


How can I efficiently manage a list of Futures and handle exceptions in Java?
Efficiently Managing a List of Futures with Exception Handling
In Java, when working with futures, one may encounter the need to wait for a list of future tasks to complete while handling any exceptions that arise. A simple approach to this would be to sequentially wait for each future and check for potential exceptions. However, this approach suffers from efficiency issues if an exception occurs earlier in the list, as subsequent tasks would still be waited on unnecessarily.
To address this, an alternative solution leverages the CompletionService class. The CompletionService receives futures as they become available and allows for early termination if an exception is encountered.
An example of how to implement this approach is provided below:
<code class="java">Executor executor = Executors.newFixedThreadPool(4); CompletionService<SomeResult> completionService = new ExecutorCompletionService<>(executor); // 4 tasks for (int i = 0; i < 4; i++) { completionService.submit(new Callable<SomeResult>() { public SomeResult call() { // ... task implementation return result; } }); } int received = 0; boolean errors = false; while (received < 4 && !errors) { Future<SomeResult> resultFuture = completionService.take(); // Blocks if none available try { SomeResult result = resultFuture.get(); received++; // ... do something with the result } catch (Exception e) { // Log the exception errors = true; } } // Potentially consider canceling any still running tasks if errors occurred</code>
By utilizing the CompletionService, you can efficiently wait for future tasks to complete while handling exceptions promptly.
The above is the detailed content of How can I efficiently manage a list of Futures and handle exceptions in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










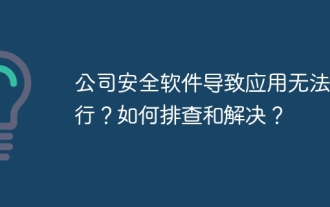
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
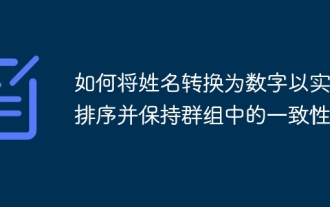
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
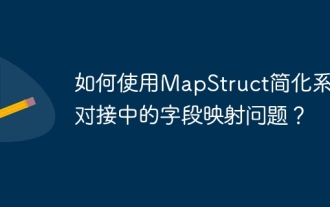
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
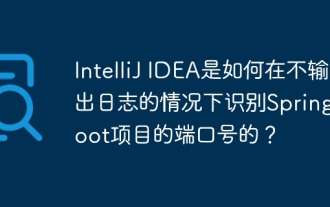
Start Spring using IntelliJIDEAUltimate version...
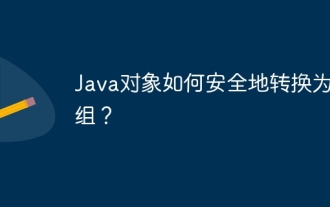
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
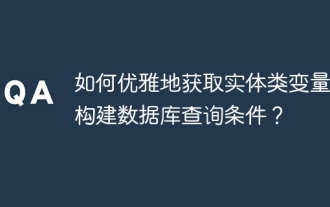
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
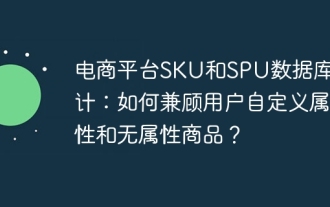
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
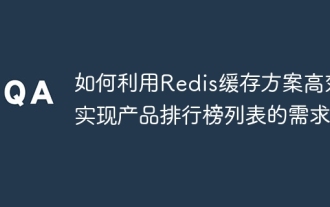
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
