How to Implement If/Else Statements in ANTLR 4 using Visitors?
If/else Statements with ANTLR 4
ANTLR 4 uses listeners by default, but it also supports visitors. Visitors provide more control over the traversal of the parse tree, making them more suitable for implementing if/else statements. To enable visitors, run the following command:
java -cp antlr-4.0-complete.jar org.antlr.v4.Tool Mu.g4 -visitor
This will generate a class called MuBaseVisitor
<code class="java">public class EvalVisitor extends MuBaseVisitor<Value> { // Override visit methods for each rule that needs to be implemented // Example: visitIf_stat for handling if/else statements @Override public Value visitIf_stat(MuParser.If_statContext ctx) { List<MuParser.Condition_blockContext> conditions = ctx.condition_block(); boolean evaluatedBlock = false; for (MuParser.Condition_blockContext condition : conditions) { Value evaluated = this.visit(condition.expr()); if (evaluated.asBoolean()) { evaluatedBlock = true; this.visit(condition.stat_block()); // Evaluate the true block break; } } if (!evaluatedBlock && ctx.stat_block() != null) { this.visit(ctx.stat_block()); // Evaluate the else block } return Value.VOID; } }</code>
Here, we iterate through the conditions and evaluate the first true one. If no condition is true and an else block is present, we evaluate that instead.
To use this visitor, create a Main class to parse and evaluate the input:
<code class="java">public class Main { public static void main(String[] args) throws Exception { MuLexer lexer = new MuLexer(new ANTLRFileStream("test.mu")); MuParser parser = new MuParser(new CommonTokenStream(lexer)); ParseTree tree = parser.parse(); EvalVisitor visitor = new EvalVisitor(); visitor.visit(tree); // Start the evaluation process } }</code>
The above is the detailed content of How to Implement If/Else Statements in ANTLR 4 using Visitors?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


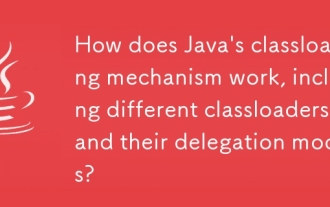
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
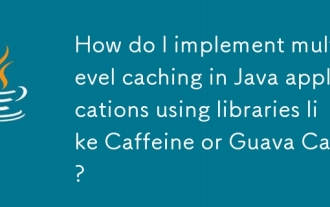
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
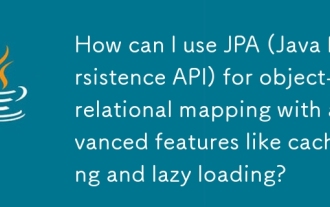
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
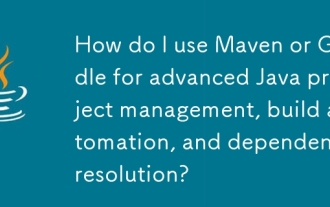
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
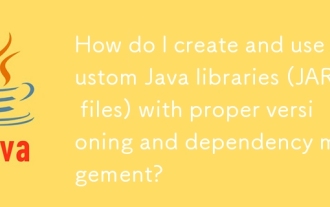
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
