In today's fast-paced development world, delivering solutions quickly is essential. However, cutting corners on code quality often leads to bugs, security vulnerabilities, and unmaintainable code. Code ethics play a pivotal role in producing not only functional but also maintainable, efficient, and secure code. Let’s explore key ethical principles in JavaScript development and how they can improve your code quality with examples.
Example: Avoid using terse or complex constructs when clearer alternatives exist.
Bad Example
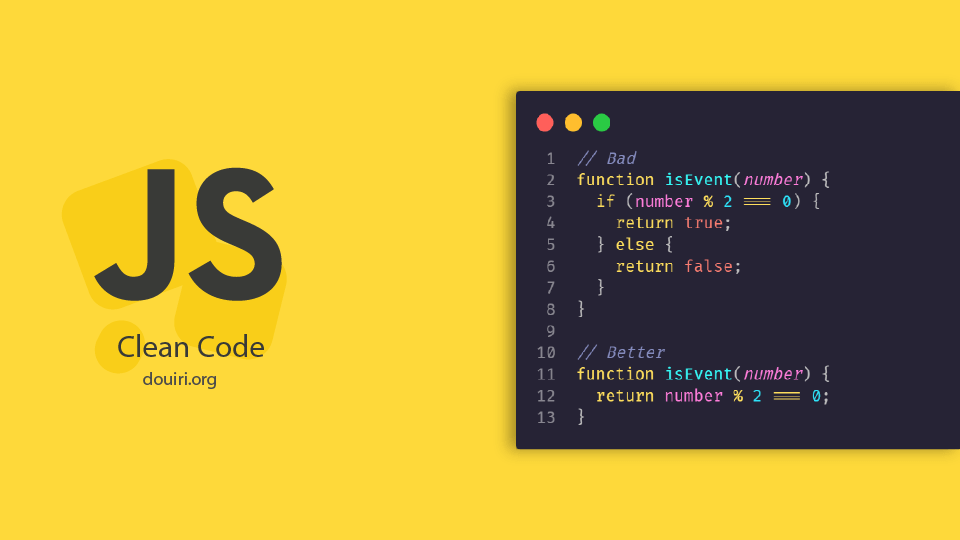
Good Example
const doubleArray = arr => arr.map(x => x * 2); // Clear and easily understood
In this example, the bitwise operator << works but is less readable than using simple multiplication. Choosing clarity ensures your team or future self can easily understand and maintain the code.
Bad Example
let count = 0; // Declared in global scope
function increment() {
count ;
}
Good Example
(() => {
let count = 0; // Encapsulated in a closure
function increment() {
count ;
}
})();
By wrapping the code in an IIFE (Immediately Invoked Function Expression), the count variable is scoped locally, avoiding potential conflicts with other parts of the code.
Bad Example
function getUser(id) {
return fetch(/user/${id}).then(res => res.json()); // No error handling
}
Good Example
async function getUser(id) {
try {
const res = await fetch(/user/${id});
if (!res.ok) {
throw new Error(Failed to fetch user: ${res.statusText});
}
return await res.json();
} catch (error) {
console.error('Error fetching user:', error);
return null;
}
}
By adding error handling, you not only prevent your app from failing silently but also provide meaningful information about what went wrong.
Bad Example
function processOrder(order) {
// Code for validating order
// Code for calculating total
// Code for processing payment
// Code for generating receipt
}
Good Example
`function validateOrder(order) { /* ... / }
function calculateTotal(order) { / ... / }
function processPayment(paymentInfo) { / ... / }
function generateReceipt(order) { / ... */ }
function processOrder(order) {
if (!validateOrder(order)) return;
const total = calculateTotal(order);
processPayment(order.paymentInfo);
generateReceipt(order);
}`
This modular approach makes your code easier to understand, test, and maintain. Each function has a single responsibility, adhering to the Single Responsibility Principle (SRP).
Bad Example
function processUser(user) {
console.log(Processing user: ${JSON.stringify(user)}); // Exposing sensitive data
// ...
}
Good Example
function processUser(user) {
console.log(Processing user: ${user.id}); // Logging only the necessary details
// ...
}
In this case, the bad example exposes potentially sensitive user information in the console. The good example logs only what’s necessary, following data privacy best practices.
Bad Example
`function createAdmin(name, role) {
return { name, role, permissions: ['create', 'read', 'update', 'delete'] };
}
function createEditor(name, role) {
return { name, role, permissions: ['create', 'read'] };
}`
Good Example
`function createUser(name, role, permissions) {
return { name, role, permissions };
}
const admin = createUser('Alice', 'Admin', ['create', 'read', 'update', 'delete']);
const editor = createUser('Bob', 'Editor', ['create', 'read']);`
By following the DRY principle, you eliminate code duplication, reducing the chance for inconsistencies or errors in future updates.
Bad Example
function calculateAPR(amount, rate) {
return amount * rate / 100 / 12; // No explanation of what the formula represents
}
Good Example
`/**
Bad Example
// No test coverage
Good Example
// Using a testing framework like Jest or Mocha
test('calculateAPR should return correct APR', () => {
expect(calculateAPR(1000, 12)).toBe(10);
});
By writing tests, you ensure your code is reliable, verifiable, and easy to refactor with confidence.
Consider using tools like ESLint or Prettier to enforce consistency in your code.
Example ESLint Configuration
{
"extends": "eslint:recommended",
"env": {
"browser": true,
"es6": true
},
"rules": {
"indent": ["error", 2],
"quotes": ["error", "single"],
"semi": ["error", "always"]
}
}
By adhering to a style guide, your codebase will maintain a consistent structure, making it easier for others to contribute and review code.
Conclusion
Ethical JavaScript coding practices ensure that your code is not only functional but also maintainable, secure, and future-proof. By focusing on clarity, modularity, error handling, and data privacy, you create a codebase that respects both your fellow developers and end users. Incorporating these practices into your workflow will help you write cleaner, more reliable code and foster a healthier development environment.
The above is the detailed content of JavaScript Code Ethics: Writing Clean, Ethical Code. For more information, please follow other related articles on the PHP Chinese website!