


How to Parse an Arithmetic Expression and Construct a Tree Representation in Java?
Parsing an Arithmetic Expression and Building a Tree from It in Java
In this article, we'll delve into the intricacies of parsing an arithmetic expression and constructing a tree representation of it in Java.
Introduction
To begin with, we'll assume that the input expression is supplied as a string and complies with the following rules:
- Negative numbers must be enclosed in parentheses.
- Parentheses must balance consistently.
Building the Tree
At its core, constructing a tree from an arithmetic expression involves utilizing a stack. As we parse the expression character by character, we push operators and numerical values onto the stack. Operators have an associated precedence, allowing us to evaluate and combine sub-expressions as we encounter them.
Approach
- Initialize the Stack: Start with an empty stack.
- Parse the Expression: Visit each character in the expression string.
- Handle Parentheses: If a parenthesis is encountered, either push it onto the stack or evaluate the sub-expression within parentheses.
- Handle Operators: On encountering an operator, compare its precedence to the current "highest precedence" in the stack. If current is lower than the new operator's precedence, push the new operator onto the stack. Otherwise, evaluate the operators on the stack until the new operator's precedence is higher or equal.
- Handle Numerical Values: Push numerical values directly onto the stack.
- Evaluate the Stack: Once all characters are processed, evaluate the remaining operators on the stack from top to bottom.
Example
Consider the expression:
(5+2)*7
We would parse it as follows:
Character | Action | Stack |
---|---|---|
( | Push ( | ( |
5 | Push 5 | (, 5 |
Push | (, 5, | |
2 | Push 2 | (, 5, , 2 |
) | Evaluate to 7, push 7 | (, 7 |
* | Push * | (, 7, * |
7 | Push 7 | 7, *, 7 |
The resulting tree would be:
(5+2)*7
Conclusion
Parsing an arithmetic expression and building a tree is a fundamental operation in computer science. This article provided a step-by-step approach using a stack, highlighting the importance of precedence rules and parenthetical balance. Implementing this algorithm in Java will enable you to create powerful applications that can process and manipulate arithmetic expressions effectively.
The above is the detailed content of How to Parse an Arithmetic Expression and Construct a Tree Representation in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










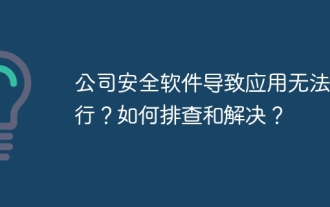
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
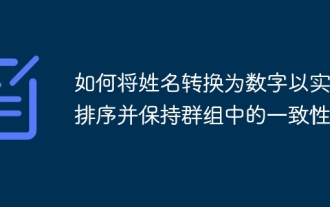
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
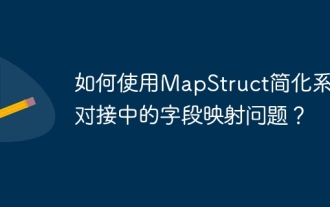
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
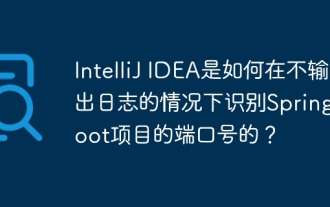
Start Spring using IntelliJIDEAUltimate version...
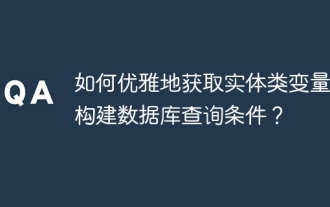
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
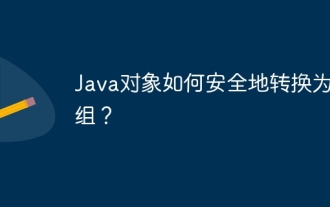
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
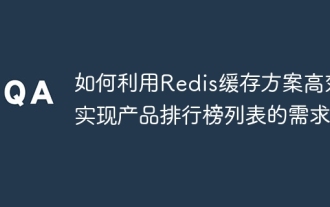
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
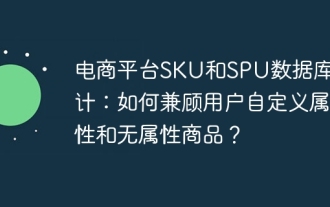
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
