


How to Generate Accurate CSS Paths from DOM Elements: Why :nth-child Matters?
How to Generate Accurate CSS Paths from DOM Elements
You've provided a function that retrieves CSS paths from DOM elements. While it effectively captures the element's hierarchy, it lacks the precision required for unique identification.
The Problem
The CSS path for the 123rd anchor element your function returns is:
html > body > div#div-id > div.site > div.clearfix > ul.choices > li
However, to fully identify the element, it should include the :nth-child selector:
html > body > div#div-id > div.site:nth-child(1) > div.clearfix > ul.choices > li:nth-child(5)
The Solution
To derive accurate CSS paths, implement the following enhancements in your function:
<code class="javascript">var cssPath = function(el) { if (!(el instanceof Element)) return; var path = []; while (el.nodeType === Node.ELEMENT_NODE) { var selector = el.nodeName.toLowerCase(); if (el.id) { selector += '#' + el.id; path.unshift(selector); break; } else { var sib = el, nth = 1; while (sib = sib.previousElementSibling) { if (sib.nodeName.toLowerCase() == selector) nth++; } if (nth != 1) selector += ":nth-of-type("+nth+")"; } path.unshift(selector); el = el.parentNode; } return path.join(" > "); };</code>
This improved function:
- Handles non-element nodes: Prevents premature loop termination when encountering non-element nodes.
- Prioritizes ID selectors: Identifies ancestors with IDs to improve path efficiency.
- Utilizes nth-of-type: Enhances readability and uniqueness of selectors.
By incorporating these modifications, you can confidently generate accurate CSS paths that accurately represent the hierarchy and position of DOM elements.
The above is the detailed content of How to Generate Accurate CSS Paths from DOM Elements: Why :nth-child Matters?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


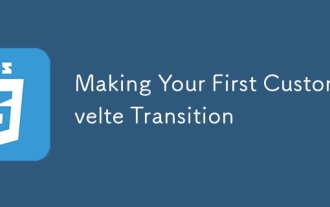
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
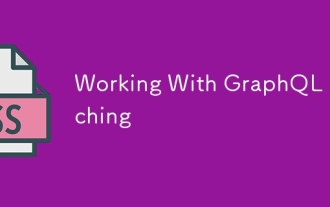
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
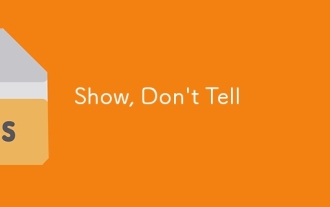
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
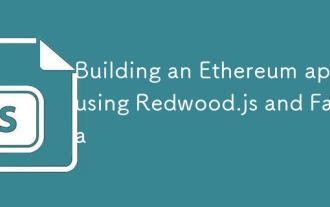
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
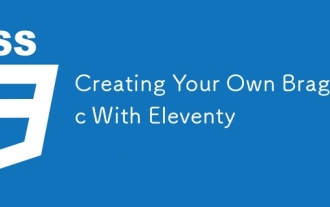
No matter what stage you’re at as a developer, the tasks we complete—whether big or small—make a huge impact in our personal and professional growth.
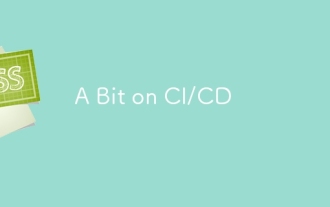
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
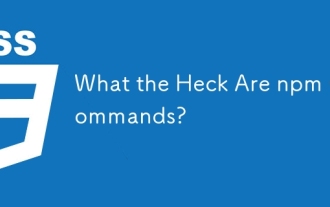
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
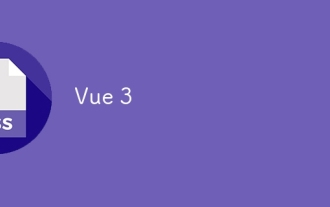
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
