


How can I use Boost.Program_options to find the line number of a specific entry in an INI file?
Cross-platform way to get line number of an INI file where given option was found
Many applications today use .ini files to store configuration data. These files are simple and easy to parse. However, finding the line number of a specific entry can be difficult without the help of a library.
Boost.Program_options is a C library that provides a convenient way to parse and store command-line options. It also has support for parsing .ini configuration files.
To use Boost.Program_options to find the line number of an .ini entry, you can use the following steps:
- Create a boost::program_options::options_description object to describe the options in your .ini file.
- Create a boost::program_options::variables_map object to store the values of the options.
- Use the boost::program_options::store() function to parse the .ini file and store the values in the variables_map object.
- Use the boost::program_options::notify() function to validate the values in the variables_map object.
If any of the values in the variables_map object are invalid, the notify() function will throw an exception. This exception will contain the line number of the invalid value.
Here is an example of how to use Boost.Program_options to find the line number of an .ini entry:
<code class="cpp">#include <boost/program_options.hpp> #include <iostream> int main(int argc, char** argv) { try { // Create an options description object. boost::program_options::options_description options; options.add_options() ("my-option", boost::program_options::value<std::string>(), "My option"); // Create a variables map object. boost::program_options::variables_map vm; // Parse the INI file. boost::program_options::store(boost::program_options::parse_config_file<char>("my.ini", options), vm); // Validate the values. boost::program_options::notify(vm); // Find the line number of the "my-option" option. if (vm.count("my-option")) { std::cout << "The line number of the \"my-option\" option is: " << vm["my-option"].source() << std::endl; } else { std::cout << "The \"my-option\" option was not found." << std::endl; } } catch (std::exception& e) { std::cerr << "Error: " << e.what() << std::endl; return 1; } return 0; }</code>
This code will parse the my.ini file and store the values in the variables_map object. It will then validate the values and find the line number of the "my-option" option. If the option is not found, the code will print an error message.
The above is the detailed content of How can I use Boost.Program_options to find the line number of a specific entry in an INI file?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


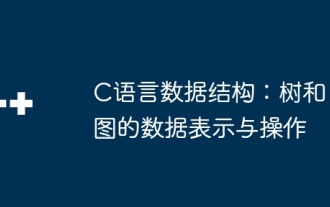
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
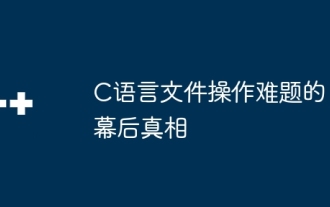
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
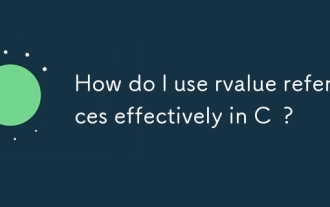
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
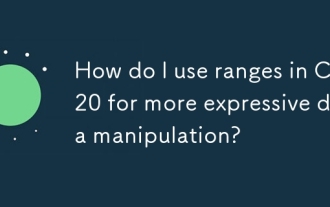
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
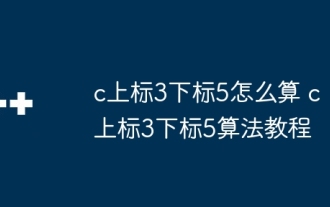
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
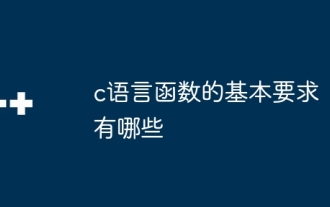
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
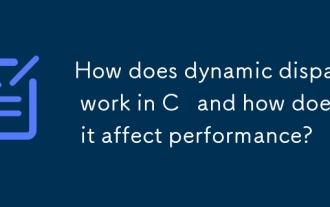
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
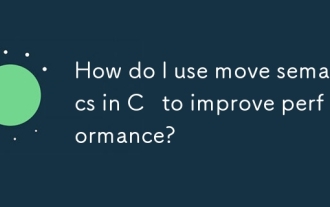
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
