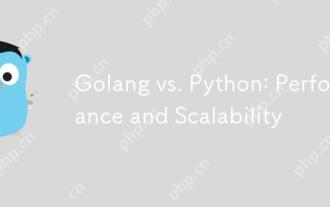
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
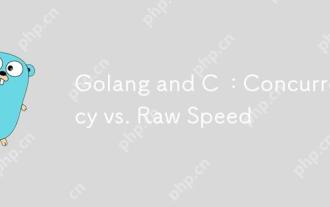
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
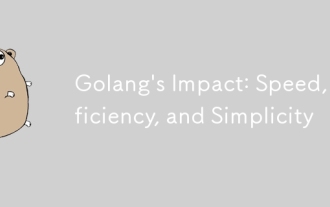
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
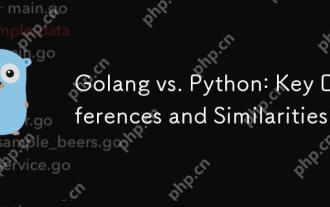
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
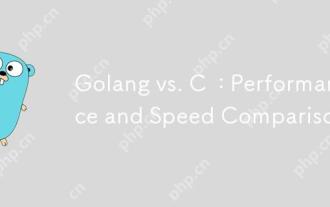
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
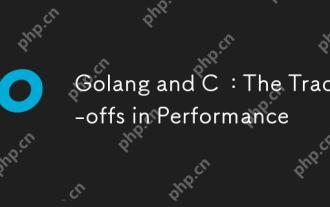
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
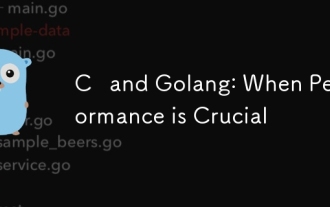
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
