


How does \'this\' behave differently in jQuery and regular JavaScript, and what are the implications for working with DOM elements and function calls?
Understanding "this" in jQuery and JavaScript
In jQuery, "this" typically refers to the DOM element that is associated with the function being called. For instance, in an event callback, "this" represents the element that triggered the event.
Example:
<code class="javascript">$("div").click(function() { // Here, `this` will be the DOM element for the div that was clicked, // so you could (for instance) set its foreground color: this.style.color = "red"; });</code>
jQuery also uses "this" in functions like html() and each():
Example (html):
<code class="javascript">$("#foo div").html(function() { // Here, `this` will be the DOM element for each div element return this.className; });</code>
Example (each):
<code class="javascript">jQuery.each(["one", "two", "three"], function() { // Here, `this` will be the current element in the array alert(this); });</code>
"this" in Generic JavaScript
Outside of jQuery, "this" in JavaScript generally refers to an object. However, this is not strictly true in ES5's strict mode, where "this" can have any value.
The value of "this" in a function call is determined by how the function is invoked. It can be explicitly set by calling the function through an object property, or it can default to the global object (window in browsers).
Example:
<code class="javascript">var obj = { firstName: "Fred", foo: function() { alert(this.firstName); } }; obj.foo(); // alerts "Fred"</code>
In this example, "this" is explicitly set to the obj object, so it can access the firstName property.
However, it's important to note that the function foo is not inherently tied to any specific object. It can be called with a different "this" value using functions like .call and .apply:
<code class="javascript">function foo(arg1, arg2) { alert(this.firstName); alert(arg1); alert(arg2); } var obj = {firstName: "Wilma"}; foo.call(obj, 42, 27); // alerts "Wilma", "42", and "27"</code>
In this example, foo is called with the obj object as "this," allowing it to access the firstName property.
ES5's strict mode introduces further complexity, allowing "this" to have non-object values like null, undefined, or primitives like strings and numbers:
<code class="javascript">(function() { "use strict"; // Strict mode test("direct"); test.call(5, "with 5"); test.call(true, "with true"); test.call("hi", "with 'hi'"); function test(msg) { console.log("[Strict] " + msg + "; typeof this = " + typeof this); } })();</code>
Output:
[Strict] direct; typeof this = undefined [Strict] with 5; typeof this = number [Strict] with true; typeof this = boolean [Strict] with 'hi'; typeof this = string
In strict mode, "this" is determined by the call site rather than the definition of the function, and it can have non-object values.
The above is the detailed content of How does \'this\' behave differently in jQuery and regular JavaScript, and what are the implications for working with DOM elements and function calls?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










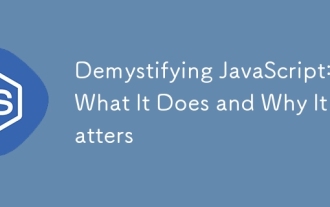
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
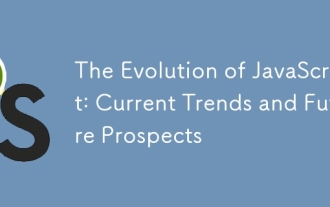
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
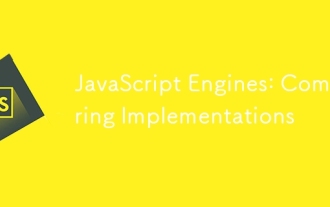
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
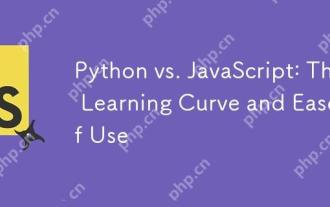
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
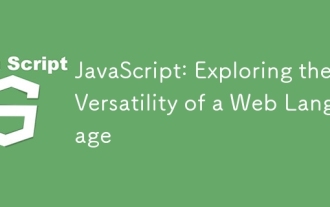
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
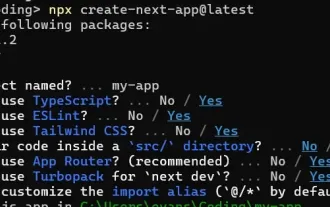
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
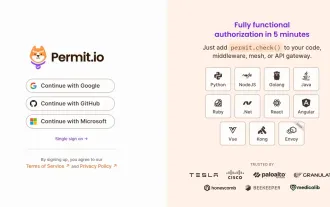
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
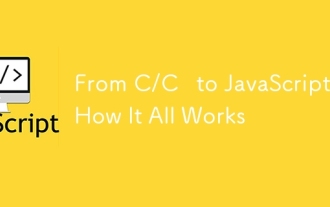
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
