React Basics~unit test/async test
When I test an async action, I use async/await in the test code.
I need to prepare for test data. In this case I use a json server.
・mock/db.json
{ "users": [ { "id": 1, "name": "Foo" } ] }
・package.json
"scripts": { "dev": "vite", "start": "vite", "build": "vite build", "test": "vitest", "preview": "vite preview", // ↓ setting a script for json server "json-server": "npx json-server -w ./mock/db.json -p 4030" },
And then I have to run a command.
npm run json-server
・src/Example.js
import GetUserData from "./components/GetUserData"; //The path of test data export const ENDPOINT_URL = 'http://localhost:4030/users/1'; const Example = () => { return ( <> <GetUserData url={ENDPOINT_URL}/> </> ); }; export default Example;
・src/components/GetUserData.jsx
import { useEffect, useState } from "react"; import axios from "axios"; const GetUserData = ({ url }) => { const [userData, setUserData] = useState(null); useEffect(() => { axios.get(url).then((response) => setUserData(response.data)); // eslint-disable-next-line react-hooks/exhaustive-deps }, []); return ( <div> {userData ? ( <> <h2>Profile</h2> <ul> <li>ID: {userData.id}</li> <li>Name: {userData.name}</li> </ul> </> ) : ( <h1>...loading</h1> )} </div> ); }; export default GetUserData;
・src/components/GetUserData.test.jsx
import { render, screen } from "@testing-library/react"; import GetUserData from "./GetUserData"; import { ENDPOINT_URL } from "../Example"; describe("Check an action of The GetUserData component", () => { test("External data fetching in progress", () => { render(<GetUserData url={ENDPOINT_URL} />); const h1El = screen.getByRole("heading", { name: "...loading" }); expect(h1El).toBeInTheDocument(); }); ★ Not using async/await test("After external data fetching", () => { render(<GetUserData url={ENDPOINT_URL} />); const h2El = screen.findByRole("heading", { name: "Profile" }); expect(h2El).toBeInTheDocument(); const itemEls = screen.findAllByRole("listitem"); expect(itemEls[0].textContent).toBe("ID: 1"); expect(itemEls[1].textContent).toBe("Name: Foo"); }); });
- If I don't use async/await, the test fails at expect(h2El).toBeInTheDocument();.
Because the test continues without user data.
・src/components/GetUserData.test.jsx
import { render, screen } from "@testing-library/react"; import GetUserData from "./GetUserData"; import { ENDPOINT_URL } from "../Example"; describe("Check an action of The GetUserData component", () => { test("External data fetching in progress", () => { render(<GetUserData url={ENDPOINT_URL} />); const h1El = screen.getByRole("heading", { name: "...loading" }); expect(h1El).toBeInTheDocument(); }); ★ Using async/await test("After external data fetching", async () => { render(<GetUserData url={ENDPOINT_URL} />); const h2El = await screen.findByRole("heading", { name: "Profile" }); expect(h2El).toBeInTheDocument(); const itemEls = await screen.findAllByRole("listitem"); expect(itemEls[0].textContent).toBe("ID: 1"); expect(itemEls[1].textContent).toBe("Name: Foo"); }); });
- If I use async/await, the test succeeds.
- Display
The above is the detailed content of React Basics~unit test/async test. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


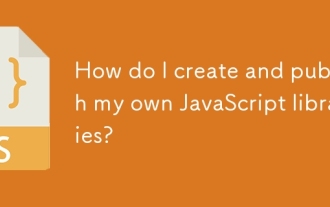
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
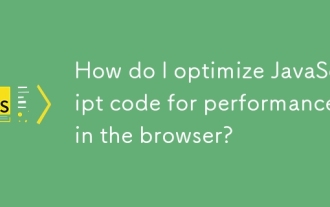
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
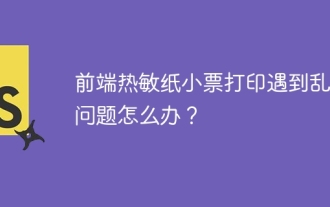
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
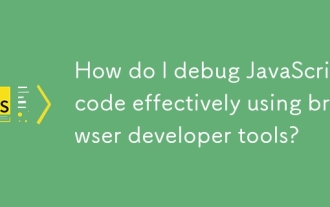
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
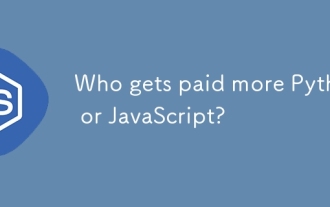
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
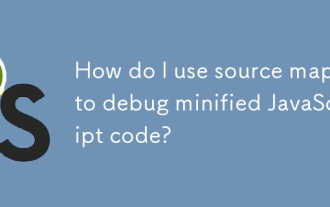
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
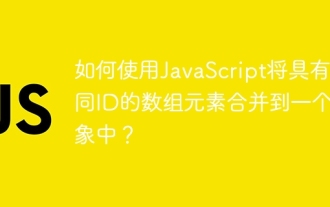
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
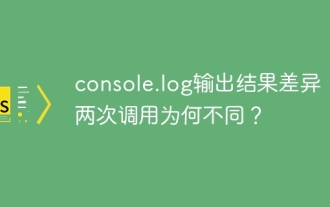
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
