How to Invoke ES6 Classes Without the `new` Keyword?
ES6: Bypassing the new Keyword for Class Constructor Invocation
In ES6, classes serve not only as blueprints for objects but also embody their constructors. The constructor() function serves as the class body, and its execution is triggered when the class is invoked. However, attempting to call a class without the required new keyword often results in the "Cannot call a class as a function" error message.
Understanding the Constructor Imperative
Classes are inherently tied to the new operator, which ensures that a new instance of the class is created upon instantiation. Invoking a class without new goes against this design principle, leading to the aforementioned error.
Alternative Solutions
If the desired behavior is to allow class invocation with or without new, several alternatives exist:
1. Using a Regular Function Instead of a Class
A regular function can mimic class-like behavior without the new requirement. It can still enforce instance-like behavior using this, but it lacks the encapsulation and inheritance features of classes.
<code class="js">function Foo(x) { this.x = x; this.hello = function() { return this.x; }; }</code>
2. Enforcing the new Keyword
If the intended purpose is to always invoke the class with new, there is no need for any workarounds. Simply adhere to the recommended constructor invocation pattern:
<code class="js">(new Foo("world")).hello(); // "hello world"</code>
3. Wrapping the Class with a Regular Function
This approach provides the flexibility to invoke the class with or without new while maintaining the benefits of using classes. The wrapping function always invokes the class constructor with new.
<code class="js">class Foo { constructor(x) { this.x = x; } hello() { return `hello ${this.x}`; } } var _old = Foo; Foo = function(...args) { return new _old(...args) }; Foo("world").hello(); // "hello world" (new Foo("world")).hello(); // Also works</code>
The above is the detailed content of How to Invoke ES6 Classes Without the `new` Keyword?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










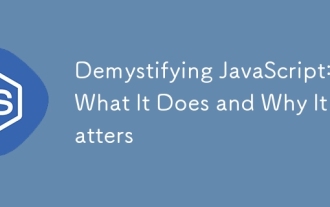
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
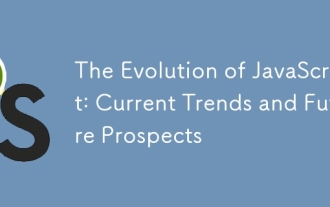
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
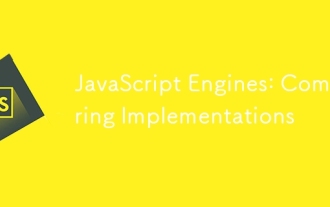
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
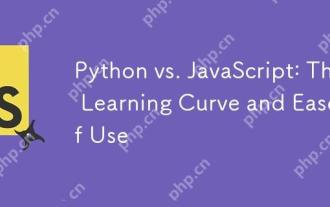
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
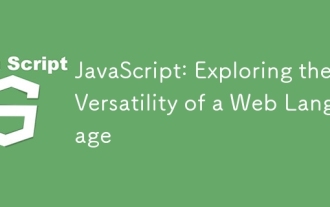
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
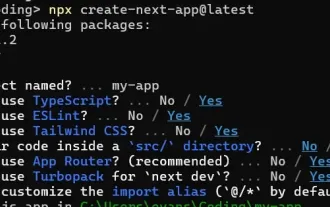
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
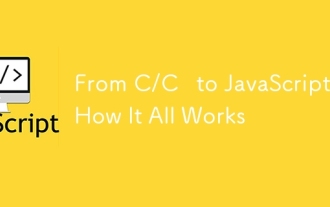
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
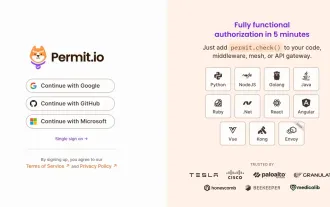
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
