


How to Remove Elements from a Python Dictionary: Del or Shallow Copy?
Delete an Element from a Dictionary
When working with dictionaries in Python, there may come a time when you need to remove an element. This can be achieved in two ways:
1. Modifying the Original Dictionary
Using the del statement, you can directly remove an element from the dictionary, thereby modifying the original dictionary:
<code class="python">del d[key]</code>
where d is the dictionary and key is the key of the element to be removed.
2. Obtain a New Dictionary without the Element
To obtain a new dictionary with the element removed, without modifying the original dictionary, you can use the following method:
<code class="python">def removekey(d, key): r = dict(d) del r[key] return r</code>
This function first creates a copy of the original dictionary d using the dict() constructor. Then, it removes the specified key from the copy and returns the modified copy r as a new dictionary.
Note:
- The dict() constructor performs a shallow copy, meaning that changes made to the new dictionary will not affect the original dictionary and vice versa.
- For large dictionaries, creating copies for every deletion operation can be inefficient. Consider using a different data structure, such as a HAMT (hashed array-mapped trie), for efficient element removal.
The above is the detailed content of How to Remove Elements from a Python Dictionary: Del or Shallow Copy?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


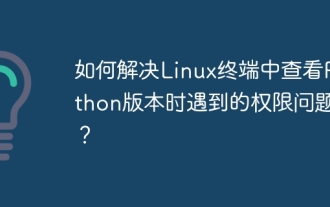
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
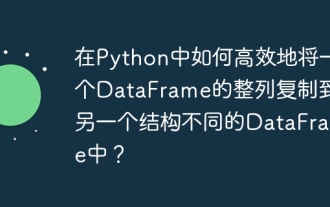
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
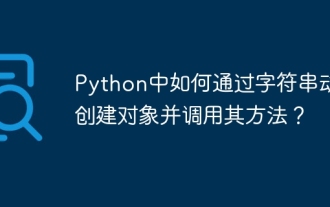
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
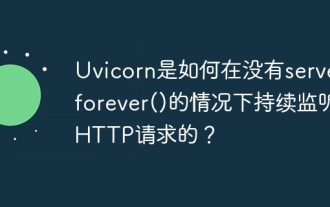
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
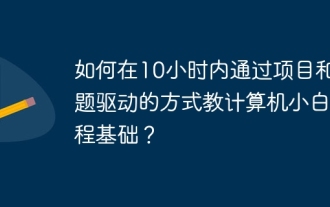
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
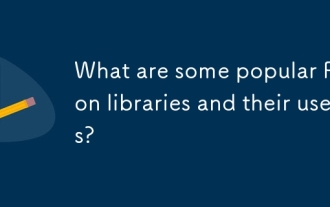
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
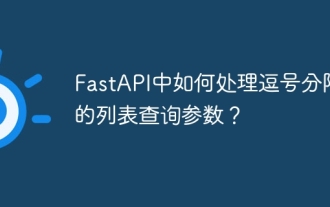
Fastapi ...
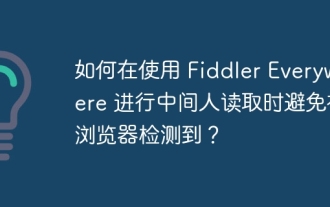
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
