


How can I store and retrieve multiple values associated with a single key in a Java HashMap?
Oct 25, 2024 am 09:46 AMRetrieving Multiple Values for a Key in a HashMap
HashMap is a commonly used Java collection that maps keys to a single value. However, in certain scenarios, you may find yourself desiring the capability to store multiple values for a given key. This raises the question: Is it possible to access multiple values associated with a particular key in a HashMap?
While HashMap natively supports only one value per key, there are techniques to achieve this functionality. One straightforward approach is to utilize a Map of List structure.
Creating a Map of List
To store multiple values for each key, we can create a HashMap that maps objects to ArrayLists of objects, as demonstrated in the code snippet below:
<code class="java">Map<Object, ArrayList<Object>> multiMap = new HashMap<>();</code>
This approach allows us to add multiple values to a single key by appending them to the corresponding ArrayList. To retrieve the third value for a given key, we can simply access the ArrayList and retrieve the third element.
Example Usage
Assuming we have a HashMap with multiple values for certain keys, we can access the third value for a key as follows:
<code class="java">List<Object> values = multiMap.get(key); Object thirdValue = values.get(2);</code>
Here, values is the list of values associated with the key, and thirdValue represents the third value in the list.
By utilizing a Map of List, you can effectively store and access multiple values for each key in a HashMap. This technique provides greater flexibility and allows you to handle more complex data structures effortlessly.
The above is the detailed content of How can I store and retrieve multiple values associated with a single key in a Java HashMap?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
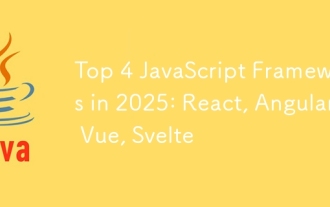
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
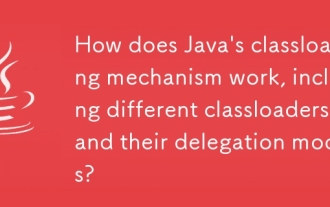
How does Java's classloading mechanism work, including different classloaders and their delegation models?
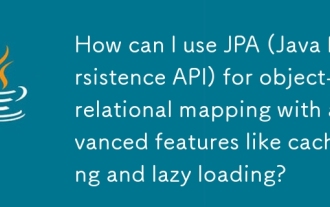
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
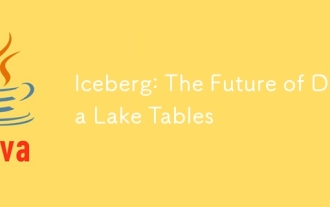
Iceberg: The Future of Data Lake Tables
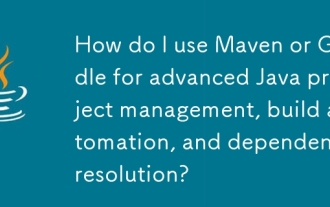
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
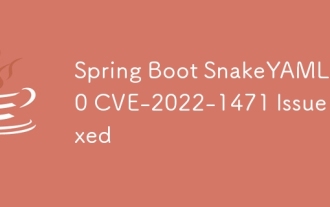
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
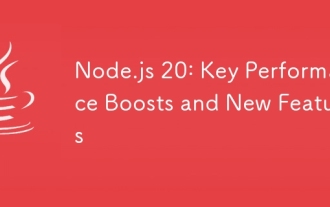
Node.js 20: Key Performance Boosts and New Features
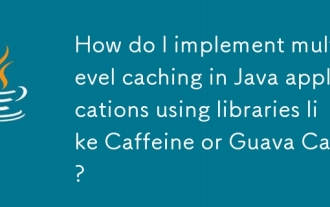
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
