How to use the Intersection Observer in React
Today we will explore how to use the intersection observer API in React with some examples.
Mozilla web documentation describes the intersection observer API as:
allows code to register a callback function that runs whenever an element they want to monitor enters or leaves another element (or the viewport), or when the value by which the two intersect changes of a requested amount. This way, sites no longer need to do anything on the main thread to observe this type of intersection of elements, and the browser is free to optimize intersection management as it sees fit.
In short, it allows us to detect when a certain element is visible in the viewport, this only happens when the element meets the desired intersection ratio.
As you can see, if you scroll down the page the intersection ratio will increase until it reaches the projected limit and at that moment the function that executes a callback is triggered.
First step
const observer = new IntersectionObserver(callbackFunction, options) observer.observer(elementToObserver)
The intersection observer constructor object needs two arguments:
- A callback function
- Options
That's all, we're ready to see some action, but first, we need to know what each option means, the options argument is an object with the following values:
const options = { root: null, rootMargin: "0px", threshold: 1 }
- root: The element that is used as a viewport to check the visibility of the target. Must be the target's ancestor. Defaults to browser viewport if not specified or null.
- rootMargin: This set of values is used to increase or decrease each side of the root element's bounding box before calculating the intersections, the options are similar to those for margin in CSS.
- threshold: A single number or an array of numbers that indicates at what percentage of the target's visibility the observer callback should run, ranges from 0 to 1.0, where 1.0 means each pixel is visible in the viewport.
Using in React
Now we will see an implementation of the intersection observer API in React.
const observer = new IntersectionObserver(callbackFunction, options) observer.observer(elementToObserver)
- Start with a reference to the element we want to observe, use the react hook useRef.
- Create a state variable isVisible, we will use it to display a message whenever our box is in the viewport.
- Declare the callback function that will receive an array of IntersectionObserverEntries as a parameter, within this function we take the first and only entry and check if it is intersecting with the viewport and if it is then we call setIsVisible with the value of entry.isIntersecting (true / FALSE).
- Create the options object with the same values as the image.
- Add the useEffect react hook and create an observer constructor using the callback function and options we just created before. It's optional in our case, but it can return a cleanup function to unwatch our target when the component is unmounted.
- Set the useRef variable on the element we want to observe.
const options = { root: null, rootMargin: "0px", threshold: 1 }
- Let's add some style to this html.
- That's all we need, simple and easy!
Remember, this is just a basic implementation and there are several ways to do this.
Now we will implement the same code we did previously, but separating all the logic in a nu hook called useElementOnScreen.
const containerRef = useRef(null) const [isVisible, setIsVisible] = useState(false) const callbackFunction = (entries) => { const [entry] = entries setIsVisible(entry.isIntersecting) } const options = { root: null, rootMargin: "0px", threshold: 1.0 } useEffect(() => { const observer = new IntersectionObserver(callbackFunction, options) if (containerRef.current) observer.observe(containerRef.current) return () => { if (containerRef.current) observer.unobserve(containerRef.current) } }, [containerRef, options]) return ( <div className="app"> <div className="isVisible">{isVisible ? "IN VIEWPORT" : "NOT IN VIEWPORT"}</div> <div className="section"></div> <div className="box" ref={containerRef}>Observe me</div> </div> )
- Create a new function called useElementOnScreen with parameter options
- Move the entire useRef, useState and useEffect inside our new hook.
- Now the only thing missing from our hook is the return statement, we pass isVisible and containerRef as an array.
- ok, we're almost there, we just need to call it in our component and see if it works!
<div className="box" ref={containerRef}>Observe me</div>
1- Import the newly created hook into our component.
2 - Initialize it with the options object.
3 - This is how we finish.
Congratulations, we have successfully used the intersection observer API and even created a hook for it!
Credits
Intersection Observer using React, originally written by producthackers
Thank you for reading this article. I hope I can provide you with some useful information. If so, I would be very happy if you would recommend this post and click the ♥ button so more people can see this.
The above is the detailed content of How to use the Intersection Observer in React. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


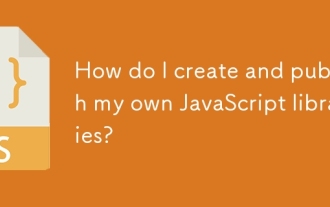
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
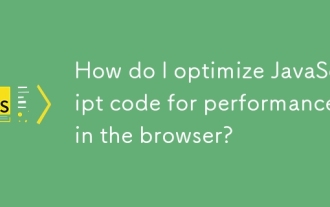
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
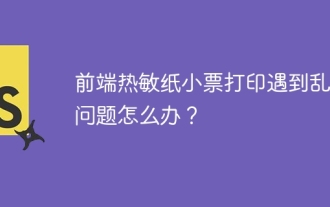
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
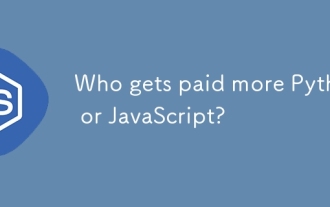
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
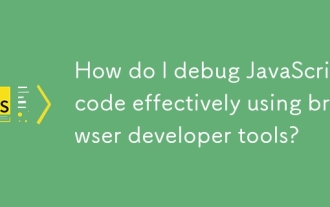
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
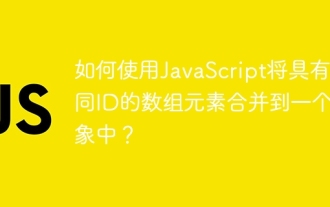
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
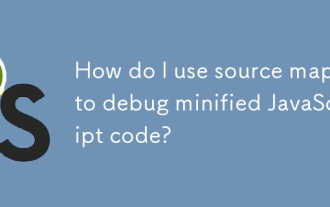
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
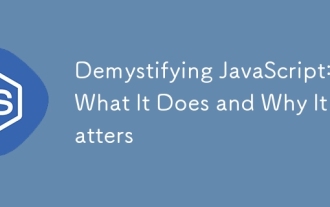
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
