## How to Effectively Implement if/Else Statements in ANTLR Using Visitors?
If/Else Statements in ANTLR using Listeners
Implementing if/else statements in ANTLR using the listener pattern can be challenging due to the fact that ANTLR decides the order in which to traverse the parse tree. To fully understand how to implement if/else patterns using listeners and visitors, consider the following:
Default Listener Generation
By default, ANTRL 4 generates listeners. However, if you provide the command line parameter -visitor to org.antlr.v4.Tool, ANTL generates visitor classes. These classes offer more control over which (sub) trees are walked/visited.
Implementing if/Else with Listener Pattern
Using listeners, you would need to introduce global variables to keep track of sub-trees that need to be evaluated and which do not. This can lead to a less clean implementation.
Advantages of Using the Visitor Pattern
Implementing if/else statements using visitors provides a cleaner approach. Visitors allow you to define your own traversal order and logic, making it easier to handle conditional logic.
To begin, use the command:
java -cp antlr-4.0-complete.jar org.antlr.v4.Tool Mu.g4 -visitor
This will generate the MuBaseVisitor class, which is the starting point for your visitor implementation.
Here's an example of handling the if/else statement with a visitor:
<code class="java">public class EvalVisitor extends MuBaseVisitor<Value> { // ... visitors for other rules // if_stat override @Override public Value visitIf_stat(MuParser.If_statContext ctx) { List<MuParser.Condition_blockContext> conditions = ctx.condition_block(); boolean evaluatedBlock = false; for(MuParser.Condition_blockContext condition : conditions) { Value evaluated = this.visit(condition.expr()); if(evaluated.asBoolean()) { evaluatedBlock = true; // evaluate this block whose expr==true this.visit(condition.stat_block()); break; } } if(!evaluatedBlock && ctx.stat_block() != null) { // evaluate the else-stat_block (if present == not null) this.visit(ctx.stat_block()); } return Value.VOID; } }</code>
Test the Implementation
To test this implementation, use the following Main class:
<code class="java">public class Main { public static void main(String[] args) throws Exception { MuLexer lexer = new MuLexer(new ANTLRFileStream("test.mu")); MuParser parser = new MuParser(new CommonTokenStream(lexer)); ParseTree tree = parser.parse(); EvalVisitor visitor = new EvalVisitor(); visitor.visit(tree); } }</code>
Compile and run the source files:
javac -cp antlr-4.0-complete.jar *.java java -cp .:antlr-4.0-complete.jar Main
After running Main, your console will output the results of evaluating the input file test.mu.
Conclusion
Implementing if/else statements in ANTLR using visitors provides a clearer and more structured approach compared to using listeners. Visitors offer more control over traversing the parse tree and allow you to implement conditional logic more efficiently.
The above is the detailed content of ## How to Effectively Implement if/Else Statements in ANTLR Using Visitors?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
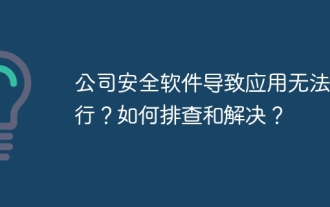
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
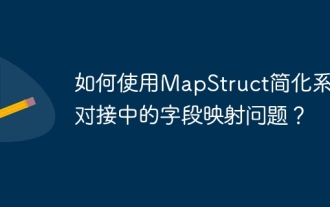
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
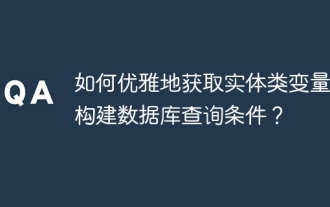
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
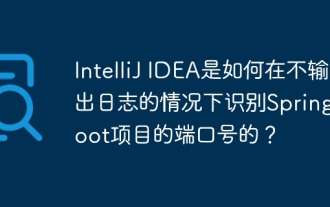
Start Spring using IntelliJIDEAUltimate version...
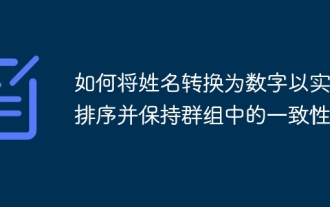
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
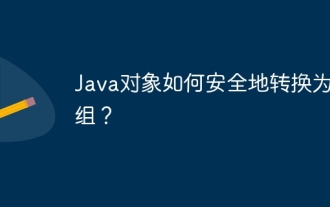
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
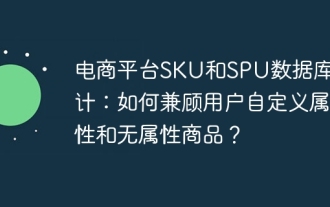
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
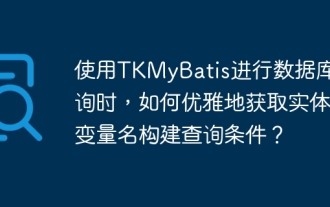
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
