How Do I Modify Strings in Go, Knowing They Are Immutable?
Altering Strings in Go: A Practical Solution
In Go, strings are immutable, meaning their contents cannot be modified once created. This can be frustrating when attempting to alter an existing string, but there is a simple solution using the fmt package.
Consider the following code:
<code class="go">package main import "fmt" func ToUpper(str string) string { new_str := str for i := 0; i < len(str); i++ { if str[i] >= 'a' && str[i] <= 'z' { chr := uint8(rune(str[i]) - 'a' + 'A') new_str[i] = chr } } return new_str } func main() { fmt.Println(ToUpper("cdsrgGDH7865fxgh")) }</code>
This code attempts to uppercase lowercase characters in a string, but you will encounter an error: "cannot assign to new_str[i]". This is because strings are immutable.
To overcome this, we can convert the string to a slice of bytes and alter that instead:
<code class="go">func ToUpper(str string) string { new_str := []byte(str) for i := 0; i < len(str); i++ { if str[i] >= 'a' && str[i] <= 'z' { chr := uint8(rune(str[i]) - 'a' + 'A') new_str[i] = chr } } return string(new_str) }</code>
Here, []byte(str) creates a byte slice from the string, and string(new_str) converts the modified byte slice back to a string.
With this change, you can now alter strings and covert lowercase characters to uppercase:
fmt.Println(ToUpper("cdsrgGDH7865fxgh")) // Output: CDSRGgdh7865FXGH
The above is the detailed content of How Do I Modify Strings in Go, Knowing They Are Immutable?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


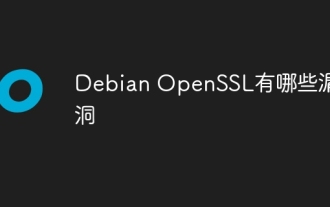
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
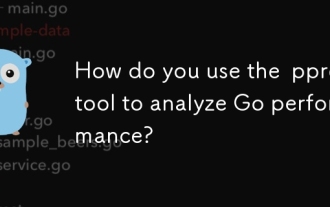
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
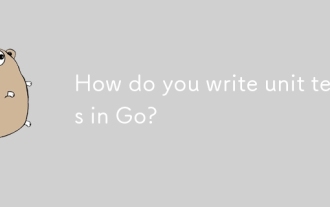
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
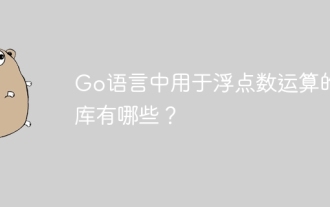
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
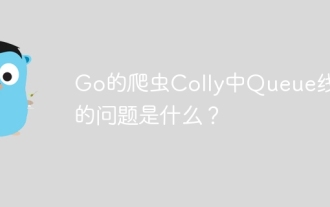
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
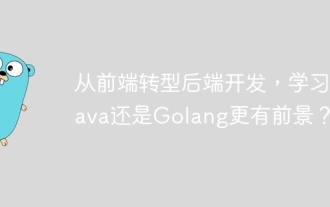
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
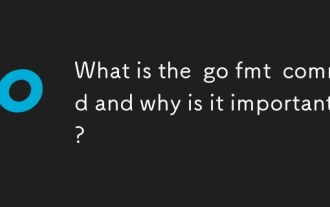
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
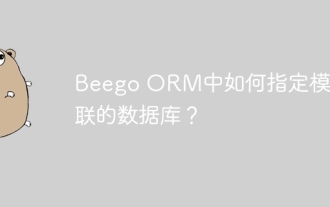
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
