## Why Does Deferring GZIP Writer Closure Lead to Data Loss in Go?
Deferring GZIP Writer Closure Can Lead to Data Loss
The GZIP writer is a commonly used utility in Go for data compression. This writer has a Close method that not only flushes data but also writes the GZIP footer, which is required for successful decompression.
In certain scenarios, it's tempting to defer the Close call to ensure it's executed at the end of a function. However, this approach can result in data loss.
To understand why, consider the following code:
<code class="go">func main() { data := []byte("Hello World") compressedData, err := zipData(data) if err != nil { panic(err) } uncompressedData, err := unzipData(compressedData) if err != nil { panic(err) } fmt.Println(string(uncompressedData)) }</code>
The zipData function uses a GZIP writer, and the unzipData function reads the compressed data:
<code class="go">func zipData(data []byte) ([]byte, error) { buf := new(bytes.Buffer) gw := gzip.NewWriter(buf) // Deferring Close here causes data loss! defer gw.Close() _, err := gw.Write(data) if err != nil { return nil, err } err = gw.Flush() if err != nil { return nil, err } return buf.Bytes(), nil }</code>
<code class="go">func unzipData(data []byte) ([]byte, error) { r := bytes.NewReader(data) gr, err := gzip.NewReader(r) if err != nil { return nil, err } defer gr.Close() uncompressed, err := ioutil.ReadAll(gr) if err != nil { return nil, err } return uncompressed, nil }</code>
The issue arises when deferring the Close call in zipData. This means the function returns before the GZIP footer is written, resulting in the omission of essential data from the compressed byte array. This error manifests as an unexpected EOF when attempting to read the decompressed data.
To resolve this issue, the Close method of the GZIP writer should be called before returning the compressed data. This ensures that the footer is written, and the data is complete.
<code class="go">func zipData(data []byte) ([]byte, error) { buf := new(bytes.Buffer) gw := gzip.NewWriter(buf) // Close the writer before returning defer gw.Close() _, err := gw.Write(data) if err != nil { return nil, err } err = gw.Flush() if err != nil { return nil, err } return buf.Bytes(), nil }</code>
By closing the writer before returning, we guarantee that the complete compressed data, including the GZIP footer, is available for decompression without any data loss.
The above is the detailed content of ## Why Does Deferring GZIP Writer Closure Lead to Data Loss in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
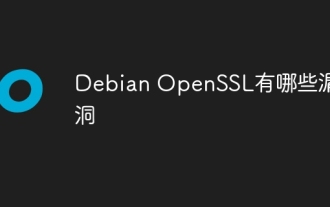
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
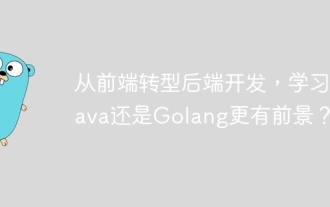
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
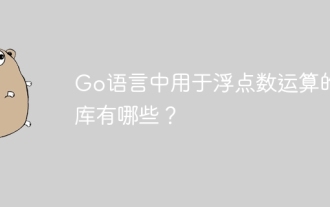
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
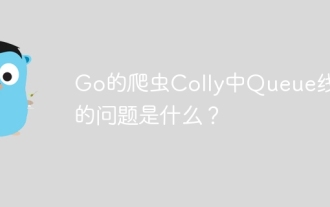
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
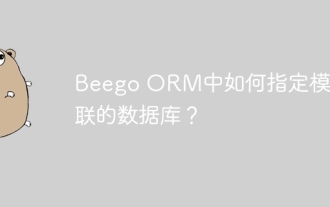
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
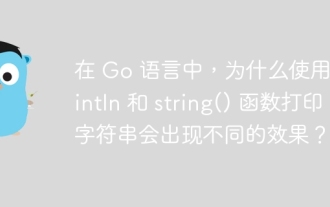
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
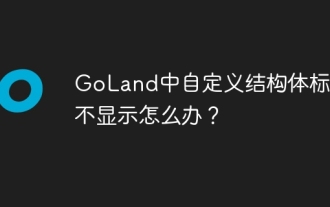
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
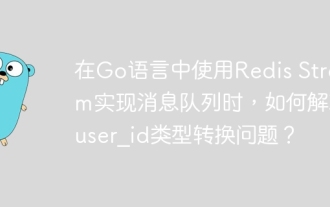
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
