


How can SSE instructions and assembly optimization improve the performance of a population count algorithm with a two-level loop?
Understanding the Issue
In your code, you handle population counts within a two-level loop and try to optimize the inner loop with assembly. The loop iterates through a byte slice and uses the __mm_add_epi32_inplace_purego function to add positional popcounts to an array.
Optimization via Assembly
To optimize the inner loop, you can implement __mm_add_epi32_inplace_purego in assembly. Below is the suggested optimized version of the function:
<code class="assembly">.text .globl __mm_add_epi32_inplace_purego __mm_add_epi32_inplace_purego: movq rdi, [rsi] movq rsi, [rdi+8] addq rsi, rdi movups (%rsi, %rax, 8), %xmm0 addq , %rsi movups (%rsi, %rax, 8), %xmm1 paddusbd %xmm0, %xmm0 paddusbd %xmm1, %xmm1 vextracti128 <pre class="brush:php;toolbar:false"><code class="assembly">.text .globl __optimized_population_count_loop __optimized_population_count_loop: movq rdi, [rsi] leaq (0, %rdi, 4), %rdx # multiple rdi by 4, rdx = counts movq rsp, r11 and rsp, -16 subq r15, r11 movq r15, r9 mov rdi, (%rsi) movq r15, rsi mov %rsi, rsi pxor %eax, %eax dec %rsi .loop: inc %rsi addq , rsi cmp rsi, rdi cmovge %rsi, rsi movsw (%rdi, %rax, 2), %ax movsw (%rsi, %rax, 2), %dx movw %ax, (%rdx) movw %dx, 2(%rdx) .end_loop:</code>
Explanation:
This assembly code optimizes the function using packed SSE instructions. It:
- Computes popcounts in 16-bit blocks using paddusbd.
- Extracts the low 128-bit portion using vextracti128.
- Adds the results to the [8]int32 array at the address given by %rdi.
Enhanced Whole Loop with Assembly
Explanation:
The complete loop is now optimized in assembly. It uses:
- A loop to process 2-byte chunks.
- Streaming loads via consecutive addq $32, rsi to avoid cache misses.
- A fast and compact way to save the results using movw.
Conclusion
This optimized version should significantly improve the performance of your algorithm for computing positional population counts.
The above is the detailed content of How can SSE instructions and assembly optimization improve the performance of a population count algorithm with a two-level loop?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










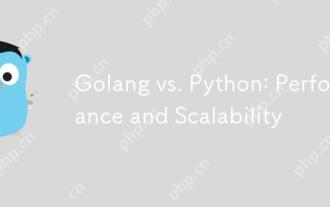
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
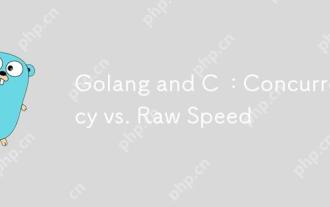
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
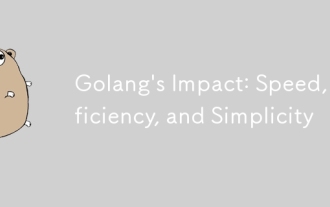
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
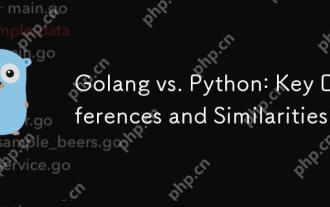
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
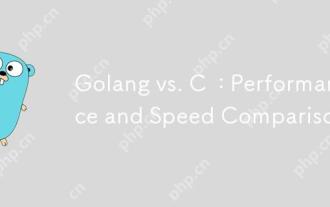
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
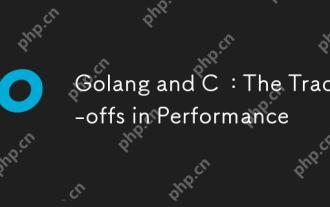
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
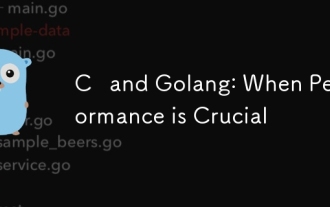
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
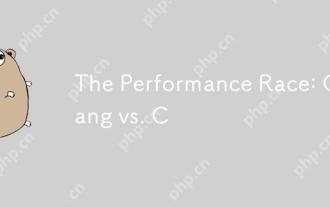
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
