


How to Index a 2D NumPy Array with Two Lists of Indices Using `np.ix_`?
Indexing a 2D Numpy array with 2 lists of indices
Problem Statement
Indexing a 2D Numpy array with two separate lists of indices is not as straightforward as using a single list of indices. This can be challenging when dealing with large arrays, as it requires broadcasting and reshaping of arrays to achieve the desired indexed selection.
Solution Using np.ix_ and Broadcasting
The np.ix_ function in Numpy can be used to create a tuple of indexing arrays that can be broadcast against each other to achieve the desired indexing pattern. This approach maintains readability and promotes code optimization.
To perform indexing using np.ix_, follow these steps:
- Create two broadcasting arrays using np.ix_ with the row and column indices.
- Use these indexing arrays to select the desired rows and columns in the original array.
Example Code
The following code demonstrates how to use np.ix_ for index-based selections:
<code class="python">import numpy as np # Create indices row_indices = [4, 2, 18, 16, 7, 19, 4] col_indices = [1, 2] # Create broadcasting arrays index_tuples = np.ix_(row_indices, col_indices) # Perform indexing x_indexed = x[index_tuples]</code>
Example Output
>>> x_indexed array([[76, 56], [70, 47], [46, 95], [76, 56], [92, 46]])
Additional Considerations
Alternative Syntax:
An alternative syntax for using np.ix_ is to use the : operator to specify all indices along an axis unless otherwise specified.
Broadcasting:
It's important to note that broadcasting occurs along the axes of the input array. Therefore, the size of the indexing arrays along each axis should match the corresponding dimensions of the input array.
Optimization:
Indexing using np.ix_ and broadcasting can provide significant performance benefits compared to iterating over indices or using boolean masks. This is especially advantageous when working with large arrays.
The above is the detailed content of How to Index a 2D NumPy Array with Two Lists of Indices Using `np.ix_`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










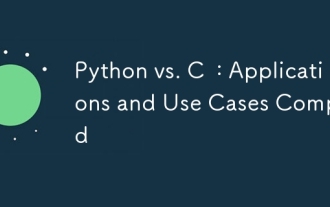
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
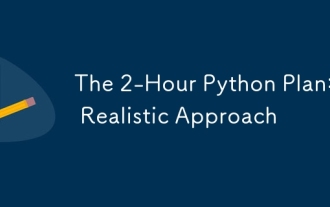
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
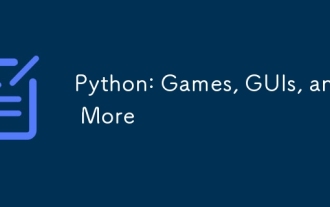
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
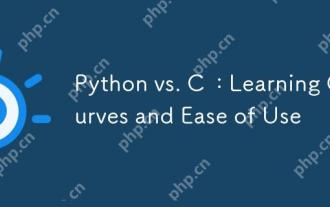
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
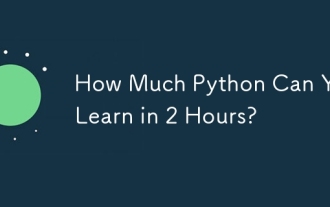
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
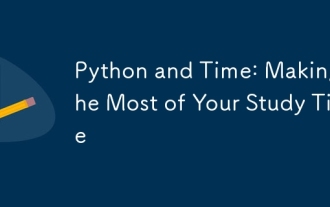
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
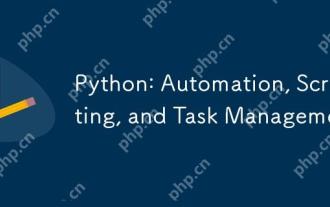
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
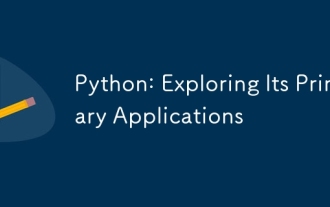
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
